How to Manipulate an Image in JavaScript
- Manipulate an Image in JavaScript
- Resize an Image in Javascript
- Crop an Image in JavaScript
- Invert an Image in JavaScript
- Apply Adjustable Noise to an Image in JavaScript
- Adjust Vibrance of an Image in JavaScript
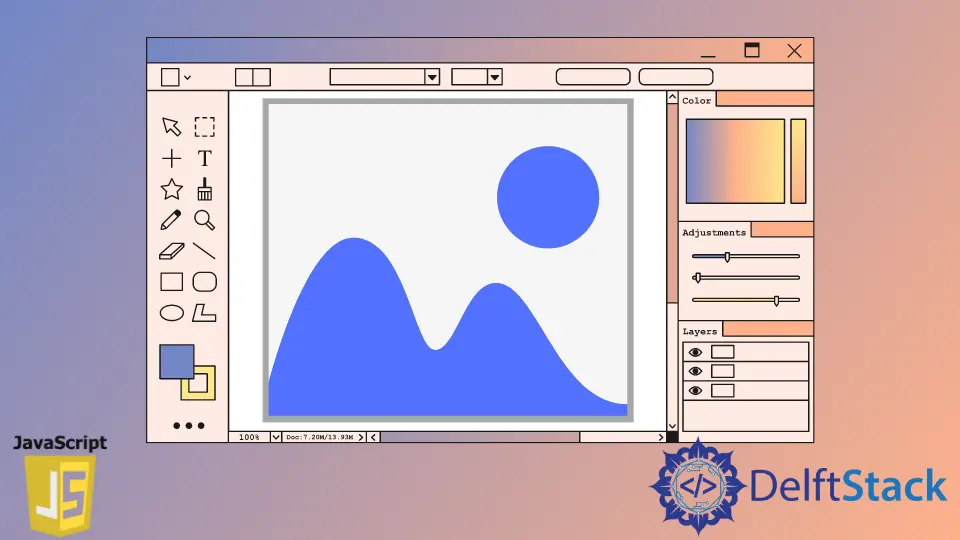
This article will introduce the image processing functions in JavaScript. We will use CamanJS, a JavaScript library, to manipulate the images.
Manipulate an Image in JavaScript
Image manipulation is used to perform various manipulation functions on an image. For example, we can increase the brightness of an image, add saturation on an image, resize an image, and many more.
To use CamanJs in your project, add the script
tag after the </body>
tag.
<script src="https://cdnjs.cloudflare.com/ajax/libs/camanjs/4.1.2/caman.full.min.js" integrity="sha512-JjFeUD2H//RHt+DjVf1BTuy1X5ZPtMl0svQ3RopX641DWoSilJ89LsFGq4Sw/6BSBfULqUW/CfnVopV5CfvRXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Then, we use the following code.
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Image Manipulation</title>
<link rel="stylesheet" href="https://bootswatch.com/4/flatly/bootstrap.min.css">
<!-- <link rel="stylesheet" href="css/style.css" /> -->
<style>
#canvas {
margin: auto;
background-color: #ececec;
width: 100%;
}
.btn-disable {
color: #fff !important;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8 m-auto">
<div class="custom-file mb-3">
<input type="file" class="custom-file-input" id="upload-file">
<label for="upload-file" class="custom-file-label">Choose Image</label>
</div>
<canvas id="canvas"></canvas>
<h4 class="text-center my-3">Filters</h4>
<div class="row my-4 text-center">
<div class="col-md-3">
<div class="btn-group btn-group-sm">
<button class="filter rmBrightness btn btn-info">-</button>
<button class="btn btn-secondary btn-disabled" disabled>Brightness</button>
<button class="filter addBrightness btn btn-info">+</button>
</div>
</div>
<div class="col-md-3">
<div class="btn-group btn-group-sm">
<button class="filter rmContrast btn btn-info">-</button>
<button class="btn btn-secondary btn-disabled" disabled>Contrast</button>
<button class="filter addContrast btn btn-info">+</button>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/camanjs/4.1.2/caman.full.min.js"
integrity="sha512-JjFeUD2H//RHt+DjVf1BTuy1X5ZPtMl0svQ3RopX641DWoSilJ89LsFGq4Sw/6BSBfULqUW/CfnVopV5CfvRXA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let img = new Image();
const uploadFile = document.getElementById("upload-file");
// Filter & Effect Handlers
document.addEventListener("click", e => {
if (e.target.classList.contains("filter")) {
if (e.target.classList.contains("addBrightness")) {
Caman("#canvas", img, function () {
this.brightness(5).render();
});
} else if (e.target.classList.contains("rmBrightness")) {
Caman("#canvas", img, function () {
this.brightness(-5).render();
});
} else if (e.target.classList.contains("addContrast")) {
Caman("#canvas", img, function () {
this.contrast(5).render();
});
} else if (e.target.classList.contains("rmContrast")) {
Caman("#canvas", img, function () {
this.contrast(-5).render();
});
}
}
});
// Upload File
uploadFile.addEventListener("change", () => {
// Get File
const file = document.getElementById("upload-file").files[0];
// Init FileReader API
const reader = new FileReader();
// Check for file
if (file) {
// Read data as URL
reader.readAsDataURL(file);
}
// Add image to canvas
reader.addEventListener(
"load",
() => {
// Create image
img = new Image();
// Set image src
img.src = reader.result;
// On image load add to canvas
img.onload = function () {
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0, img.width, img.height);
canvas.removeAttribute("data-caman-id");
};
},
false
);
});
</script>
</html>
Resize an Image in Javascript
To resize an image, use the code below.
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Image Manipulation</title>
<link rel="stylesheet" href="https://bootswatch.com/4/flatly/bootstrap.min.css">
<!-- <link rel="stylesheet" href="css/style.css" /> -->
<style>
#canvas {
margin: auto;
background-color: #ececec;
width: 100%;
}
.btn-disable {
color: #fff !important;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8 m-auto">
<div class="custom-file mb-3">
<input type="file" class="custom-file-input" id="upload-file">
<label for="upload-file" class="custom-file-label">Choose Image</label>
</div>
<canvas id="canvas"></canvas>
<h4 class="text-center my-3">Filters</h4>
<div class="row my-4 text-center">
<div class="col-md-3">
<div class="btn-group btn-group-sm">
<button class="filter resize btn btn-info"> Resize </button>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/camanjs/4.1.2/caman.full.min.js"
integrity="sha512-JjFeUD2H//RHt+DjVf1BTuy1X5ZPtMl0svQ3RopX641DWoSilJ89LsFGq4Sw/6BSBfULqUW/CfnVopV5CfvRXA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let img = new Image();
const uploadFile = document.getElementById("upload-file");
// Filter & Effect Handlers
document.addEventListener("click", e => {
if (e.target.classList.contains("filter")) {
if (e.target.classList.contains("resize")) {
Caman("#canvas", img, function () {
this.resize({
width: 1000,
height:200
}).render();
});
}
}
});
// Upload File
uploadFile.addEventListener("change", () => {
// Get File
const file = document.getElementById("upload-file").files[0];
// Init FileReader API
const reader = new FileReader();
// Check for file
if (file) {
// Read data as URL
reader.readAsDataURL(file);
}
// Add image to canvas
reader.addEventListener(
"load",
() => {
// Create image
img = new Image();
// Set image src
img.src = reader.result;
// On image load add to canvas
img.onload = function () {
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0, img.width, img.height);
canvas.removeAttribute("data-caman-id");
};
},
false
);
});
</script>
</html>
Crop an Image in JavaScript
We can also crop images using CamanJs. We must specify the width, height, and optionally, the top left corner coordinates where the cropping starts.
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Image Manipulation</title>
<link rel="stylesheet" href="https://bootswatch.com/4/flatly/bootstrap.min.css">
<style>
#canvas {
margin: auto;
background-color: #ececec;
width: 100%;
}
.btn-disable {
color: #fff !important;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8 m-auto">
<div class="custom-file mb-3">
<input type="file" class="custom-file-input" id="upload-file">
<label for="upload-file" class="custom-file-label">Choose Image</label>
</div>
<canvas id="canvas"></canvas>
<h4 class="text-center my-3">Filters</h4>
<div class="row my-4 text-center">
<div class="col-md-3">
<div class="btn-group btn-group-sm">
<button class="filter crop btn btn-info"> Crop </button>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/camanjs/4.1.2/caman.full.min.js"
integrity="sha512-JjFeUD2H//RHt+DjVf1BTuy1X5ZPtMl0svQ3RopX641DWoSilJ89LsFGq4Sw/6BSBfULqUW/CfnVopV5CfvRXA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let img = new Image();
const uploadFile = document.getElementById("upload-file");
// Filter & Effect Handlers
document.addEventListener("click", e => {
if (e.target.classList.contains("filter")) {
if (e.target.classList.contains("crop")) {
Caman("#canvas", img, function () {
this.crop(500, 300).render();
});
}
}
});
// Upload File
uploadFile.addEventListener("change", () => {
// Get File
const file = document.getElementById("upload-file").files[0];
// Init FileReader API
const reader = new FileReader();
// Check for file
if (file) {
// Read data as URL
reader.readAsDataURL(file);
}
// Add image to canvas
reader.addEventListener(
"load",
() => {
// Create image
img = new Image();
// Set image src
img.src = reader.result;
// On image load add to canvas
img.onload = function () {
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0, img.width, img.height);
canvas.removeAttribute("data-caman-id");
};
},
false
);
});
</script>
</html>
Invert an Image in JavaScript
Inverting an image subtracts each color channel value from 255. It doesn’t take any argument.
See the code below.
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Image Manipulation</title>
<link rel="stylesheet" href="https://bootswatch.com/4/flatly/bootstrap.min.css">
<!-- <link rel="stylesheet" href="css/style.css" /> -->
<style>
#canvas {
margin: auto;
background-color: #ececec;
width: 100%;
}
.btn-disable {
color: #fff !important;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8 m-auto">
<div class="custom-file mb-3">
<input type="file" class="custom-file-input" id="upload-file">
<label for="upload-file" class="custom-file-label">Choose Image</label>
</div>
<canvas id="canvas"></canvas>
<h4 class="text-center my-3">Filters</h4>
<div class="row my-4 text-center">
<div class="col-md-3">
<div class="btn-group btn-group-sm">
<button class="filter invert btn btn-info"> Invert </button>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/camanjs/4.1.2/caman.full.min.js"
integrity="sha512-JjFeUD2H//RHt+DjVf1BTuy1X5ZPtMl0svQ3RopX641DWoSilJ89LsFGq4Sw/6BSBfULqUW/CfnVopV5CfvRXA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let img = new Image();
const uploadFile = document.getElementById("upload-file");
// Filter & Effect Handlers
document.addEventListener("click", e => {
if (e.target.classList.contains("filter")) {
if (e.target.classList.contains("invert")) {
Caman("#canvas", img, function () {
this.invert().render();
});
}
}
});
// Upload File
uploadFile.addEventListener("change", () => {
// Get File
const file = document.getElementById("upload-file").files[0];
// Init FileReader API
const reader = new FileReader();
// Check for file
if (file) {
// Read data as URL
reader.readAsDataURL(file);
}
// Add image to canvas
reader.addEventListener(
"load",
() => {
// Create image
img = new Image();
// Set image src
img.src = reader.result;
// On image load add to canvas
img.onload = function () {
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0, img.width, img.height);
canvas.removeAttribute("data-caman-id");
};
},
false
);
});
</script>
</html>
Apply Adjustable Noise to an Image in JavaScript
We can also apply an adjustable noise to the image, ranging from 0 to infinity. The higher the value, the more noise is used.
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Image Manipulation</title>
<link rel="stylesheet" href="https://bootswatch.com/4/flatly/bootstrap.min.css">
<!-- <link rel="stylesheet" href="css/style.css" /> -->
<style>
#canvas {
margin: auto;
background-color: #ececec;
width: 100%;
}
.btn-disable {
color: #fff !important;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8 m-auto">
<div class="custom-file mb-3">
<input type="file" class="custom-file-input" id="upload-file">
<label for="upload-file" class="custom-file-label">Choose Image</label>
</div>
<canvas id="canvas"></canvas>
<h4 class="text-center my-3">Filters</h4>
<div class="row my-4 text-center">
<div class="col-md-3">
<div class="btn-group btn-group-sm">
<button class="filter rmNoise btn btn-info">-</button>
<button class="btn btn-secondary btn-disabled" disabled>Noise</button>
<button class="filter addNoise btn btn-info">+</button>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/camanjs/4.1.2/caman.full.min.js"
integrity="sha512-JjFeUD2H//RHt+DjVf1BTuy1X5ZPtMl0svQ3RopX641DWoSilJ89LsFGq4Sw/6BSBfULqUW/CfnVopV5CfvRXA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let img = new Image();
const uploadFile = document.getElementById("upload-file");
// Filter & Effect Handlers
document.addEventListener("click", e => {
if (e.target.classList.contains("filter")) {
if (e.target.classList.contains("addNoise")) {
Caman("#canvas", img, function () {
this.noise(5).render();
});
} else if (e.target.classList.contains("rmNoise")) {
Caman("#canvas", img, function () {
this.noise(-5).render();
});
}
}
});
// Upload File
uploadFile.addEventListener("change", () => {
// Get File
const file = document.getElementById("upload-file").files[0];
// Init FileReader API
const reader = new FileReader();
// Check for file
if (file) {
// Read data as URL
reader.readAsDataURL(file);
}
// Add image to canvas
reader.addEventListener(
"load",
() => {
// Create image
img = new Image();
// Set image src
img.src = reader.result;
// On image load add to canvas
img.onload = function () {
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0, img.width, img.height);
canvas.removeAttribute("data-caman-id");
};
},
false
);
});
</script>
</html>
Adjust Vibrance of an Image in JavaScript
Vibrance increases the intensity of colors on low-saturated images.
It ranges from -100 to 100. Values < 0 will desaturate the image while values > 0 will saturate it.
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Image Manipulation</title>
<link rel="stylesheet" href="https://bootswatch.com/4/flatly/bootstrap.min.css">
<!-- <link rel="stylesheet" href="css/style.css" /> -->
<style>
#canvas {
margin: auto;
background-color: #ececec;
width: 100%;
}
.btn-disable {
color: #fff !important;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-8 m-auto">
<div class="custom-file mb-3">
<input type="file" class="custom-file-input" id="upload-file">
<label for="upload-file" class="custom-file-label">Choose Image</label>
</div>
<canvas id="canvas"></canvas>
<h4 class="text-center my-3">Filters</h4>
<div class="row my-4 text-center">
<div class="col-md-3">
<div class="btn-group btn-group-sm">
<button class="filter rmVibrance btn btn-info">-</button>
<button class="btn btn-secondary btn-disabled" disabled>Vibrance</button>
<button class="filter addVibrance btn btn-info">+</button>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/camanjs/4.1.2/caman.full.min.js"
integrity="sha512-JjFeUD2H//RHt+DjVf1BTuy1X5ZPtMl0svQ3RopX641DWoSilJ89LsFGq4Sw/6BSBfULqUW/CfnVopV5CfvRXA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script>
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let img = new Image();
const uploadFile = document.getElementById("upload-file");
// Filter & Effect Handlers
document.addEventListener("click", e => {
if (e.target.classList.contains("filter")) {
if (e.target.classList.contains("addVibrance")) {
Caman("#canvas", img, function () {
this.vibrance(5).render();
});
} else if (e.target.classList.contains("rmVibrance")) {
Caman("#canvas", img, function () {
this.vibrance(-5).render();
});
}
}
});
// Upload File
uploadFile.addEventListener("change", () => {
// Get File
const file = document.getElementById("upload-file").files[0];
// Init FileReader API
const reader = new FileReader();
// Check for file
if (file) {
// Read data as URL
reader.readAsDataURL(file);
}
// Add image to canvas
reader.addEventListener(
"load",
() => {
// Create image
img = new Image();
// Set image src
img.src = reader.result;
// On image load add to canvas
img.onload = function () {
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0, img.width, img.height);
canvas.removeAttribute("data-caman-id");
};
},
false
);
});
</script>
</html>