Java でプライベート メソッドをテストする
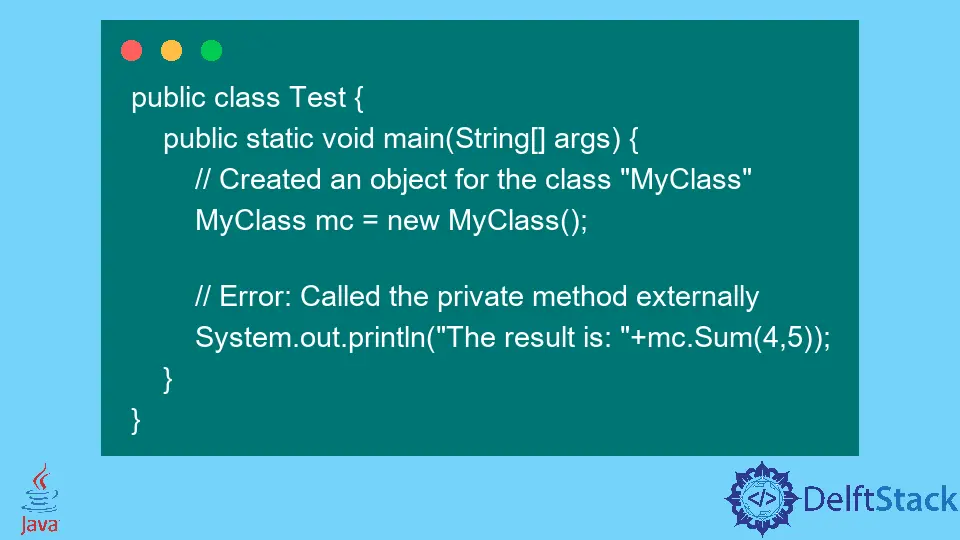
テスターとして作業している場合は、指定されたコードに対して多くのテスト操作を実行できます。 ただし、private
メソッド を含むクラスがある場合、テスト操作を実行できない場合があります。
private
メソッドは、外部からアクセスできないメソッドです。つまり、外部から呼び出すことはできません。 クラスの内部要素でのみ使用できます。
内部呼び出しにのみ応答しますが、private
メソッドをすばやくテストできるアイデアがあります。 この記事では、private
メソッドをテストする方法を示します。
Java で private
メソッドをテストする
テストする必要がある以下のようなクラスがあるとします。
class MyClass {
private int Sum(int a, int b) { // method is private
return a + b;
}
}
ご覧のとおり、上記のコードには Sum()
という名前の private
メソッドがあります。 Test
という別のクラスでメソッドをテストしようとするとどうなるか見てみましょう。
public class Test {
public static void main(String[] args) {
// Created an object for the class "MyClass"
MyClass mc = new MyClass();
// Error: Called the private method externally
System.out.println("The result is: " + mc.Sum(4, 5));
}
}
以下のエラーが返されます。
error: Sum(int,int) has private access in MyClass
Sum()
は MyClass
クラスの private
メソッドであるため、外部からアクセスすることはできません。 したがって、これをチェックする唯一の方法は、メソッド Sum()
を呼び出すクラス MyClass
内に public
メソッドを含めることです。
public
メソッドは次のようになります。
public int NewSum(int a, int b) {
// A public method that can call the private method internally.
return Sum(a, b);
}
上記のメソッドは、クラス MyClass
内にあるため、Sum()
メソッドを呼び出します。 クラスMyClass
のフルバージョンは次のようになります。
class MyClass {
private int Sum(int a, int b) { // Private method
return a + b;
}
public int NewSum(int a, int b) { // Public method
return Sum(a, b);
}
}
MyClass
を更新した後、private
メソッド Sum()
をテストできるようになりました。 ただし、MyClass
のオブジェクトから public
メソッド NewSum()
を呼び出す必要があります。
これで、更新されたテスト クラスは次のようになります。
class Test {
public static void main(String[] args) {
// Created an object for the class "MyClass"
MyClass mc = new MyClass();
// Called the public method "NewSum()"
System.out.println("The result is: " + mc.NewSum(4, 5));
}
}
上記のコード フェンスでは、オブジェクト MyClass
クラスからクラス NewSum()
を呼び出していることがわかります。 メソッド Sum()
にエラーやバグがない場合、以下の出力が表示されます。
The result is: 9
上記では、private
メソッドをテストし、それが正常に機能するかどうかを確認する最も基本的な方法を共有しました。
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn