The pack() Method in Java
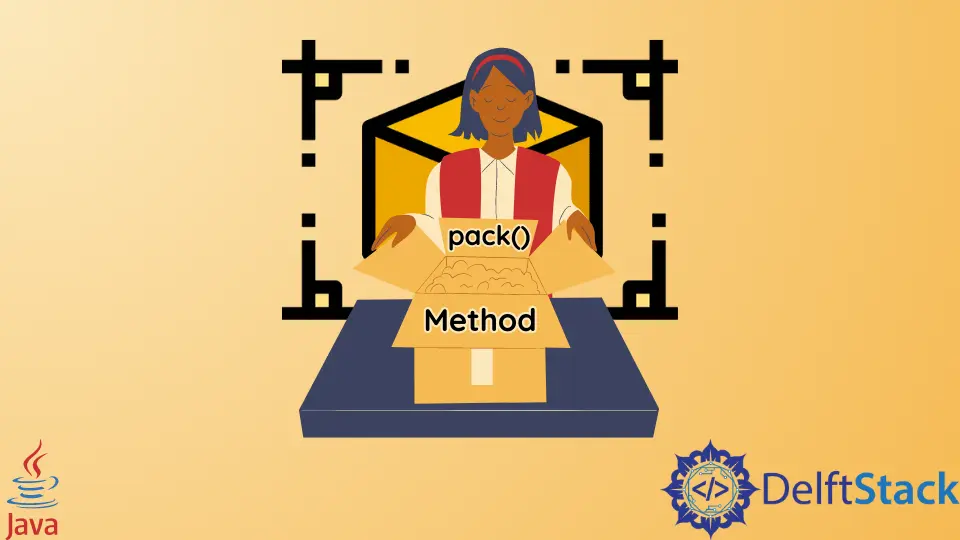
Defined in the Window class in Java, the pack()
method sizes the frame such that the frame’s contents are at or above their preferred sizes.
We can replace the pack()
method by defining the frame size explicitly. To do this, we use the setSize()
or setBounds()
methods. The setBounds()
method also sets the frame location.
But these methods do not leave the frame layout manager in charge of the frame size, unlike the pack()
method. The frame layout manager can adjust to different platforms and other factors that affect the component size. Therefore, it is advisable to use the pack()
method.
Syntax:
public void pack()
The pack()
method binds all the components together. To see how it works, let us look at a working example. We will make a button that returns some text in the text field on clicking it.
import java.awt.event.*;
import javax.swing.*;
public class ButtonDemo {
public static void main(String[] args) {
JFrame jf = new JFrame("Button Example");
final JTextField tf = new JTextField();
// using setBounds() method for textfield
tf.setBounds(50, 50, 150, 20);
JButton b = new JButton("Click this button");
// using setBounds() method for Button
b.setBounds(50, 100, 95, 30);
b.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
tf.setText("Java is interesting.");
}
});
jf.add(b);
jf.add(tf);
jf.setSize(300, 300);
jf.setLayout(null);
jf.setVisible(true);
}
}
Output:
Note that we are not using the pack()
method anywhere in this example. Instead, we make use of the setBounds()
method. The arguments of the setBounds()
method for the text field are 50, 50, 150, 20
and for the button, they are 50, 100, 95, 30
.
Also, note that the button is unable to display the entire text. We will look into this too in the below sections. We know that the layout managers automatically set the size and position of added components.
Since the pack()
method is the one that makes the layout managers in charge of the frame, we need to use the setBounds()
method in its absence.
Use of the SetBounds()
Method in Java
To use this in place of the pack()
method, we need to pass arguments for specifying the position and size of the components inside the main window.
It takes four arguments. The first two are for specifying the position(top-left)
of the component and the last two are for specifying the size(width-height)
of the component.
Syntax:
setBounds(int x - coordinate, int y - coordinate, int width, int height)
Look at the following piece of code in the above example.
jf.setLayout(null);
When we use the setBounds()
method, we set the layout manager
as null.
Use of the Pack()
Method in Java
Now, look at one more example that uses the pack()
method.
import java.awt.*;
import javax.swing.*;
public class ButtonDemo extends JFrame {
public ButtonDemo() { // This is the name of the class
// This will appear in the title bar of output window
setTitle("Buttons using pack()");
setLayout(new FlowLayout());
setButton();
// This calls the pack method
pack();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
void setButton() {
for (int i = 1; i < 9; i++) {
add(new JButton("Button" + i));
}
}
public static void main(String args[]) {
new ButtonDemo();
}
}
Output:
In this example, we are not using the setBounds()
method to set the position and size of the components. Instead, we call the pack()
method, which takes care of the size and position of components on its own.
Here, the ButtonDemo
class extends the JFrame
class. The JFrame
class is a container that acts as a window for holding the various components of the GUI like buttons, labels, and text fields.
Also, we can use the following code in place of the for
loop, but it unnecessarily increases the code length.
JButton b1 = new JButton("Button 1");
JButton b2 = new JButton("Button 2");
JButton b3 = new JButton("Button 3");
JButton b4 = new JButton("Button 4");
JButton b5 = new JButton("Button 5");
JButton b6 = new JButton("Button 6");
JButton b7 = new JButton("Button 7");
JButton b8 = new JButton("Button 8");
add(b1);
add(b2);
add(b3);
add(b4);
add(b5);
add(b6);
add(b7);
add(b8);
To know more about making a frame using Java, refer to this documentation.
If we remove the pack()
method, we will get the output, but the buttons are visible only when resizing the panel. This is the output when we do not use either pack()
or setBounds()
methods.
We can resize it to see the buttons.
But this is not a good representation, and therefore, we must use either of the pack()
or setBounds()
methods. The pack()
method is easy to use and precise and serves the purpose in just one line of code.
The setBounds()
method needs the x
and y
coordinates as arguments. This might need a lot of changing and readjusting because determining the coordinates at once is often not easy.
Also, the pack()
method adjusts the button’s size accordingly, but the setBounds()
method cannot do that on its own. We need to pass different arguments for width and height for different text lengths inside the button.
Look at the output of the first example again.
The button cannot display the complete text Click this button
because the size of the button is smaller than the requirement of this text. To rectify this, we will have to change the value of the width argument.
But if we use the pack()
method, it will resize the button on its own as per the text inside it.
import java.awt.*;
import javax.swing.*;
public class ButtonDemo extends JFrame {
public ButtonDemo() {
setTitle("Using the pack() method");
setLayout(new FlowLayout());
setButton();
// Calling the pack() method
pack();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
}
void setButton() {
for (int i = 1; i < 7; i++) {
add(new JButton("Click this Button" + i));
}
}
public static void main(String args[]) {
new ButtonDemo();
}
}
Output:
Note that the entire text fits inside the buttons without having to resize it manually. This is the advantage of using the pack()
method over the setBounds()
method.
To know more about making a GUI using Swing, refer to this documentation.
Conclusion
The pack()
method makes the layout manager in charge, which resizes and positions the components on the panel properly.
We can use the setBounds()
method as an alternative to the pack()
method but the use of the pack()
method is preferred because it easily adjusts to changes on its own, unlike the setBounds()
method. The pack()
method is necessary to bind all the components together.