Inline if Statement in JavaScript
-
Simple Inline
if
Statement With Ternary Operation in JavaScript -
Multiple Condition Inline
if
Statement Ternary Operation in JavaScript -
Inline
if
Statement With Logical Operator in JavaScript
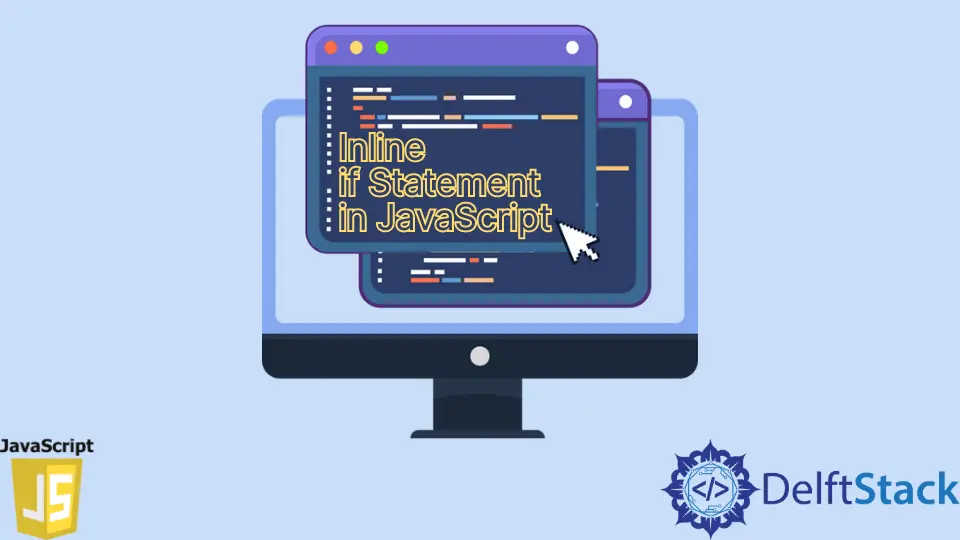
Conditional operation is one of the basic coding conceptions for any programming language. The convention is to infer the best fit output from multiple available conditions. JavaScript supports both the usual if...else
structure and the ternary operators.
In the following section, we will introduce how to apply conditional statements using ternary operators and logical operators.
Simple Inline if
Statement With Ternary Operation in JavaScript
Generally, this kind of coding practice states the base condition first and separates the possible outcome by a ?
. The possible results are set apart with a colon (:)
. Ternary operation structure only takes a single line of code to bring the result, thus named as inline if
statement.
Code Snippet:
var x = 5;
var y = 10;
var z = x > y ? x : y;
console.log(z);
Output:
The example explains the condition where the variable x
is compared with the variable y
. If x
is greater than y
, then z
holds the value of x
, other than that value of y
. This is the alternative to the basic if...else
structure.
Multiple Condition Inline if
Statement Ternary Operation in JavaScript
Multiple conditions refer to more than one condition; more specifically, it is the structure for if...else if...else
. Each condition scope will have a return case, and the applicable condition’s return value is the answer. The documentation gives a better preview for the case.
Code Snippet:
var x = 5;
var y = 5;
var z = x > y ? 'true' : x < y ? 'false' : 'false';
console.log(z);
Output:
According to the output, it is seen that here an if
statement (x>y)
was present also upon that there was a return case "true"
. Later for if else
, we have (x<y)
as the condition, and that returns "false"
. Also, if the applied condition gets matched, we return "false"
, which is our else
statement.
Inline if
Statement With Logical Operator in JavaScript
In this practice, a given condition that satisfies the return value is written after the (&&)
operator. And if it is directed to the else
condition, the return value is set after the ||
operator. Let’s see the demonstration with a code example.
Code Snippet:
var x = 5;
var y = 10;
var z = (x < y) && x || y;
console.log(z);
Output: