How to Fix Error: The System Cannot Find the File Specified in Java
Sheeraz Gul
Feb 02, 2024
Java
Java Error
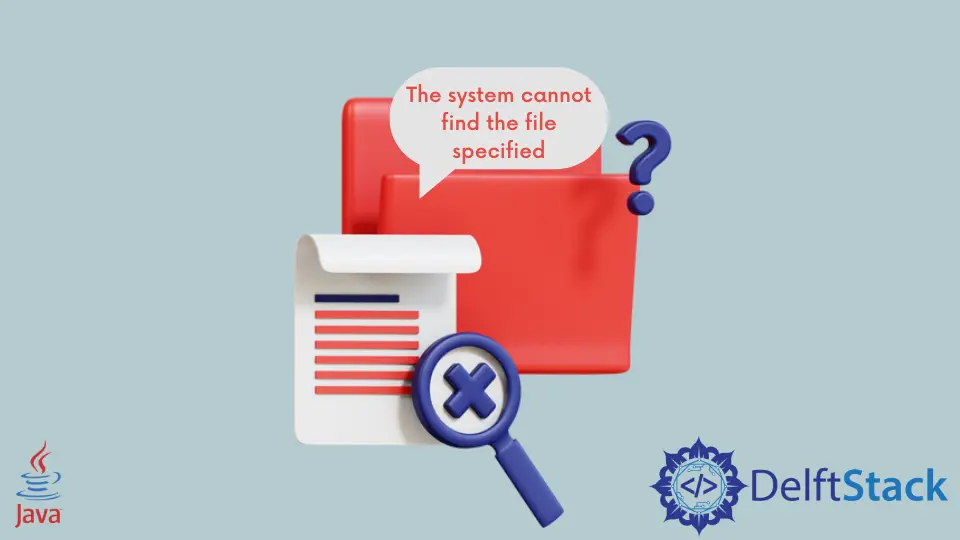
This tutorial demonstrates solving the The system cannot find the file specified
error in Java.
Fix the The system cannot find the file specified
Error in Java
The Java error The system cannot find the file specified
occurs when a file we are loading doesn’t occur in the directory or the file name is incorrect. It is also an exception from the Java IO package, which is thrown when the system cannot find a file with the given name.
Let’s try an example that will throw this same error:
package delftstack;
import java.io.*;
public class Example {
public static void main(String[] args) {
try {
File NewFile = new File("NewDelftstack.txt");
System.out.println(NewFile.getCanonicalPath());
FileInputStream File_Input_Stream = new FileInputStream(NewFile);
DataInputStream Data_Input_Stream = new DataInputStream(File_Input_Stream);
BufferedReader Buffered_Reader = new BufferedReader(new InputStreamReader(Data_Input_Stream));
String line;
while ((line = Buffered_Reader.readLine()) != null) {
System.out.println(line);
}
Data_Input_Stream.close();
} catch (Exception e) {
System.err.println("Error: " + e.getMessage());
}
}
}
The file NewDelftstack.txt
is not in the directory, so the above code will throw the error. See output:
C:\Users\Sheeraz\eclipse-workspace\Demos\NewDelftstack.txt
Error: NewDelftstack.txt (The system cannot find the file specified)
To solve this issue, ensure you have entered the correct file name and path. We can also check the list of files in Java which will help us if we have entered the right name and path.
See example:
package delftstack;
import java.io.*;
public class Example {
public static void main(String[] args) {
try {
File file = new File(".");
for (String fileNames : file.list()) System.out.println(fileNames);
File NewFile = new File("NewDelftstack.txt");
System.out.println(NewFile.getCanonicalPath());
FileInputStream File_Input_Stream = new FileInputStream(NewFile);
DataInputStream Data_Input_Stream = new DataInputStream(File_Input_Stream);
BufferedReader Buffered_Reader = new BufferedReader(new InputStreamReader(Data_Input_Stream));
String line;
while ((line = Buffered_Reader.readLine()) != null) {
System.out.println(line);
}
Data_Input_Stream.close();
} catch (Exception e) {
System.err.println("Error: " + e.getMessage());
}
}
}