Method Hiding in Java
- Implementation of the Method Hiding Concept in Java
- Differences Between Method Hiding and Overriding in Java
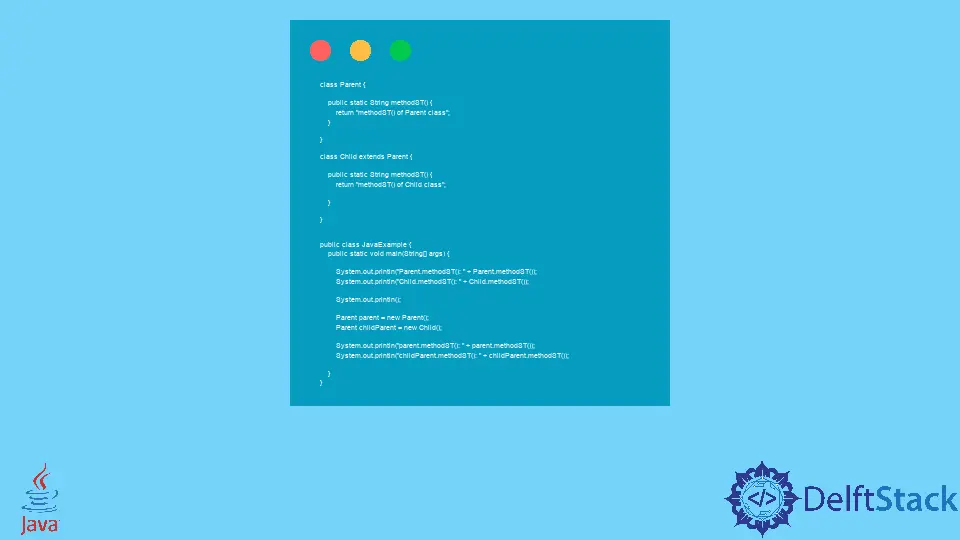
In this article, we will be looking at the concept of method hiding in Java. Method Hiding is similar to overriding, but it comes into play when using the same method signature for static functions in child and parent classes.
Implementation of the Method Hiding Concept in Java
In the following example, we take three classes, a Parent
and Child
class with the JavaExample
class with the main()
method. The Child
class inherits the Parent
class. Both the Parent
and Child
classes have two methods.
In the Parent
class, we have a method called methodST()
that is a static
function and returns a String. The Child
class also has a methodST()
with the same return type and is static; only the string is changed.
As both the methods are static, we can call it using the class’s names, and when we call the methodST()
from the Parent
class, its method is called, and the string is printed.
But when we call the methodST()
function of the Child
class, its method is executed instead of the Parent
class even if it inherits the Parent
class. It is called Method Hiding because the child hides the parent’s method.
Suppose we create two objects, one of the Parent
class and another of the Child
class. The Parent
method is only called because the reference is for Parent
, and the static methods are attached to references of classes instead of their objects.
class Parent {
public static String methodST() {
return "methodST() of Parent class";
}
}
class Child extends Parent {
public static String methodST() {
return "methodST() of Child class";
}
}
public class JavaExample {
public static void main(String[] args) {
System.out.println("Parent.methodST(): " + Parent.methodST());
System.out.println("Child.methodST(): " + Child.methodST());
System.out.println();
Parent parent = new Parent();
Parent childParent = new Child();
System.out.println("parent.methodST(): " + parent.methodST());
System.out.println("childParent.methodST(): " + childParent.methodST());
}
}
Output:
Parent.methodST(): methodST() of Parent class
Child.methodST(): methodST() of Child class
parent.methodST(): methodST() of Parent class
childParent.methodST(): methodST() of Parent class
Differences Between Method Hiding and Overriding in Java
We need static methods in both the parent and the child classes for method hiding while overriding works with non-static methods.
In overriding, the methods are called using the runtime object, while the compiler calls the static methods using the reference of a class in method hiding.
Method hiding is also known as Early Binding, while overriding is known as Late Binding.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn