How to Get Child Element in JavaScript
- Understanding the DOM Structure
-
Using
childNodes
Property -
Using
children
Property -
Using
firstChild
andlastChild
-
Using
querySelectorAll
- Conclusion
- FAQ
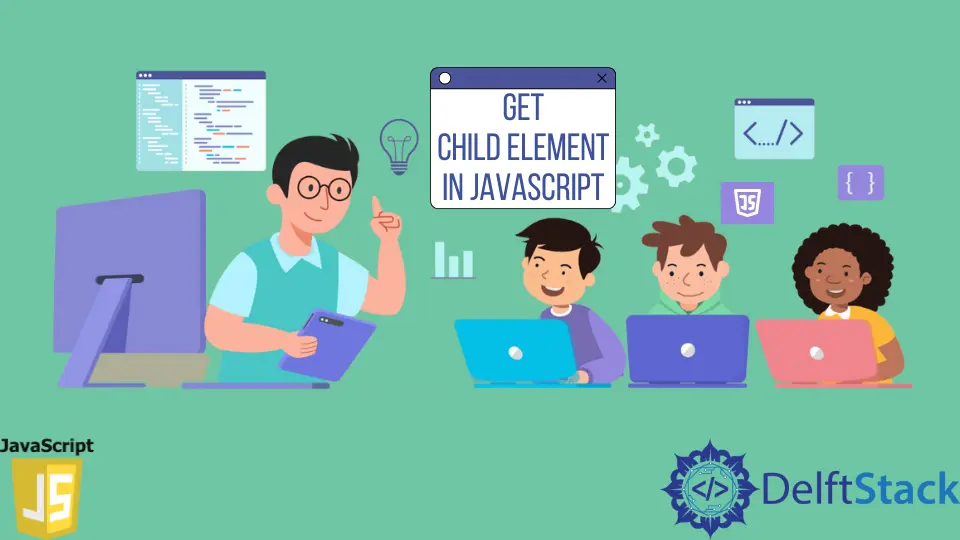
JavaScript is a powerful language that can manipulate the Document Object Model (DOM) of a web page. One common task developers face is retrieving child elements from a parent node.
This article will guide you through the various methods of getting child elements in JavaScript, specifically focusing on how to access child nodes from the body of an HTML document. Understanding these techniques is essential for anyone looking to enhance their web development skills. Whether you’re a beginner or an experienced developer, mastering child node retrieval will undoubtedly help you create more dynamic and interactive web pages.
Understanding the DOM Structure
Before diving into the methods of getting child elements, it’s important to understand the structure of the DOM. The DOM represents the document as a tree of nodes, where each node corresponds to a part of the document. The body of an HTML document is a parent node that contains various child nodes, including elements like paragraphs, divs, images, and more.
To effectively retrieve child nodes, you can utilize several JavaScript methods, each with its own specific use cases and advantages. Let’s explore these methods in detail.
Using childNodes
Property
One of the simplest ways to get child elements in JavaScript is by using the childNodes
property. This property returns a live NodeList of child nodes for a specified parent node. It includes all types of nodes: element nodes, text nodes, and comment nodes.
Here’s how you can use it:
const body = document.body;
const children = body.childNodes;
children.forEach(node => {
console.log(node);
});
Output:
#text
<div>Some content</div>
<p>Another content</p>
In this example, we first access the body of the document using document.body
. By calling body.childNodes
, we retrieve a list of all its child nodes. The forEach
method is then used to iterate through this list, logging each node to the console. Note that the output includes text nodes (like whitespace) alongside element nodes. This can be useful if you need to account for all child nodes, but if you’re only interested in element nodes, you might want to consider other methods.
Using children
Property
If you only want to retrieve element nodes and ignore text and comment nodes, the children
property is your best bet. This property returns a live HTMLCollection of the child elements of a specified parent node.
Here’s an example:
const body = document.body;
const children = body.children;
for (let i = 0; i < children.length; i++) {
console.log(children[i]);
}
Output:
<div>Some content</div>
<p>Another content</p>
In this code snippet, we again access the body of the document. However, this time we use body.children
, which gives us only the child elements. We then loop through the HTMLCollection with a for
loop and log each child element to the console. This method is particularly useful when you want to manipulate or analyze only the elements within the body, without the clutter of text or comment nodes.
Using firstChild
and lastChild
If you need to access just the first or last child of a parent node, you can use the firstChild
and lastChild
properties. These properties return the first and last child nodes of the specified parent node, respectively.
Here’s how you can use these properties:
const body = document.body;
const firstChild = body.firstChild;
const lastChild = body.lastChild;
console.log(firstChild);
console.log(lastChild);
Output:
#text
<p>Another content</p>
In this example, we access the first and last child nodes of the body. The firstChild
will typically return the first node in the body, which may be a text node due to whitespace. The lastChild
returns the last node, which is generally an element node. This method is useful when you need to specifically target the first or last element for manipulation or styling.
Using querySelectorAll
Another powerful method for retrieving child elements is using querySelectorAll
. This method allows you to select child elements based on CSS selectors, making it very versatile.
Here’s how you can use it:
const body = document.body;
const paragraphs = body.querySelectorAll('p');
paragraphs.forEach(p => {
console.log(p);
});
Output:
<p>Another content</p>
In this example, we use body.querySelectorAll('p')
to select all paragraph elements within the body. The result is a NodeList of matching elements, which we then iterate over with forEach
. This method is particularly useful when you want to target specific elements based on their tags, classes, or other attributes, providing a more refined approach to DOM manipulation.
Conclusion
Retrieving child elements in JavaScript is an essential skill for web developers. Whether you use the childNodes
property to get all types of nodes, the children
property for element-only access, or methods like firstChild
, lastChild
, and querySelectorAll
for more specific needs, each approach has its advantages. Understanding these methods will enhance your ability to create dynamic web applications that respond to user interactions effectively. Practice using these techniques, and soon you’ll be manipulating the DOM like a pro!
FAQ
-
What is the difference between
childNodes
andchildren
?
childNodes
includes all types of nodes (elements, text, comments), whilechildren
includes only element nodes. -
Can I use
firstChild
andlastChild
withchildren
?
No,firstChild
andlastChild
return the first and last nodes of any type, not limited to elements. -
How do I select child elements by class name?
You can usequerySelectorAll('.className')
to select elements with a specific class. -
Is
querySelectorAll
better thanchildren
?
It depends on your needs.querySelectorAll
is more versatile with selectors, whilechildren
is faster for direct access to child elements. -
Can I modify child elements once I retrieve them?
Yes, you can manipulate the properties and styles of child elements after retrieving them using any of the methods discussed.