Equivalent of Ruby unless Statement in JavaScript
- Using Negation in If-Else Statements
- Creating a User-Defined Unless Function
- Using the Ternary Operator for Conditional Execution
- Conclusion
- FAQ
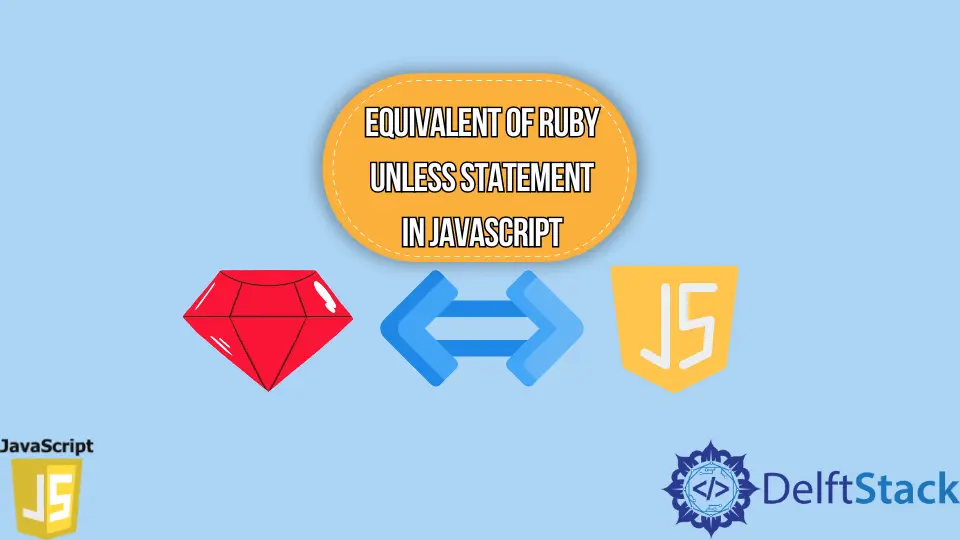
JavaScript, unlike Ruby, does not have a built-in unless
statement. Instead, it primarily relies on if-else
conditions and the ternary operator to control the flow of logic. For developers coming from a Ruby background, this might feel a bit limiting at first. However, there are effective ways to implement similar functionality in JavaScript. You can create a user-defined function or simply negate your conditions in an if
statement to mimic the behavior of unless
.
In this article, we’ll explore these methods in detail, providing clear examples and explanations to help you grasp how to achieve the equivalent of Ruby’s unless
in JavaScript.
Using Negation in If-Else Statements
One of the simplest ways to replicate the behavior of Ruby’s unless
in JavaScript is by using negation in an if
statement. This approach requires you to think in reverse: instead of executing code when a condition is true, you execute it when the condition is false. Here’s how you can implement this:
let isRaining = false;
if (!isRaining) {
console.log("It's a great day for a walk!");
}
Output:
It's a great day for a walk!
In this example, the variable isRaining
is set to false
. The if
statement checks the negated condition !isRaining
. Since isRaining
is false, the code inside the block executes, resulting in the message about the great day for a walk. This method is straightforward and aligns closely with the logic of Ruby’s unless
.
However, it’s essential to ensure that the condition you are negating accurately reflects the logic you want to implement. This method is particularly useful for simple conditions where readability is not compromised.
Creating a User-Defined Unless Function
If you prefer a more structured approach, you can create a user-defined function that behaves like Ruby’s unless
. This method encapsulates the logic in a reusable function, enhancing code organization and readability. Here’s how you can define such a function:
function unless(condition, callback) {
if (!condition) {
callback();
}
}
let isSunny = true;
unless(isSunny, function() {
console.log("Let's go to the beach!");
});
Output:
In this case, the unless
function takes two parameters: a condition
and a callback
. It checks if the condition is false. If it is, the callback function is executed. Since isSunny
is true
, the message about going to the beach does not display.
Creating a user-defined function adds clarity to your code, especially in larger projects where you might want to maintain a consistent style. This method allows you to write more expressive and readable code, closely resembling Ruby’s syntax and logic.
Using the Ternary Operator for Conditional Execution
Another way to mimic Ruby’s unless
is by using the ternary operator. This approach is particularly useful for inline conditions where you want to execute a short piece of code based on a condition. Here’s how you can implement this:
let isWeekend = false;
isWeekend ? console.log("Time to relax!") : console.log("Back to work!");
Output:
Back to work!
In this example, the ternary operator checks the isWeekend
variable. If it is true
, it logs “Time to relax!”. Since isWeekend
is false
, it executes the second part of the ternary operator, logging “Back to work!”.
While the ternary operator can be a concise way to handle conditions, it’s essential to use it judiciously. Overusing it can lead to code that is difficult to read and maintain. However, for simple conditions, it can be an elegant solution that reduces the need for longer if-else
statements.
Conclusion
While JavaScript does not have a direct equivalent to Ruby’s unless
, there are several effective methods to achieve similar functionality. Whether you choose to use negation in if
statements, create a user-defined function, or leverage the ternary operator, each approach allows you to maintain clean and readable code. Understanding these alternatives can enhance your programming skills and make your JavaScript code more expressive. By implementing these strategies, you can easily replicate the behavior of Ruby’s unless
statement in your JavaScript projects.
FAQ
-
What is the main difference between Ruby’s unless and JavaScript’s if statements?
Ruby’sunless
executes code when the condition is false, while JavaScript’sif
requires the condition to be true. -
Can I use unless in JavaScript directly?
No, JavaScript does not have a built-inunless
statement; you must implement it using other methods.
-
How do I create a user-defined unless function in JavaScript?
You can define a function that takes a condition and a callback, executing the callback if the condition is false. -
Is using negation in if statements a good practice?
Yes, it can be effective for simple conditions, but ensure it maintains code readability. -
When should I use the ternary operator in JavaScript?
Use the ternary operator for concise, inline conditions, but avoid overcomplicating your code with it.