JavaScript OR Condition in the if Statement
-
JavaScript
OR
Condition Example UsingIF
Statement -
Examine the Given
HTML
Code in Few Steps - Alternative Way to Get Same Results
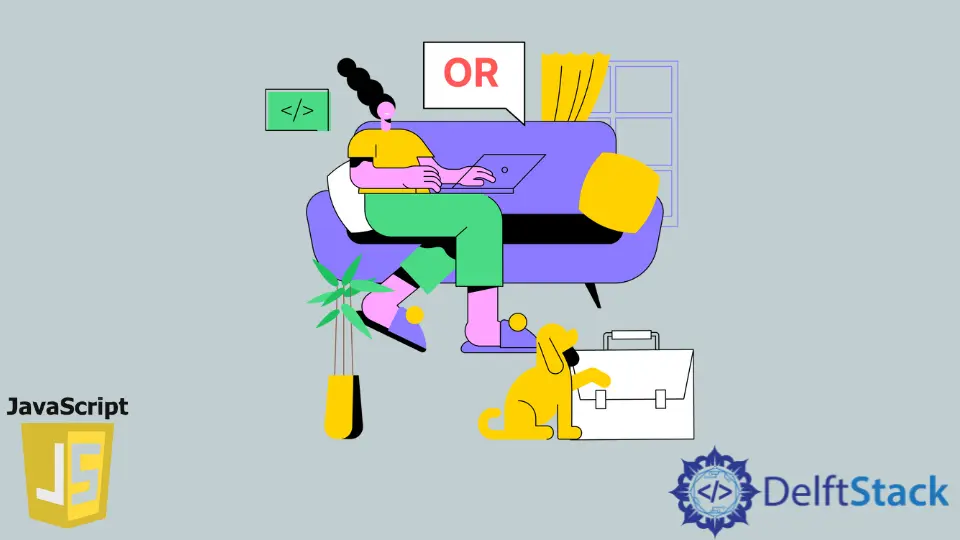
JavaScript contains conditional statements like if-else
, switch cases
, etc. These statements are conditional and used to check whether the given condition is true or not; for this, we use OR ||
and &&
operators.
Operator || (OR)
checks all conditions and determines if any condition is true or not. Are you confused? No worries, we will discuss it further with the help of an example to better understand || (OR)
Operator.
JavaScript OR
Condition Example Using IF
Statement
The below HTML
source code shows a form input that we will use to get the input string value from the user like any alphabet, and there is a button submit on which we have called JavaScript function checkVowel()
on click.
checkVowel()
function contains an if statement
condition with || (OR) operator
to check the user input alphabet is vowel or not.
<!DOCTYPE html>
<html>
<head>
<title>
HTML | Window Print() method example
</title>
<script type="text/javascript">
</script>
</head>
<body>
<h2>Hi Users Check your choosen alphabet is a vowel or not.</h2>
<form id="form" onsubmit="return false;">
<input type="text" id="userInput" maxlength="1" placeholder="Enter any alphabet" />
<input type="submit" onclick="checkVowel();" />
</form>
<script>
function checkVowel() {
var variable = document.getElementById("userInput").value;
if(variable =="a" || variable=="A"){
alert(variable+" is vowel")
}
else if(variable =="e" || variable=="E"){
alert(variable+" is vowel")
}
else if(variable =="i" || variable=="I"){
alert(variable+" is vowel")
}
else if(variable =="o" || variable=="O"){
alert(variable+" is vowel")
}
else if(variable =="u" || variable=="U"){
alert(variable+" is vowel")
}
else{
alert(variable+" is not vowel")
}
}
</script>sss
</body>s
<html>
In this HTML
page source, we have created a form input type of text to get the alphabet from the user.
You can see the submit button that triggers the checkVowel()
method.
In the body of the checkVowel()
method, we have implemented a few if-else
conditions with ||
(OR) operator. This will check the user given alphabet with upper case and lower case vowel both if any of the if
statement meets the required condition, the method will show a pop up alert message which is Given alphabet is a vowel
.
If the given value is not matched with any given condition, the program will execute the else
condition that shows an alert message Given alphabet is not a vowel
.
Examine the Given HTML
Code in Few Steps
Follow these four easy steps and get a clear understanding of the ||
(OR) operator.
-
Create a text document. You can use notepad or any other text editing tool.
-
Paste the given code in the created text file.
-
Save that text file with the extension of
.html
and open it with any default browser. -
You can see an input form field enter any alphabet and press the submit button; it will display an alert box that shows the message alphabet is vowel or not.
Alternative Way to Get Same Results
You can also achieve the same functionality with the switch
statement using the ||
(OR) operator, as shown below.
switch (variable) {
case 'a' || 'A':
alert(variable + ' is vowel')
break;
Just use switch
(variable) with case
containing ||
operator instead of if
statements.