How to Return Multiple Values in Java
- Return Multiple Values of the Same Type Using Array in Java
- Return Multiple Values Using a Custom Class in Java
- Return Multiple Values Using a List in Java
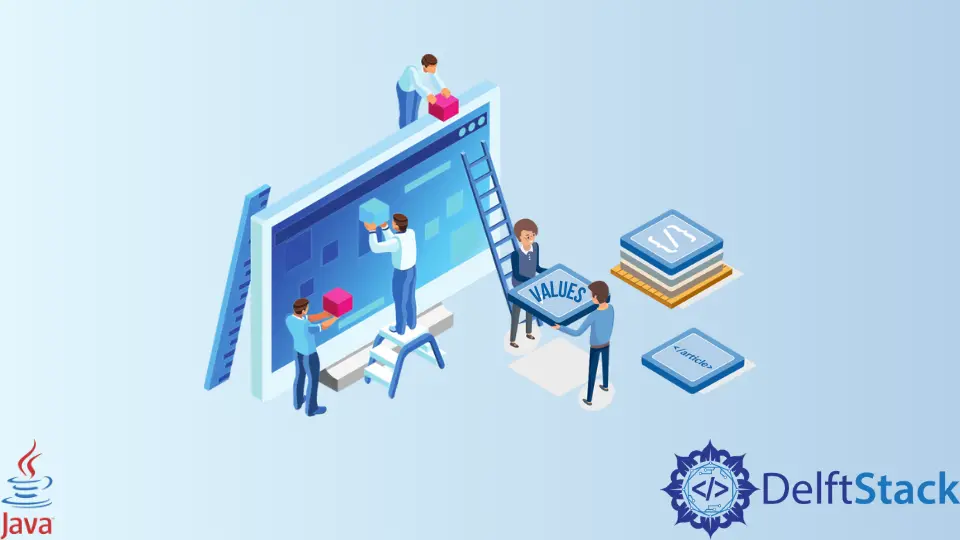
In this tutorial, we will learn to return multiple values in Java. We cannot return more than one value from a method directly in Java, but we can use other features of Java, like Array
, Pair
, List
etc. to achieve that.
Return Multiple Values of the Same Type Using Array in Java
We can return more than one values that are of the same data type using an array. As array stores multiple values so we can return an array from a method as in our example. Below, we create a method method1()
that has a return type of String[]
array of Strings. In method1()
, we create three local variables and assign them with values, now we create an array of type String
called array1
.
Now we set the indexes of array1
with the variables. Then we return the array using return array1
. In the main()
method, we call method1()
and convert it to a String using Arrays.toString()
and we can see the array of all the values in the output.
import java.util.Arrays;
public class MultipleObjects {
public static void main(String[] args) {
String getArray = Arrays.toString(method1());
System.out.println("Array values: " + getArray);
}
private static String[] method1() {
String name = "John Doe";
String city = "New York";
String gender = "male";
String[] array1 = new String[3];
array1[0] = name;
array1[1] = city;
array1[2] = gender;
return array1;
}
}
Output:
Array values: [John Doe, New York, male]
Return Multiple Values Using a Custom Class in Java
In this example, we create a custom class, ExampleClass
, with three different types of variables. In the ExampleClass
constructor, we get the parameters and initialize all the variables with values. We create a method method1()
that returns an instance of ExampleClass
. In method1()
we call the constructor of ExampleClass
and pass values in it. Inside the main()
function we call the method1()
method that returns the object of ExampleClass
.
Now we get the values using the object getValues
. Notice that we can use values of different types.
public class MultipleObjects {
public static void main(String[] args) {
ExampleClass getValues = method1();
System.out.println("Value1: " + getValues.var1);
System.out.println("Value2: " + getValues.var2);
System.out.println("Value3: " + getValues.var3);
}
private static ExampleClass method1() {
return new ExampleClass(20, "ExampleString", true);
}
static class ExampleClass {
int var1;
String var2;
boolean var3;
ExampleClass(int var1, String var2, boolean var3) {
this.var1 = var1;
this.var2 = var2;
this.var3 = var3;
}
}
}
Output:
Value1: 20
Value2: ExampleString
Value3: true
Return Multiple Values Using a List in Java
We make a List
of all the values that we want to return in this program. In method1()
, we create three variables of different data types and then call Arrays.asList()
to create a List
and pass all the variables in it that will make a list of Object
. In main()
, we call method1()
and get the list of objects and print it in the console.
import java.util.Arrays;
import java.util.List;
public class MultipleObjects {
public static void main(String[] args) {
List<Object> getList = method1();
System.out.println("List of values: " + getList);
}
private static List<Object> method1() {
int var1 = 15;
String var2 = "ExampleString";
boolean var3 = false;
return Arrays.asList(var1, var2, var3);
}
}
Output:
List of values: [15, ExampleString, false]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn