Private Methods in Java
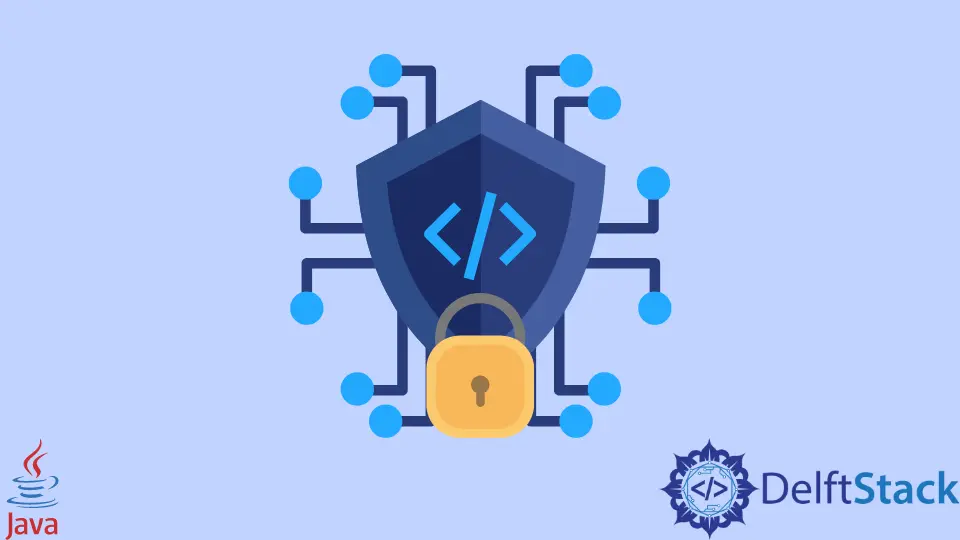
Private methods in Java have a private access modifier which means they have limited access to the defining class and are not accessible in the child class in inheritance; that is why they are not eligible for overriding.
However, a method can be created in the child class and could be accessed in the parent class. This tutorial demonstrates how to create and use private methods in Java.
Private Methods in Java
As mentioned above, private methods are only accessible in the defining class; we consider the points below for the private methods.
- Private methods are Class X are only accessible in Class X.
- Package-private members or methods of package X are only accessible within all the classes of the X package.
Let’s try to create and use private methods in Java. See example:
package delftstack;
public class Private_Methods {
private void print() {
System.out.println("The Private Method can only be printed in the defining Class");
}
public static void main(String[] args) {
Private_Methods Demo = new Private_Methods();
Demo.print();
Private_Methods_Child Demo1 = new Private_Methods_Child();
Demo1.print();
}
}
class Private_Methods_Child extends Private_Methods {
public void print() {
System.out.println("The Public Method can be printed anywhere");
}
}
The code above creates a private method and calls it in the same class and also a public method to call it in the parent class; the output will be:
The Private Method can only be printed in the defining Class
The Public Method can be printed anywhere
If we change the public method to private in the child class, it will throw an exception. See example:
package delftstack;
public class Private_Methods {
private void print() {
System.out.println("The Private Method can only be printed in the defining Class");
}
public static void main(String[] args) {
Private_Methods Demo = new Private_Methods();
Demo.print();
Private_Methods_Child Demo1 = new Private_Methods_Child();
Demo1.print();
}
}
class Private_Methods_Child extends Private_Methods {
private void print() {
System.out.println("The Public Method can be printed anywhere");
}
}
We cannot access the print
method from the child class. See output:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The method print() from the type Private_Methods_Child is not visible
at delftstack.Private_Methods.main(Private_Methods.java:11)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook