How to Fix Error: No Such Element Exception While Using Scanner in Java
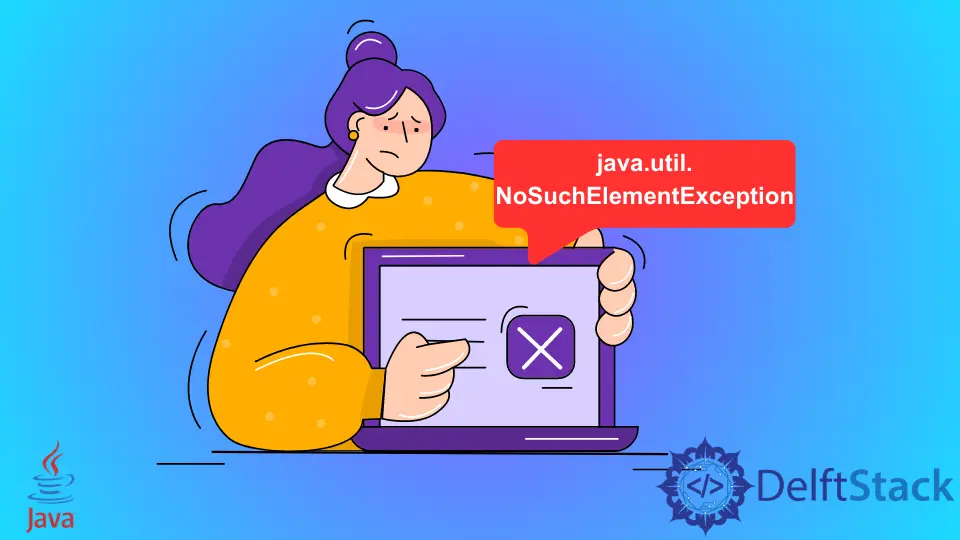
This tutorial will demonstrate solving the NoSuchElementException
error while using the Scanner in Java.
No Such Element Exception While Using Scanner in Java
The Scanner class is used to get the user input in a Java program. It uses several utility methods like next()
, nextInt()
, etc.
When working with these methods, the Scanner can throw a NoSuchElementException
error in Java. The reason for these exceptions are given below:
-
Suppose we have two Scanner objects for getting user input; if we close one Scanner and get the input from the other one, it will throw the
NoSuchElementExcpetion
. This is because when we close one Scanner, it will close the Input Stream; that is why the other Scanner cannot read from the same Input Stream because theclose()
method also closes theSystem.in
input stream.Here is an example:
package delftstack; import java.util.*; public class Example { public static void main(String args[]) { String DemoString = "Hello, This is delftstack.com"; Scanner DemoScanner1 = new Scanner(System.in); Scanner DemoScanner2 = new Scanner(System.in); DemoScanner1.close(); DemoScanner2.next(); } }
The code above will throw the
NoSuchElementException
. See output:
```text
Exception in thread "main" java.util.NoSuchElementException
at java.base/java.util.Scanner.throwFor(Scanner.java:937)
at java.base/java.util.Scanner.next(Scanner.java:1478)
at delftstack.Example.main(Example.java:13)
```
To solve this issue, we can use just one Scanner. This also applies if one Scanner is used in one method and the other in another method.
The `close()` method will close all the input streams.
-
While using Scanner, we are reading the line, and if there is no line left to read, it will throw the
NoSuchElementException
. See example:package delftstack; import java.util.*; public class Example { public static void main(String args[]) { String DemoString = "Hello, This is delftstack.com"; Scanner DemoScanner1 = new Scanner(DemoString); System.out.println(DemoScanner1.nextLine()); System.out.println(DemoScanner1.nextLine()); } }
<!--
test:
command: java
expected_output: 'Hello, This is delftstack.com
'
-->
<!--adsense-->
To solve the issue, we use the `hasNextLine()` to check if the Scanner has the next line. It returns true if the Scanner has the next line; otherwise, it returns false.
See example:
```java
package delftstack;
import java.util.*;
public class Example {
public static void main(String args[]) {
String DemoString = "Hello, This is delftstack.com";
Scanner DemoScanner1 = new Scanner(DemoString);
while (DemoScanner1.hasNextLine()) {
System.out.println(DemoScanner1.nextLine());
}
}
}
```
<!--
test:
command: java
expected_output: 'Hello, This is delftstack.com
'
-->
The output for this code is:
```text
Hello, This is delftstack.com
```
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack