Test Private Method in Java
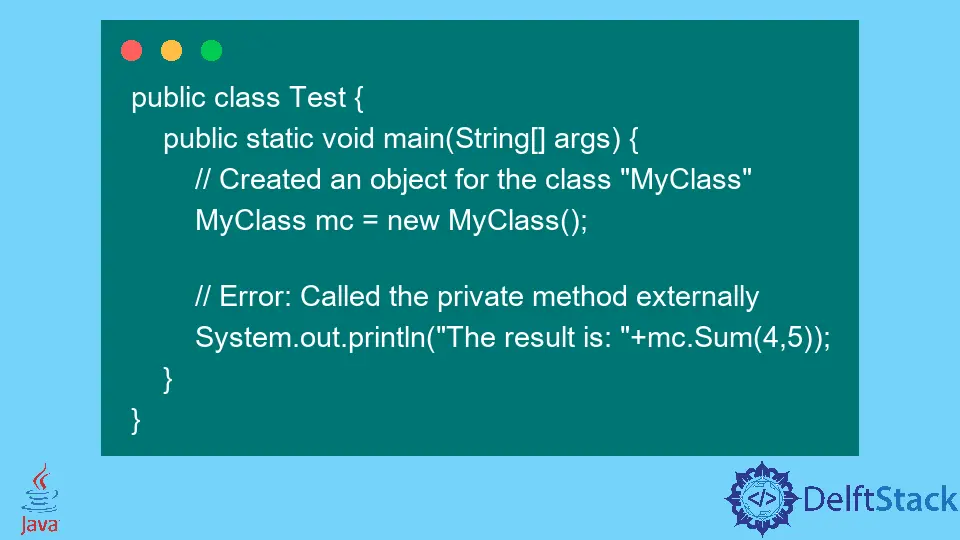
If you are working as a tester, you may perform many testing operations on the given code. But sometimes, performing a test operation is not accessible if you have a class containing a private
method.
A private
method is a method that is not accessible from the outside, which means you can’t call it externally. It is only available for the internal elements of the class.
It will only respond to the internal calling, but there is an idea through which you can test a private
method quickly. This article will show how we can test a private
method.
Test private
Method in Java
Suppose we have a class like the below that we need to test:
class MyClass {
private int Sum(int a, int b) { // method is private
return a + b;
}
}
As we can see, the above code has a private
method named Sum()
. Let’s see what happens if we try to test the method with another class called Test
.
public class Test {
public static void main(String[] args) {
// Created an object for the class "MyClass"
MyClass mc = new MyClass();
// Error: Called the private method externally
System.out.println("The result is: " + mc.Sum(4, 5));
}
}
It will return you with the below error:
error: Sum(int,int) has private access in MyClass
Because the Sum()
is a private
method in class MyClass
, we can not access it externally. So now, the only way to check it is to include a public
method inside the class MyClass
that will call the method Sum()
.
The public
method will be like the below:
public int NewSum(int a, int b) {
// A public method that can call the private method internally.
return Sum(a, b);
}
The above method will call the Sum()
method as it is inside the class MyClass
. Now the full version of the class MyClass
will look like this:
class MyClass {
private int Sum(int a, int b) { // Private method
return a + b;
}
public int NewSum(int a, int b) { // Public method
return Sum(a, b);
}
}
After updating the MyClass
, you can now test the private
method Sum()
. But you need to call the public
method NewSum()
from the object of MyClass
.
Now our updated test class will look like the below:
class Test {
public static void main(String[] args) {
// Created an object for the class "MyClass"
MyClass mc = new MyClass();
// Called the public method "NewSum()"
System.out.println("The result is: " + mc.NewSum(4, 5));
}
}
In the above code fence, you can see that we have called the class NewSum()
from the object MyClass
class. If the method Sum()
does not have any error or bug, it will show you the below output:
The result is: 9
Above, we have shared the most basic way to test a private
method and see if it works fine.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn