How to Add Days to Current Date in JavaScript
-
Understand JavaScript
Date
Class -
Add Days to
Date()
in JavaScript -
Add Days to
Date()
Usingprototype
in JavaScript
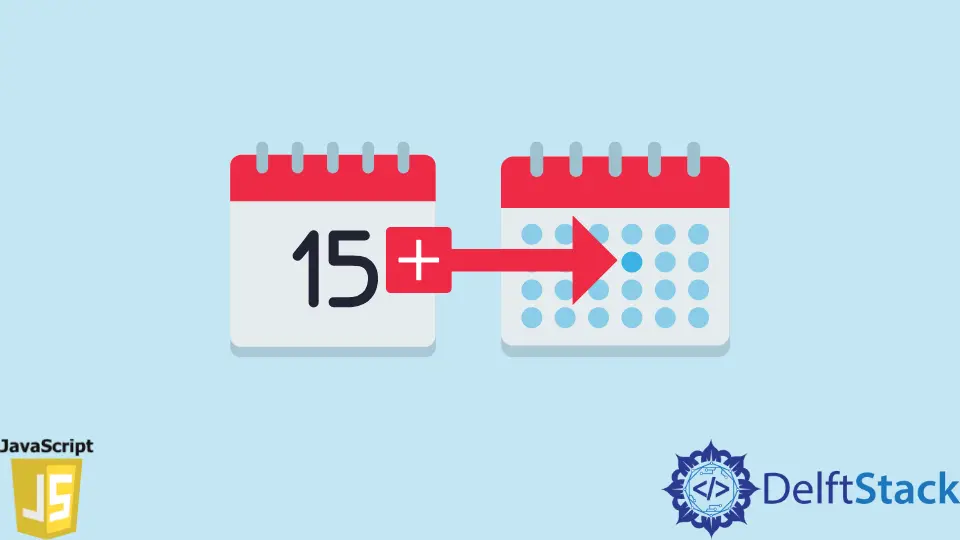
This tutorial will introduce how to add a new day to the current date or a custom date in JavaScript. We will first introduce what is Date
and different methods in JavaScript Date
class.
Understand JavaScript Date
Class
In JavaScript, the Date
class is basically the number of milliseconds that passed since midnight on January 1, 1970, UTC. It is not the same as the Unix epoch, which is used in computers to record date and time values.
To start using the Date
class, we need to create a new Date
object to deal with, and creating that object can be done in many ways as we can see in the following example:
var myDate = new Date();
var myDateOne = new Date('August 19, 2020 23:15:30');
var myDateTwo = new Date('2020-08-17T03:24:00');
var myDateThree = new Date(2020, 07, 17);
var myDateFour = new Date(2020, 07, 17, 3, 24, 0);
console.log(myDate);
console.log(myDateOne);
console.log(myDateTwo);
console.log(myDateThree);
console.log(myDateFour);
When we call the Date()
constructor without giving any parameters, the Date
object will have the current date and time; while when given a parameter, that object will have the parsed string representing that point of time.
If we give the appropriate date and time components value like in the example new Date(2020, 07, 17)
, the index should start from 0, not 1.
Output:
Fri Nov 13 2020 19:16:58 GMT+0200 (Eastern European Standard Time)
Mon Aug 17 2020 23:15:30 GMT+0200 (Eastern European Standard Time)
Mon Aug 17 2020 03:24:00 GMT+0200 (Eastern European Standard Time)
Mon Aug 17 2020 00:00:00 GMT+0200 (Eastern European Standard Time)
Mon Aug 17 2020 03:24:00 GMT+0200 (Eastern European Standard Time)
We frequently use many methods from the Date
class; we will explain some of them in the next sections.
Add Days to Date()
in JavaScript
Suppose we want to create a function that adds a certain number of days in JavaScript. In that case, we can implement it using the Date
class method named getDate()
which returns the day of the month, between 1 and 31, for the selected date according to local time, and the method setDate()
to set the day of the month for that specific date.
For example, if the date is 11/02/2020
, adding one day will be 12/02/2020
. If it was 31/03/2020
, it shall be 01/04/2020
as the only valid range is [1, 31]
.
Let’s see the following example of adding days to the Date
object.
function addDaysToDate(date, days) {
var res = new Date(date);
res.setDate(res.getDate() + days);
return res;
}
var tmpDate = new Date(2020, 07, 20); // Augest 20, 2020
console.log(addDaysToDate(tmpDate, 2));
Output:
Sat Aug 22 2020 00:00:00 GMT+0200 (Eastern European Standard Time)
If we are interested in adding that day to today, we can replace new Date(date)
with new Date()
in the above code, or we can pass the new Date()
to the function directly.
var tmpDate = new Date(); // Today
console.log(addDaysToDate(tmpDate, 2)); // today + 2
Output:
Sun Nov 15 2020 22:55:06 GMT+0200 (Eastern European Standard Time)
Add Days to Date()
Using prototype
in JavaScript
If we want to create a prototype to the Date
class that takes only the numeric input as the number of days to add, we can implement it as the following example:
Date.prototype.addDays =
function(noOfDays) {
var tmpDate = new Date(this.valueOf());
tmpDate.setDate(tmpDate.getDate() + noOfDays);
return tmpDate;
}
var myDate = new Date(); // today
console.log(myDate.addDays(2)); // today + 2
Output:
Sun Nov 15 2020 22:59:06 GMT+0200 (Eastern European Standard Time)
Related Article - JavaScript Date
- How to Add Months to a Date in JavaScript
- How to Add Minutes to Date in JavaScript
- How to Add Hours to Date Object in JavaScript
- How to Calculate Date Difference in JavaScript
- How to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
- How to Get First and Last Day of Month Using JavaScript