Private Static Variable in Java
- Understanding Private Static Variables
- Practical Example of Private Static Variables
- Benefits of Using Private Static Variables
- Conclusion
- FAQ
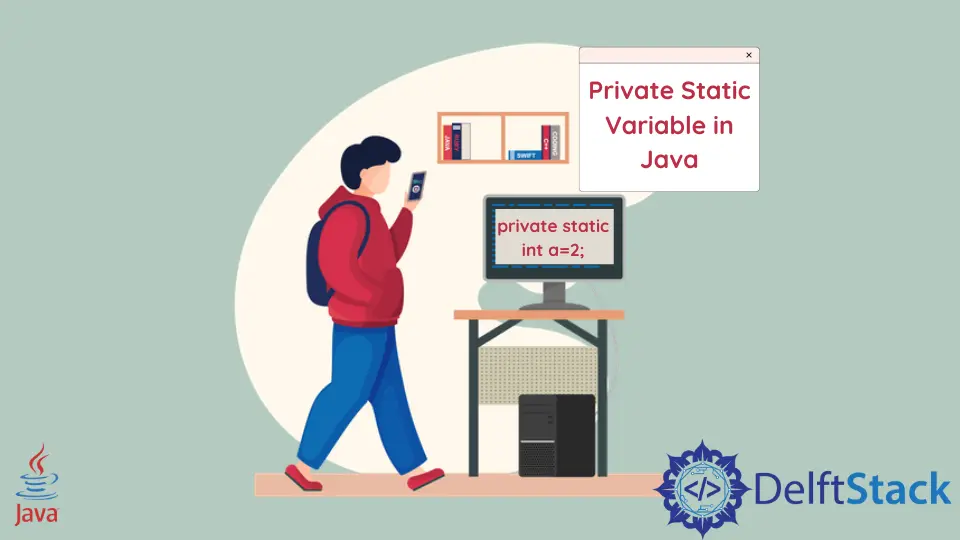
In the world of Java programming, understanding the concept of private static variables is essential for writing efficient and maintainable code. A private static variable belongs to the class rather than any instance of the class, which means that it retains its value across all instances. This feature can be particularly useful when you want to maintain a shared state or count across multiple objects without exposing the variable to the outside world.
In this tutorial, we will dive deep into what private static variables are, how they work, and provide practical examples to illustrate their use. By the end of this article, you will have a solid grasp of private static variables in Java, enabling you to leverage this powerful feature in your own applications.
Understanding Private Static Variables
Private static variables in Java are declared using the private
and static
keywords. The private
keyword restricts access to the variable, ensuring that it cannot be accessed directly from outside the class. On the other hand, the static
keyword means that the variable belongs to the class itself rather than to any individual object. This unique combination allows for shared data across instances while keeping it encapsulated within the class.
Declaring a Private Static Variable
To declare a private static variable, you use the following syntax:
class MyClass {
private static int myStaticVar = 0;
}
In this example, myStaticVar
is a private static variable. It can only be accessed through methods within MyClass
, ensuring that its value can be controlled and modified as needed.
Accessing a Private Static Variable
Accessing a private static variable typically involves creating static methods within the class. Here’s how you can do that:
class MyClass {
private static int myStaticVar = 0;
public static void increment() {
myStaticVar++;
}
public static int getValue() {
return myStaticVar;
}
}
In this code, increment()
is a static method that increases the value of myStaticVar
, while getValue()
retrieves its current value. Since both methods are static, they can be called without creating an instance of MyClass
.
Practical Example of Private Static Variables
Let’s explore a practical scenario where private static variables can be beneficial. Suppose you are developing a simple application to track the number of instances of a class created.
class InstanceCounter {
private static int instanceCount = 0;
public InstanceCounter() {
instanceCount++;
}
public static int getInstanceCount() {
return instanceCount;
}
}
Here, every time a new instance of InstanceCounter
is created, the constructor increments the instanceCount
variable. You can retrieve the total number of instances created using the getInstanceCount()
method.
Testing the InstanceCounter Class
To see this in action, you might write the following code in your main method:
public class Main {
public static void main(String[] args) {
new InstanceCounter();
new InstanceCounter();
new InstanceCounter();
System.out.println("Total instances created: " + InstanceCounter.getInstanceCount());
}
}
In this example, three instances of InstanceCounter
are created. The output will show the total number of instances created.
Output:
Total instances created: 3
The instanceCount
variable remains consistent across all instances, demonstrating the utility of private static variables in managing shared data.
Benefits of Using Private Static Variables
Using private static variables comes with several advantages:
-
Data Encapsulation: Since the variable is private, it cannot be accessed directly from outside the class, promoting better encapsulation and data hiding.
-
Shared State: Static variables are shared among all instances of the class, making them ideal for maintaining a shared state or count.
-
Memory Efficiency: A single copy of the static variable is created, which can lead to better memory management, especially when dealing with a large number of instances.
-
Ease of Access: Static methods can be called without creating an instance of the class, allowing for easy access to the shared variable.
Conclusion
In conclusion, private static variables in Java are a powerful feature that allows developers to manage shared data effectively while ensuring encapsulation. By understanding how to declare, access, and utilize these variables, you can enhance your Java applications significantly. Whether you’re tracking instance counts or maintaining shared states, private static variables can help streamline your code and improve its efficiency. Embrace this concept and watch your Java programming skills soar to new heights!
FAQ
-
What is a private static variable in Java?
A private static variable is a class-level variable that is only accessible within the class, allowing for shared data across all instances. -
How do you access a private static variable?
You can access a private static variable through static methods defined within the class. -
Why use private static variables?
They provide encapsulation, shared state across instances, memory efficiency, and easy access without needing to create an instance. -
Can a private static variable be modified?
Yes, a private static variable can be modified through static methods within the class. -
Are private static variables thread-safe?
Private static variables are not inherently thread-safe. You may need to implement synchronization mechanisms to ensure thread safety in a multi-threaded environment.