How to Resolve Java.Lang.RuntimeException: Unable to Instantiate Activity ComponentInfo
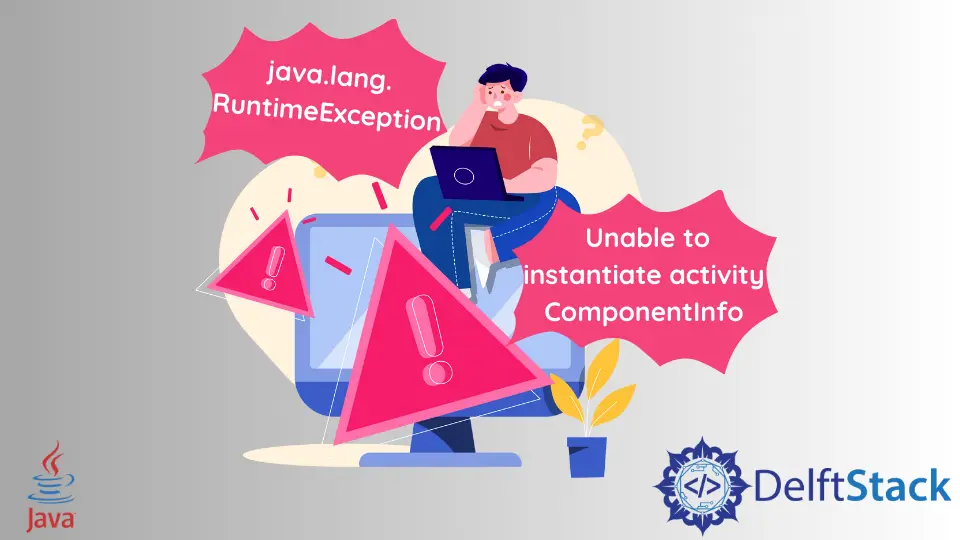
Today, we will learn about another runtime exception that says Unable to instantiate activity ComponentInfo
.
We will explore different possible reasons that result in java.lang.RuntimeException: Unable to instantiate activity ComponentInfo
. Finally, we will have a solution to eradicate it.
Resolve the java.lang.RuntimeException: Unable to instantiate activity ComponentInfo
Error
Example Code for Error Demonstration (MainActivity.java
file):
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
// write your remaining code here
}
Example Code (AndroidManifest.xml
file):
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.example.app">
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.App"
tools:targetApi="31">
<activity
android:name="MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
When we try to run this while launching an application in the android emulator, we get the error saying java.lang.RuntimeException: Unable to instantiate activity ComponentInfo
.
There are a few possible causes that we must consider to resolve this. All of them are listed below.
-
Make sure that your
Activity
is added to theAndroidManifest.xml
file. Why is it necessary?It is because whenever we want to make a new
Activity
, we must register in ourAndroidManifest.xml
file. Also, verify all the access modifiers. -
We also get this error when we try to view before
onCreate()
, which is incorrect and results in an error stating unable to instantiate activity component info error. -
Another reason for getting
java.lang.RuntimeException: Unable to instantiate activity ComponentInfo
is that we have added ourActivity
inAndroidManifest.xml
, which is declared asabstract
. In other words, we can say that theActivity
we are trying to access is declaredabstract
. -
Make sure that we are not missing a preceding dot before an activity path (this thing is causing an error in the example code given above).
-
We also have to face this error if we did not declare our
MainActivity.java
file aspublic
. Also, check if your file is in the right package or not.
Now, we know all the possible reasons. How can we fix it?
See the following solution.
Example Code for Solution (MainActivity.java
file):
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
// write your remaining code here
}
Example Code (AndroidManifest.xml
file):
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="com.example.app">
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.App"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
We have added a preceding dot before an activity path. We also confirm that our MainActivity.java
file is declared as public
, and we are not trying to access an Activity
declared as abstract
.
Be careful of all the points given as causes because those points are actual solutions if we consider them.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack