The sqrt() Method in Java
-
Use the
sqrt()
Method to Find the Square Root of a Number in Java -
Find the Square Root of a Number Without Using
sqrt()
Method in Java
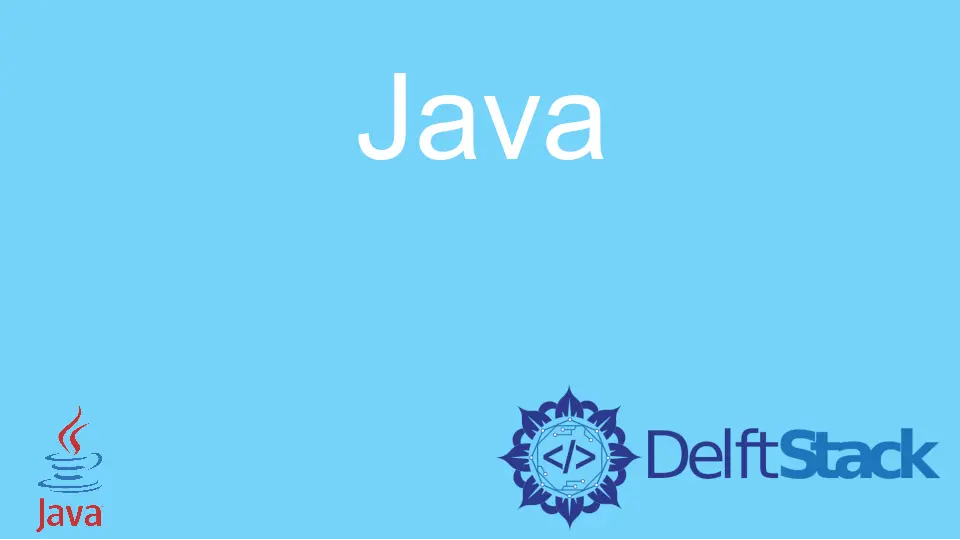
This tutorial demonstrates the sqrt()
method in Java with various code examples. It also educates about the Math.pow()
method and a custom formula that we can use to find a square root of a number without using the sqrt()
method.
Use the sqrt()
Method to Find the Square Root of a Number in Java
The java.lang.Math
package contains the sqrt()
method. It returns the square root of a number whose type is double
and passed to the sqrt()
method as an argument.
If the passed argument is either NaN
or a negative number, it returns NaN
.
The sqrt()
function outputs positive infinity if we pass the positive infinity as an argument. And, if the sqrt()
method gets negative or positive zero, the results would be the same as the arguments.
Let’s explore all of the scenarios by using various example codes.
Example Code (if the passed argument is a positive number of type double):
import java.lang.Math;
public class calSquareRoot {
public static void main(String args[]) {
double number = 25;
double sqrt = Math.sqrt(number);
System.out.println("The square root of " + number + " is " + sqrt);
}
}
Output:
The square root of 25.0 is 5.0
This code example shows that we have to import
the java.lang.Math
package to use the sqrt()
method in the Java program. Inside the main
method, we declare and initialize a variable number
of type double with a value of 25
.
Then, we pass this number
to the sqrt()
method and store the returned value in the sqrt
variable, which is further used in System.out.println
to print a specified number
’s square root.
The procedure will remain the same for all the upcoming examples in this section, but we will update the value of the number
to experiment with different situations.
Example Code (if the passed argument is NaN
):
import java.lang.Math;
public class calSquareRoot {
public static void main(String args[]) {
double number = Double.NaN;
double sqrt = Math.sqrt(number);
System.out.println("The square root of " + number + " is " + sqrt);
}
}
Output:
The square root of NaN is NaN
Example Code (if the passed argument is a negative number):
import java.lang.Math;
public class calSquareRoot {
public static void main(String args[]) {
double number = -5;
double sqrt = Math.sqrt(number);
System.out.println("The square root of " + number + " is " + sqrt);
}
}
Output:
The square root of -5.0 is NaN
Example Code (if the passed argument is a positive infinity):
import java.lang.Math;
public class calSquareRoot {
public static void main(String args[]) {
double number = Double.POSITIVE_INFINITY;
double sqrt = Math.sqrt(number);
System.out.println("The square root of " + number + " is " + sqrt);
}
}
Output:
The square root of Infinity is Infinity
Example Code (if the passed argument is negative or positive zero):
import java.lang.Math;
public class calSquareRoot {
public static void main(String args[]) {
double number = -0;
double sqrt = Math.sqrt(number);
System.out.println("The square root of " + number + " is " + sqrt);
number = 0;
sqrt = Math.sqrt(number);
System.out.println("The square root of " + number + " is " + sqrt);
}
}
Output:
The square root of 0.0 is 0.0
The square root of 0.0 is 0.0
We have covered all the possible examples discussed at the beginning of this section. It’s time to explore other methods to find the square root without using the sqrt()
method.
