How to Resolve Unreported Exception IOException Must Be Caught or Declared to Be Thrown in Java
-
Addressing
unreported exception IOException
Error in Java -
Resolving
Unreported Exception IOException
in Java: Methods and Solutions -
Best Practices for Exception Handling in Java to Avoid
Unreported Exception IOException
- Conclusion
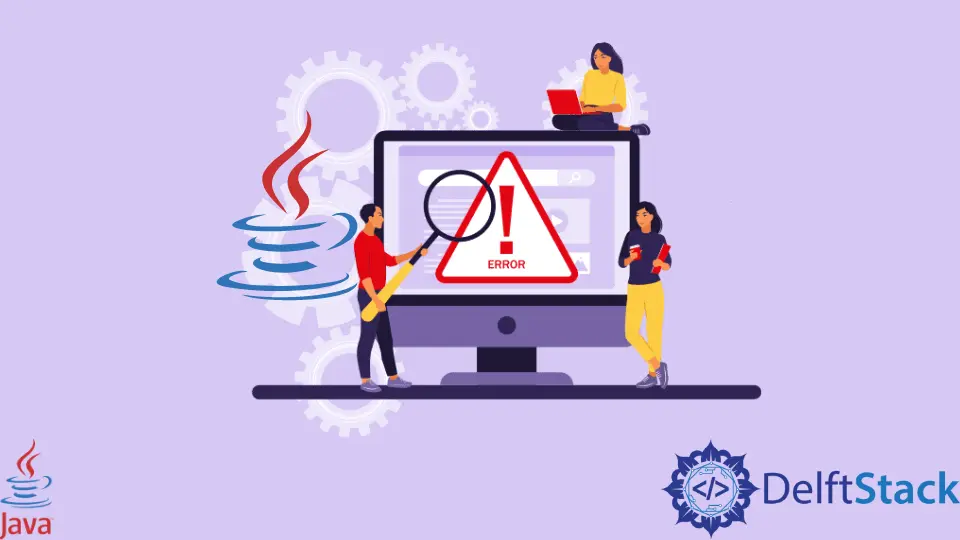
This article comprehensively addresses the causes, solutions, and effective methods for resolving the Unreported Exception IOException
error in Java, while also highlighting best practices to prevent its occurrence.
Addressing unreported exception IOException
Error in Java
Resolving the unreported exception IOException
error in Java is crucial for program stability and reliability. IOExceptions
represent issues with input/output operations, such as file handling.
Ignoring or neglecting this error can lead to unexpected program crashes or incorrect behavior, as vital error information is left unhandled. By addressing this error, developers ensure proper handling of potential issues, enhancing the robustness of their applications.
It also promotes cleaner code, making it more maintainable and comprehensible for both developers and users, ultimately contributing to a smoother and more dependable software experience.
The unreported exception IOException; must be caught or declared to be thrown
error in Java typically occurs when there is code that may throw an IOException
, but the exception is not properly handled. Here are various causes of this error along with corresponding solutions:
Causes | Solutions |
---|---|
IO operation without proper handling | Use try-catch blocks to handle IOException or declare the method to throw IOException . |
Inadequate handling of checked exceptions | Add a try-catch block or declare the method to throw IOException as appropriate. |
Missing throws declaration in method signature | Add a throws declaration to the calling method or handle the exception using try-catch . |
Incorrect exception type handling | Ensure that the catch block specifies IOException or its specific subtypes. |
By addressing these causes and applying the suggested solutions, you can effectively resolve the unreported exception IOException
error in your Java code. Choose the solution that best fits the context and requirements of your application.
The heading can be improved for clarity and conciseness. Consider:
Resolving Unreported Exception IOException
in Java: Methods and Solutions
throws
Declaration
Using throws
declaration in Java is vital for signaling potential IOExceptions
to calling code. Unlike try-catch
blocks, it shifts the responsibility of handling exceptions to the caller, promoting cleaner and more modular code.
This approach enhances code readability and reduces redundancy, making it a concise and effective method for addressing unreported exception IOException
.
Example Code:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class IOExceptionThrowsExample {
public static void main(String[] args) {
try {
readFile("example.txt");
} catch (IOException e) {
System.err.println("An error occurred while reading the file: " + e.getMessage());
}
}
public static void readFile(String filename) throws IOException {
// Code that may throw IOException
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
// Close the resources
reader.close();
}
}
In the provided code explanation, we first import necessary classes from the java.io
package, including BufferedReader
, FileReader
, and IOException
. The example code is encapsulated within a class named IOExceptionThrowsExample
.
The main
method demonstrates calling the readFile
method and handling potential IOException
using a try-catch
block. Within the readFile
method, we declare that it may throw an IOException
, and inside the method, a BufferedReader
is used to read the content of a specified file within a while
loop.
Finally, we ensure proper resource management by closing the BufferedReader
after completing the IO operation.
Output:
Successful Execution of the Code | IOException Handling in the Code |
---|---|
![]() |
![]() |
Suppose the file example.txt
is present and readable. In that case, the program will successfully read and print its content to the console. If an IOException
occurs during the file reading process, the catch
block in the main
method will be executed, providing a descriptive error message.
The example demonstrates how the throws
declaration enhances code readability and facilitates a robust approach to exception management in Java.
Try-Catch
Handling
Using try-catch
blocks in Java is essential for handling IOExceptions
locally within the code. It ensures graceful error handling, preventing unexpected program termination.
Unlike throws declarations, try-catch
allows immediate response and tailored error-handling strategies, making it a concise and effective method for resolving unreported exception IOException
.
Example Code:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class IOExceptionTryCatchExample {
public static void main(String[] args) {
readFile("example.txt");
}
public static void readFile(String filename) {
// Code that may throw IOException
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
// Close the resources
reader.close();
} catch (IOException e) {
// Handle the IOException
System.err.println("An error occurred during file reading: " + e.getMessage());
}
}
}
The provided code explanation involves importing necessary classes from the java.io
package, including BufferedReader
, FileReader
, and IOException
. The example code is encapsulated within a class named IOExceptionTryCatchExample
.
In the main
method, the readFile
method is invoked, which may throw an IOException
. Instead of explicitly catching the exception in the main
method, it relies on the try-catch
block within the readFile
method.
Inside the readFile
method, an IOException
is handled using a try-catch
block. The try
block contains code to open a BufferedReader
and read the content of a specified file within a while
loop.
If an IOException
occurs during this process, the catch
block handles it by printing an error message with the exception’s details. Additionally, resources are closed within a finally
block to ensure proper resource management, regardless of whether an exception occurs or not.
Output:
Successful Execution of the Code | IOException Handling in the Code |
---|---|
![]() |
![]() |
Suppose the file example.txt
is present and readable. In that case, the program will successfully read and print its content to the console.
If an IOException
occurs during the file reading process, the catch
block in the readFile
method will be executed, providing a descriptive error message.
In this example, we have demonstrated how encapsulating code that may throw an IOException
within a try-catch
block can effectively resolve the unreported exception IOException
error.
Multiple Exceptions Handling
Handling multiple exceptions in Java provides versatility by allowing specific responses to various exceptional scenarios, such as IOExceptions
. Unlike single-catch approaches, it tailors error-handling strategies based on the type of exception, enhancing code precision and resilience.
This method ensures a comprehensive approach to resolving unreported exception IOException
with simplicity and effectiveness.
Example Code:
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class IOExceptionMultipleExceptionsExample {
public static void main(String[] args) {
try {
readFile("example.txt");
} catch (FileNotFoundException e) {
System.err.println("File not found: " + e.getMessage());
} catch (IOException e) {
System.err.println("An error occurred during file reading: " + e.getMessage());
}
}
public static void readFile(String filename) throws FileNotFoundException, IOException {
// Code that may throw FileNotFoundException or IOException
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
// Close the resources
reader.close();
}
}
The code starts by importing necessary classes from the java.io
package, including BufferedReader
, FileReader
, FileNotFoundException
, and IOException
. It is encapsulated within a class named IOExceptionMultipleExceptionsExample
.
In the main
method, the readFile
method is invoked, capable of throwing both FileNotFoundException
and IOException
.
Each exception type is handled separately using multiple catch
blocks. The readFile
method declares its potential exceptions in its signature.
Inside the try
block, a BufferedReader
is used to read the content of a specified file within a while
loop. If a FileNotFoundException
or IOException
occurs during this process, the corresponding catch
block is executed.
Regardless of exceptions, resources are closed within a finally
block for proper resource management.
Output:
Successful Execution of the Code | IOException Handling in the Code |
---|---|
![]() |
![]() |
Suppose the file example.txt
is present and readable. In that case, the program will successfully read and print its content to the console.
If the file is not found, the FileNotFoundException catch
block will be executed, providing a specific error message. If an IOException
occurs during the file reading process, the IOException catch
block will be executed, providing a more general error message.
In this example, we’ve illustrated how to handle both FileNotFoundException
and IOException
when resolving the unreported exception IOException
error.
Best Practices for Exception Handling in Java to Avoid Unreported Exception IOException
Handle or Declare
Always handle checked exceptions, like IOException
, using try-catch
blocks or declare them in the method signature using the throws
clause.
Specific Exception Handling
Be specific in catching exceptions. Instead of catching a general Exception
, catch specific exceptions relevant to the operation, such as IOException
.
Use try-with-resources
For resource management, use try-with-resources
when dealing with classes implementing AutoCloseable
to ensure proper closing without explicit close statements.
Consistent Exception Handling
Maintain consistency in exception handling across your codebase. Adopt a unified approach to handle exceptions for better readability and maintainability.
Proper Resource Management
Ensure proper resource management by closing files, streams, or connections in a finally
block or using try-with-resources
to avoid resource leaks.
Thorough Testing
Rigorously test your code, especially scenarios where IOExceptions
may occur. Robust testing helps identify and address potential issues before deployment.
Document Exception Handling
Document the reasons for choosing specific exception-handling strategies. Clear documentation helps other developers understand the rationale behind your choices.
Use Custom Exceptions
Consider using custom exceptions for specific application-related issues. This enhances code clarity and allows for more targeted exception handling.
Graceful Error Reporting
Provide meaningful error messages in catch blocks to facilitate effective debugging and provide users with clear information about encountered issues.
Code Review
Regularly conduct code reviews to ensure adherence to exception-handling best practices. A fresh set of eyes can catch potential issues or suggest improvements.
Conclusion
Understanding the causes and solutions for the Unreported Exception IOException
error in Java is essential for building robust and error-resilient applications. By exploring various methods, including throws
declarations, try-catch
blocks, and handling multiple exceptions, developers can tailor their approach to specific scenarios.
Adopting best practices, such as consistent exception handling, thorough testing, and proper resource management, ensures code reliability. Emphasizing the importance of documentation and custom exceptions further contributes to clear and maintainable code.
Applying these insights collectively empowers developers to both address and proactively prevent the occurrence of unreported exception IOException
, promoting the creation of stable and user-friendly Java applications.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack