The Difference Between Static and Dynamic Binding in Java
- Static Binding in Java
- Example of Static Binding in Java
- Dynamic Binding in Java
- Example of Dynamic Binding in Java
- Static Binding and Dynamic Binding in Java
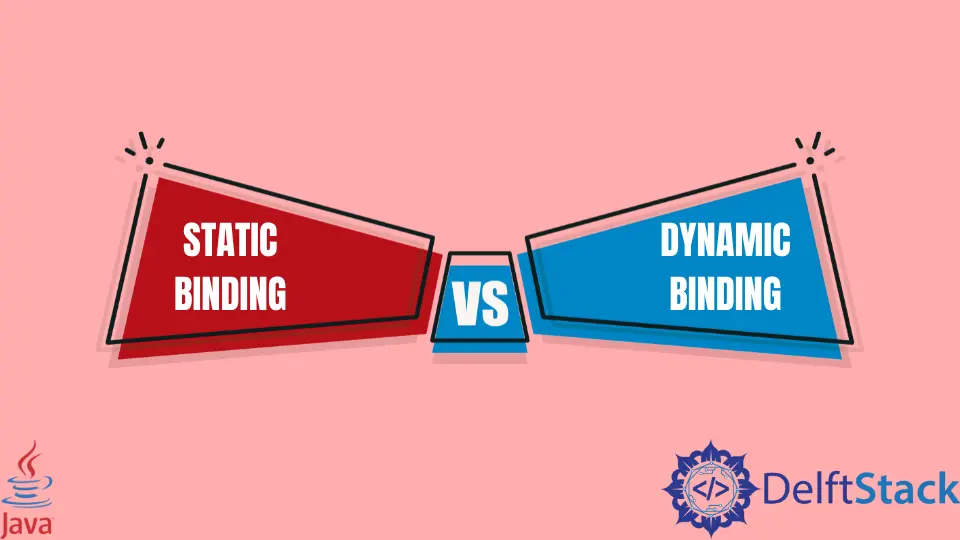
This tutorial introduces the difference between static binding and dynamic binding in Java and lists some example codes to guide you on the topic.
Binding is linking a method call to the method implementation, and it has two types: static and dynamic. According to polymorphism, an object can have many forms, and these forms can be resolved at either compile-time or runtime.
Static Binding happens when the method call is linked to method implementation at compile-time. Dynamic Binding occurs when the binding takes place during runtime. Let’s learn more about these two types of binding and look into some examples.
Static Binding in Java
As discussed above, Static Binding is the binding that occurs during compile time. It is also referred to as Early Binding because it occurs during the compile-time itself.
In the case of Static Binding, the compiler knows exactly which implementation of the method to use. It uses the type or class information for binding; method overloading is an example of Static Binding.
Note that all static, final, and private methods use Static Binding because child classes cannot override static, final, and private methods. The compiler knows at compile-time which method implementation to use. Since the compiler knows that the methods of the parent class cannot be overridden, it always uses the parent class implementation of the method.
Additionally, Static Binding also performs better as less overhead is involved.
Example of Static Binding in Java
In the code below, the school class has a static ringBell()
method, and this class is extended by Classroom
. The Classroom
also has a ringBell()
method.
Since the ringBell()
method is static, the compiler just checks the class type and does not consider the object class assigned. So when a School
class is used to create an object of Classroom(School s2 = new Classroom())
, the implementation of the School
class is considered and not the Classroom
class.
class School {
public static void ringBell() {
System.out.println("Ringing the school bell...");
}
}
class Classroom extends School {
public static void ringBell() {
System.out.println("Ringing the classroom bell...");
}
}
public class Main {
public static void main(String[] args) {
School s1 = new School(); // Type is School
s1.ringBell();
Classroom c1 = new Classroom(); // Type is Classroom
c1.ringBell();
School s2 = new Classroom(); // Type is School
s2.ringBell();
}
}
Output:
Ringing the school bell...
Ringing the classroom bell...
Ringing the school bell...
Dynamic Binding in Java
Dynamic Binding resolves the binding at runtime because the compiler does not know which implementation to use at compile-time.
It is also known as Late Binding because the binding is resolved at a later stage during runtime and not during compile-time. Dynamic Binding also uses the object for binding and not the type or class; method overriding is an example of Dynamic Binding.
Example of Dynamic Binding in Java
In the code below, the ringBell()
method is not static, so overriding will occur. The compiler does not know which implementation of the ringBell()
method should be used, so binding is resolved at runtime.
At runtime, the object is considered and not the type which was used to create it. So when a School
class is used to create an object of Classroom
, then the implementation of the Classroom
class is considered and not the School
class.
class School {
public void ringBell() {
System.out.println("Ringing the school bell...");
}
}
class Classroom extends School {
@Override
public void ringBell() {
System.out.println("Ringing the classroom bell...");
}
}
public class Main {
public static void main(String[] args) {
School s1 = new School(); // Type is School and object is of School
s1.ringBell();
Classroom c1 = new Classroom(); // Type is Classroom and object is of Classroom
c1.ringBell();
School s2 = new Classroom(); // Type is School and object is of Classroom
s2.ringBell();
}
}
Output:
Ringing the school bell...
Ringing the classroom bell...
Ringing the classroom bell...
Static Binding and Dynamic Binding in Java
Static Binding is the connection of the method call to the method implementation at compile-time. On the other hand, Dynamic Binding is the connection of the method call to the method implementation at runtime.
Knowing both of these techniques is important to understand the concept of polymorphism. Static Binding is used by static, final, and private methods; this binding comes into action in the case of overloaded methods. Dynamic Binding is used for virtual methods (by default virtual in Java) and used to bind overridden methods.