Find the Square Root of a Number Without Using sqrt()
Method in Java
For this section, we will cover the Math.pow()
method and a custom formula below.
We’ll make sure that both approaches fulfill the sqrt()
method’s rules that are given below.
- Returns the square root of a positive number.
- Returns
NaN
ifNaN
or a negative number is passed. - Returns the same passed number if negative zero or positive zero.
- Returns
Infinity
if it getsDouble.POSITIVE_INFINITY
.
Use the Math.pow()
Method to Find the Square Root of a Number in Java
Example Code:
public class calSquareRoot {
public static void main(String[] args) {
double positiveNumber = 25;
double negativeNumber = -25;
double nan = Double.NaN;
double postiveInfinity = Double.POSITIVE_INFINITY;
double positiveZero = 0;
double negativeZero = -0;
double sqrt = 0;
sqrt = Math.pow(positiveNumber, 0.5);
System.out.println("The Square root of " + positiveNumber + " = " + sqrt);
sqrt = Math.pow(nan, 0.5);
System.out.println("The Square root of " + nan + " = " + sqrt);
sqrt = Math.pow(negativeNumber, 0.5);
System.out.println("The Square root of " + negativeNumber + " = " + sqrt);
sqrt = Math.pow(postiveInfinity, 0.5);
System.out.println("The Square root of " + postiveInfinity + " = " + sqrt);
sqrt = Math.pow(positiveZero, 0.5);
System.out.println("The Square root of " + positiveZero + " = " + sqrt);
sqrt = Math.pow(negativeZero, 0.5);
System.out.println("The Square root of " + negativeZero + " = " + sqrt);
}
}
Output:
The Square root of 25.0 = 5.0
The Square root of NaN = NaN
The Square root of -25.0 = NaN
The Square root of Infinity = Infinity
The Square root of 0.0 = 0.0
The Square root of 0.0 = 0.0
As we know, the √number = number½
is mathematically approved. So, we can use the power function (Math.pow()
) to calculate the power, which is the value of the first parameter raised to 0.5 here.
And it is a square root of the given number.
Use Custom Formula Method to Find the Square Root of a Number in Java
Example Code:
public class calSquareRoot {
public static double squareRoot(double number) {
if (number < 0 || Double.isNaN(number))
return Double.NaN;
else if (number == Double.POSITIVE_INFINITY)
return Double.POSITIVE_INFINITY;
else if (number == 0 || number == -0)
return number;
else if (number > 0) {
double temp;
double sqrt = number / 2;
do {
temp = sqrt;
sqrt = (temp + (number / temp)) / 2;
} while ((temp - sqrt) != 0);
return sqrt;
}
else {
return -1;
}
}
public static void main(String[] args) {
double positiveNumber = 25;
double negativeNumber = -25;
double nan = Double.NaN;
double postiveInfinity = Double.POSITIVE_INFINITY;
double positiveZero = 0;
double negativeZero = -0;
double sqrt = 0;
sqrt = squareRoot(positiveNumber);
System.out.println("The Square root of " + positiveNumber + " = " + sqrt);
sqrt = squareRoot(nan);
System.out.println("The Square root of " + nan + " = " + sqrt);
sqrt = squareRoot(negativeNumber);
System.out.println("The Square root of " + negativeNumber + " = " + sqrt);
sqrt = squareRoot(postiveInfinity);
System.out.println("The Square root of " + postiveInfinity + " = " + sqrt);
sqrt = squareRoot(positiveZero);
System.out.println("The Square root of " + positiveZero + " = " + sqrt);
sqrt = squareRoot(negativeZero);
System.out.println("The Square root of " + negativeZero + " = " + sqrt);
}
}
Output:
The Square root of 25.0 = 5.0
The Square root of NaN = NaN
The Square root of -25.0 = NaN
The Square root of Infinity = Infinity
The Square root of 0.0 = 0.0
The Square root of 0.0 = 0.0
Here, we are using the formula below.
Inside the main
function, we have different values that are passed to squareRoot()
method where we have if-else
conditions to check the rules.
We return the values depending on the given rules for finding the square root of a number and implement the given formula if the given number is greater than 0. Remember, the first square root number must be an inputNumber/2
.