Java의 개인 메서드 테스트
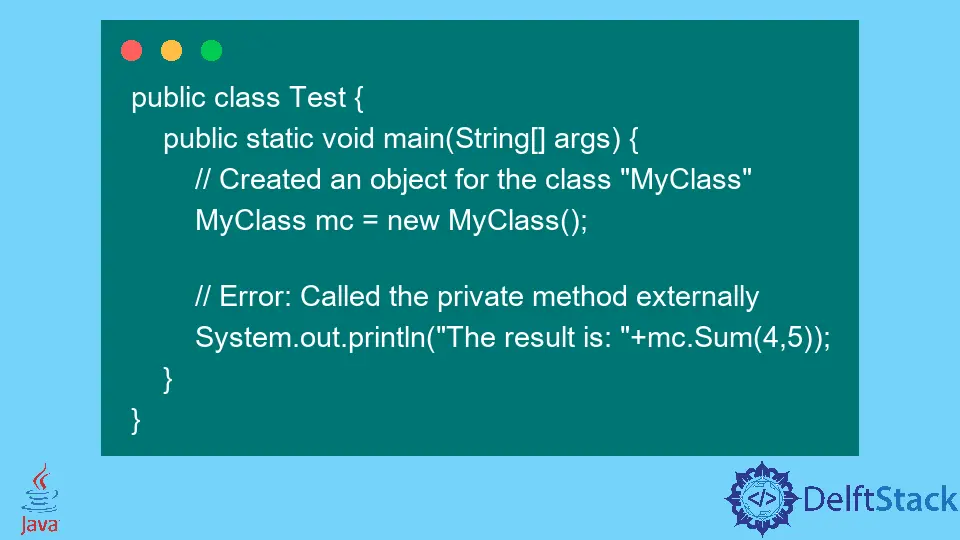
테스터로 작업하는 경우 주어진 코드에 대해 많은 테스트 작업을 수행할 수 있습니다. 그러나 때때로 private
메소드를 포함하는 클래스가 있는 경우 테스트 작업 수행에 액세스할 수 없습니다.
private
메서드는 외부에서 액세스할 수 없는 메서드이므로 외부에서 호출할 수 없습니다. 클래스의 내부 요소에만 사용할 수 있습니다.
내부 호출에만 응답하지만 private
메서드를 빠르게 테스트할 수 있는 아이디어가 있습니다. 이 기사에서는 개인
메소드를 테스트하는 방법을 보여줍니다.
Java에서 private
메서드 테스트
테스트해야 하는 아래와 같은 클래스가 있다고 가정합니다.
class MyClass {
private int Sum(int a, int b) { // method is private
return a + b;
}
}
보시다시피 위의 코드에는 Sum()
이라는 private
메서드가 있습니다. Test
라는 다른 클래스로 메서드를 테스트하려고 하면 어떤 일이 발생하는지 봅시다.
public class Test {
public static void main(String[] args) {
// Created an object for the class "MyClass"
MyClass mc = new MyClass();
// Error: Called the private method externally
System.out.println("The result is: " + mc.Sum(4, 5));
}
}
아래와 같은 오류가 반환됩니다.
error: Sum(int,int) has private access in MyClass
Sum()
은 MyClass
클래스의 비공개
메서드이므로 외부에서 액세스할 수 없습니다. 이제 이를 확인하는 유일한 방법은 Sum()
메서드를 호출하는 MyClass
클래스 내부에 public
메서드를 포함하는 것입니다.
public
메서드는 다음과 같습니다.
public int NewSum(int a, int b) {
// A public method that can call the private method internally.
return Sum(a, b);
}
위 메소드는 MyClass
클래스 내부에 있는 Sum()
메소드를 호출합니다. 이제 MyClass
클래스의 정식 버전은 다음과 같습니다.
class MyClass {
private int Sum(int a, int b) { // Private method
return a + b;
}
public int NewSum(int a, int b) { // Public method
return Sum(a, b);
}
}
MyClass
를 업데이트한 후 이제 private
메서드인 Sum()
을 테스트할 수 있습니다. 그러나 MyClass
객체에서 public
메서드 NewSum()
을 호출해야 합니다.
이제 업데이트된 테스트 클래스는 아래와 같습니다.
class Test {
public static void main(String[] args) {
// Created an object for the class "MyClass"
MyClass mc = new MyClass();
// Called the public method "NewSum()"
System.out.println("The result is: " + mc.NewSum(4, 5));
}
}
위의 코드 펜스에서 MyClass
클래스 개체에서 NewSum()
클래스를 호출한 것을 볼 수 있습니다. Sum()
메서드에 오류나 버그가 없으면 다음과 같은 결과가 표시됩니다.
The result is: 9
위에서 private
메서드를 테스트하고 제대로 작동하는지 확인하는 가장 기본적인 방법을 공유했습니다.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn