Method Overloading in Java
- Introduction to Method Overloading in Java
- Pros and Cons of Using Method Overloading in Java
- Use Method Overloading in Java
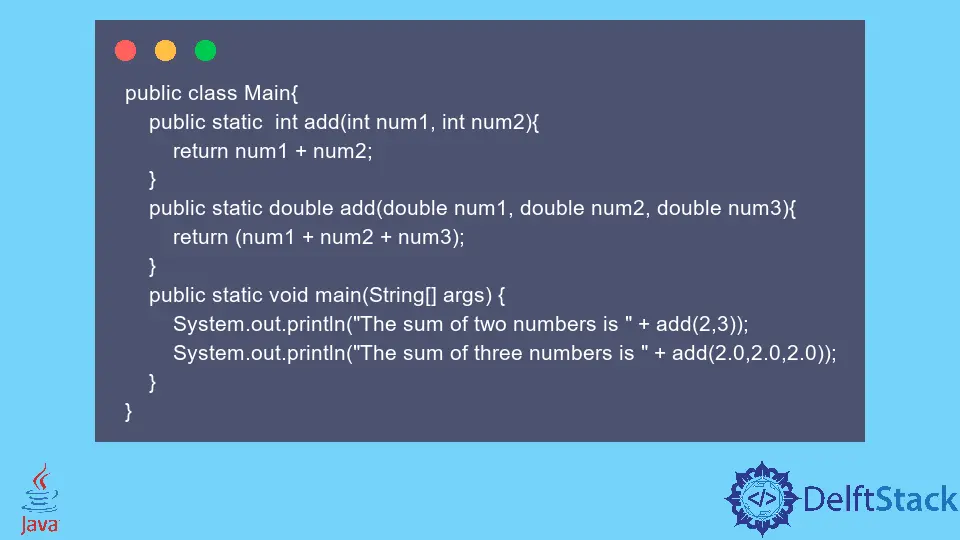
If you are learning Java programming, you may have heard about the method overloading. We will go through an introduction to method overloading in Java in this article.
Introduction to Method Overloading in Java
Various terms can be used as an alternative to method overloading. We can address method overloading as function overloading, static polymorphism, or compile-time polymorphism, so don’t be confused if you hear about any of them because all are the same.
Having many functions/methods with the same name but the different input parameters, types of input parameters, or both, is called method overloading/function overloading. These functions/methods are known as overloaded methods or overloaded functions.
Pros and Cons of Using Method Overloading in Java
The advantages of method overloading are listed below.
- It boosts the readability and cleanliness of the code.
- It gives the flexibility to call various methods having the same name but different parameters.
- We can also have various return types for each function having the same name as others.
The following are the disadvantages of method overloading.
- Understanding the technique of method overloading is a bit tricky for an absolute beginner.
- It requires more effort in designing and finalizing the number of parameters and their data types.
Use Method Overloading in Java
Suppose you have an application that calculates the area of different shapes, for instance, the area of a circle and the area of a triangle.
We know the number of parameters, their data types, and the formula of all the shapes. So, we can define the overloaded functions for this situation.
Here are the function definitions for calculating the area of the shapes that have the same function names but different numbers of input parameters:
double calcAreaOfShape(double radius); // area of a circle
double calcAreaOfShape(double base, double height); // area of a triangle
The second example is about adding different numbers. We can have single or multiple input parameters with different data types this time.
int add(int num1, int num2);
float add(float num1, float num2, float num3);
/*Remember, the return type can't differ from the data type of
input parameters of the same function.*/
int add(float num1, float num2); // it is invalid
The function overloading concept is also useful when we have a requirement like the function can be called depending on passing a sequence of data types in input parameters. See the following function definitions:
void display(String fullname, int age);
void display(int age, String fullname);
Let’s implement all of these scenarios one by one. Below is an example of using method overloading in Java.
Source Code:
public class Main {
public static void calcAreaOfShape(double radius) {
System.out.println("The Area of a Circle is " + (3.14 * radius * radius));
}
public static void calcAreaOfShape(double base, double height) {
System.out.println("The Area of a Triangle is " + (0.5 * base * height));
}
public static void main(String[] args) {
calcAreaOfShape(5.0);
calcAreaOfShape(3.0, 3.0);
}
}
Output:
The Area of a Circle is 78.5
The Area of a Triangle is 4.5
We call the calcAreaOfShape()
method twice inside the main function. First for the circle with one input parameter and second for calculating the triangle area by passing two input parameters.
We also want to calculate the area of a rectangle that takes two parameters (length
and width
). There are two ways to do that.
The first way is to pass the third parameter of the String
type that tells whether it is being called for rectangle or triangle. Remember, you have to update the function signature from two input parameters to three input parameters (see the following snippet).
Source Code:
public class Main {
public static void calcAreaOfShape(double radius) {
System.out.println("The Area of a Circle is " + (3.14 * radius * radius));
}
public static void calcAreaOfShape(double a, double b, String shapeName) {
if (shapeName == "triangle")
System.out.println("The Area of a Triangle is " + (0.5 * a * b));
else if (shapeName == "rectangle")
System.out.println("The Area of a Rectangle is " + (a * b));
else
System.out.println("Wrong Shape is Passed");
}
public static void main(String[] args) {
calcAreaOfShape(5.0);
calcAreaOfShape(3.0, 3.0, "triangle");
calcAreaOfShape(4.0, 2.0, "rectangle");
}
}
Output:
The Area of a Circle is 78.5
The Area of a Triangle is 4.5
The Area of a Rectangle is 8.0
The above code is working fine, but there are some issues. The first issue is that the variable names should be base
& height
when called for triangle while we need length
and width
for the rectangle.
We’ve changed the variable names to a
and b
to use them for both (rectangle and triangle), but we are losing code readability and understandability.
The second issue is to write multiple if-else
conditions to handle all the situations because the user can enter rectangle
, Rectangle
, or RECTANGLE
.
To resolve all these limitations and adopt a professional approach, we prefer function overriding for this kind of circumstances where we use the instanceOf
to check which object is called.
Source Code (Functions with a different number of input parameters and data types):
public class Main {
public static int add(int num1, int num2) {
return num1 + num2;
}
public static double add(double num1, double num2, double num3) {
return (num1 + num2 + num3);
}
public static void main(String[] args) {
System.out.println("The sum of two numbers is " + add(2, 3));
System.out.println("The sum of three numbers is " + add(2.0, 2.0, 2.0));
}
}
Output:
The sum of two numbers is 5
The sum of three numbers is 6.0
We use function overloading to handle many input parameters and various data types here.
Source Code (Functions with a sequence of data types of input parameters):
public class Main {
public static void display(String fullname, int age) {
System.out.println("I am " + fullname + ", I am " + age + " years old");
}
public static void display(int age, String fullname) {
System.out.println("I am " + age + ", how old are you, " + fullname + "?");
}
public static void main(String[] args) {
display("Thomas Christopher", 34);
display(45, "John");
}
}
Output:
I am Thomas Christopher, I am 34 years old
I am 45, how old are you, John?
In the above example, we are using function overloading to handle the order of input parameters.