How to Use Static Methods in Java
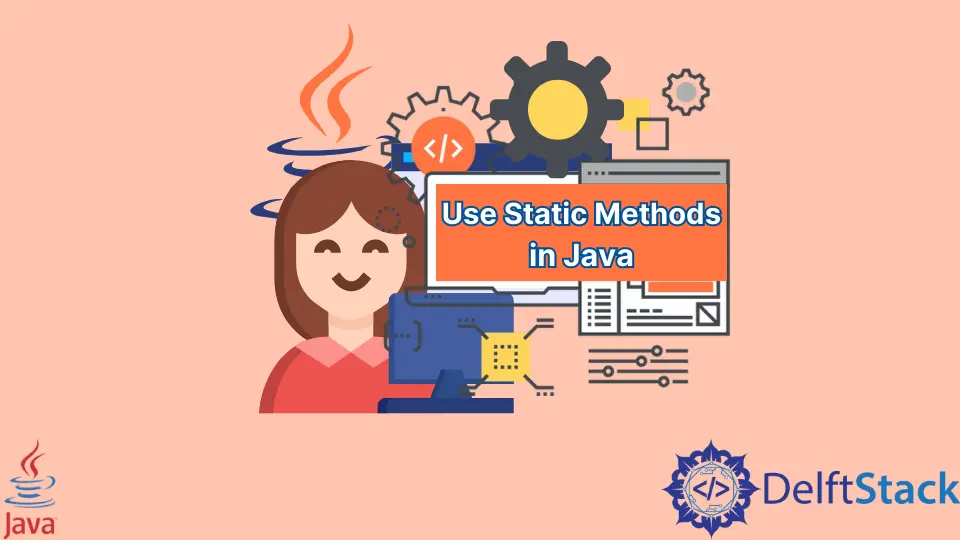
In Java, the static
keyword invokes a method or a variable without instantiating the instance of the class. You don’t have to make the instance variable, and the class name is directly used to make a call to the member variables and methods.
An example of such a public method is the main
method. The function does not need any object to call this command; hence, the program execution begins from the main.
Static methods or variables belong to a class instead of its object. You don’t need to create the instance of the class to call the static methods. Static members are also allowed to access the static variables and change the value of the same ones.
The code inside the static methods is less likely to change. Additionally, the static methods cannot get overridden. The code is less likely to change, so it must be extracted in the function.
The advantages gained from the static variables are listed below.
- The use of static variables increases the program performance. Once they are created, they get directly injected as and when needed.
- The method calls becomes easier when there is no need to add a
new
keyword along with the method name. Instead, the class name gets used to calling the methods.
Below is the program that demonstrates the use of static in Java.
public class Main {
public static void main(String[] args) {
College clg = new College();
clg.display();
System.out.println(clg.clgName);
System.out.println(College.getName());
}
}
class College {
static String name = "IJK";
String clgName = "IIT";
static String getName() {
// return clgName;
return name;
}
public void display() {
System.out.println("I am in College");
}
}
In the code block above, the use of the static method and static variables are present.
The code creates a public driver class named StaticMethodRepresentation
and a non-public College
class. The college class holds both static and non-static members. The driver function tries to create the instance of the College
class using the new
keyword. The new keyword invokes the public constructor of the College
class.
As no public constructor is defined, the JVM calls the default constructor in the class. The class instance is used to invoke the public display
function of the College
class; that gets invoked and prints the string present.
Similarly, using the instance of that class, one can access its member variable. But to access the static functions, there is no need to create a new class instance. Instead, the class name can be used to calling it. Hence, in the last print statement, the class name invokes the static function.
In the function definition, there is a commented line. The line tries to access the non-static member of the class. Still, static
members can access only the static members; hence, it is not feasible to access them. Therefore, a static string is returned from the function.
The output of the code block above, showing the static keyword usage, is shown below.
I am in College IIT IJK
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn