The Method Is Undefined for the Type Error in Java
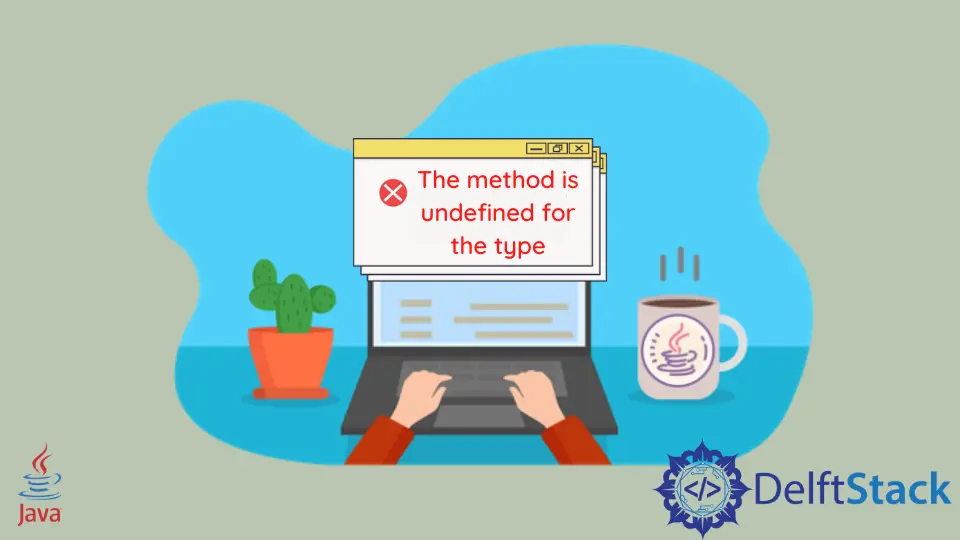
Java is a versatile programming language, but even seasoned developers can run into frustrating errors. One such error is the “method is undefined for the type” message, which can leave you scratching your head.
This tutorial aims to demystify this error, providing insights into its causes and effective solutions. Whether you’re a beginner or an experienced coder, understanding this error will enhance your Java programming skills. By the end of this article, you’ll be equipped to identify and fix this common issue, ensuring your Java applications run smoothly.
Understanding the Error
The “method is undefined for the type” error in Java typically occurs during compilation. It indicates that the Java compiler cannot find a method that you are trying to call on a particular object or class. This can happen for several reasons, such as a typo in the method name, incorrect method parameters, or attempting to call a method on an incompatible object type.
For instance, if you attempt to invoke a method that doesn’t exist in the class, the compiler will throw this error. Additionally, if you’re working with inheritance, the method might be defined in a superclass but not in the subclass you are currently using. Understanding the context of this error is crucial for resolving it effectively.
Common Causes of the Error
Typographical Errors
One of the most common reasons for encountering the “method is undefined for the type” error is a simple typo in the method name. Java is case-sensitive, meaning that myMethod()
and mymethod()
are treated as different identifiers. Always double-check your method calls to ensure they match the defined method names exactly.
Incorrect Object Type
Another frequent cause is calling a method on the wrong object type. If you’re trying to call a method that belongs to a different class, the compiler will not recognize it. For example, if you have a method defined in a class but are trying to call it on an instance of a different class, you will encounter this error.
Method Signature Mismatch
The method signature includes the method name and its parameter types. If you call a method with the wrong number or type of arguments, the compiler won’t find a matching method, resulting in this error. Always ensure that the arguments you pass match the method’s expected parameters.
Solutions to Fix the Error
Solution 1: Check Method Names and Parameters
One of the first steps in resolving this error is to carefully check the method names and parameters. Make sure that you are calling the method with the correct name and that the parameters match the expected types.
public class Example {
public void displayMessage(String message) {
System.out.println(message);
}
public static void main(String[] args) {
Example example = new Example();
example.displayMessage("Hello, World!");
}
}
Output:
Hello, World!
In this code, we define a method displayMessage
that takes a String
parameter. When we call this method in the main
function, we ensure that the parameter type matches what is defined. If we had called example.displayMessage(5);
, we would encounter the “method is undefined for the type” error because an integer does not match the expected String
type.
Solution 2: Verify Object Types
If you suspect that the error might be due to using an incorrect object type, verify the type of the object you are calling the method on. Make sure that the object is an instance of the class where the method is defined.
class Animal {
public void makeSound() {
System.out.println("Animal sound");
}
}
class Dog extends Animal {
public void makeSound() {
System.out.println("Bark");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.makeSound();
}
}
Output:
Bark
In this example, we have a superclass Animal
and a subclass Dog
. We create an Animal
reference that points to a Dog
object. When we call makeSound()
, the correct method is invoked due to polymorphism. If we had tried to call a method that only exists in Dog
on an Animal
reference, we would face the “method is undefined for the type” error.
Solution 3: Use Proper Inheritance
Inheritance can sometimes lead to confusion if methods are not properly overridden or if the subclass does not inherit the method correctly. Ensure that the method you are trying to call is either inherited or defined in the subclass.
class Parent {
public void show() {
System.out.println("Parent class");
}
}
class Child extends Parent {
public void show() {
System.out.println("Child class");
}
}
public class Main {
public static void main(String[] args) {
Parent child = new Child();
child.show();
}
}
Output:
Child class
In this case, the show()
method is defined in both the Parent
and Child
classes. When we create a Parent
reference pointing to a Child
object and call show()
, it invokes the Child
class’s version of the method. If we had not overridden the method in the Child
class, we would have encountered the error when trying to call a method that was not defined in the Child
class.
Conclusion
The “method is undefined for the type” error in Java can be frustrating, but understanding its causes and solutions can help you navigate through it with ease. By checking method names, verifying object types, and ensuring proper inheritance, you can effectively resolve this error. Remember, attention to detail is key in programming. With practice and awareness, you can avoid this common pitfall and enhance your Java coding skills.
FAQ
-
what causes the method is undefined for the type error?
The error is caused by calling a method that does not exist for a particular object type, often due to typos, incorrect object types, or mismatched method signatures. -
how can I check if a method exists in a class?
You can refer to the class documentation or use an IDE that provides auto-completion and method suggestions to verify if the method exists. -
can this error occur in interfaces?
Yes, if a class does not implement all the methods defined in an interface, you may encounter this error when trying to call those methods. -
how do I fix a signature mismatch error?
Ensure that the method call has the correct number and types of arguments that match the method definition. -
is this error specific to Java?
While the error message may vary, similar issues can occur in other programming languages when a method is called incorrectly.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack