How to Fix Error: Javac Cannot Find Symbol Error in Java
- Importance of Understanding and Resolving the Issue
-
Common Causes of
Javac Cannot Find Symbol
Errors -
Cannot Find Symbol
Errors -
Best Practices to Avoid
Cannot Find Symbol
Errors - Conclusion
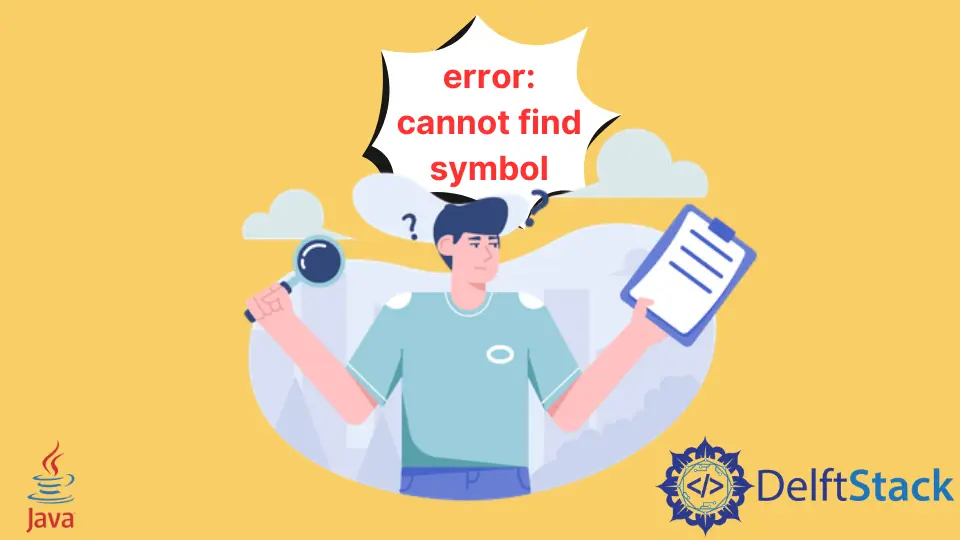
This article delves into common causes, solutions, and best practices for avoiding the javac cannot find symbol
error in Java, offering essential insights for robust and error-free code development.
Importance of Understanding and Resolving the Issue
Understanding and resolving javac cannot find symbol
is paramount in Java programming. This error signals a mismatch between code references and their definitions, potentially leading to compilation failures.
Rectifying it ensures code accuracy, preventing runtime issues. A solid grasp of this concept is fundamental for maintaining clean, functional code and fostering a smoother development process.
Common Causes of Javac Cannot Find Symbol
Errors
The cannot find symbol
error in Java typically occurs when the Java compiler (javac
) encounters a reference to a variable, method, or class that it cannot locate in the current scope or the imported packages. Here are some common reasons for this error and their corresponding solutions:
Sure, here’s the information presented in tabular format:
Cause | Solution | |
---|---|---|
1 | Typo or Misspelling | Double-check the spelling and ensure that the symbol is correctly named. |
2 | Incorrect Scope | Make sure the symbol is declared within the appropriate scope, either locally or globally. |
3 | Incorrect Import Statement | Add the correct import statement for the class or package containing the symbol. |
4 | Missing Compilation | Compile the source files containing the symbol using the javac command and ensure they are in the classpath . |
5 | Order of Compilation | Change the order of compilation so that the file containing the symbol is compiled before the referencing file. |
6 | Incorrect Package | Use the correct package name in the import statement or move the class containing the symbol to the appropriate package. |
7 | Access Modifier Issues | Check the access modifier of the symbol and ensure it allows visibility to the current context (e.g., use public or package-private as needed). |
8 | Interface Implementation | Implement the missing methods if the class is implementing an interface or extend the class properly. |
9 | Compilation Order in Maven or Gradle | Ensure that the dependencies and module structure are set up correctly in your build tool configuration. |
By addressing these common issues, you should be able to resolve the cannot find symbol
errors in your Java code.
Cannot Find Symbol
Errors
In the intricate world of Java programming, encountering the infamous javac cannot find symbol
error is not uncommon. This error, often accompanied by frustration, arises when the Java compiler (javac
) encounters a reference to a symbol that it cannot locate in the code.
Symbols can be variables, methods, or classes, and their absence or misplacement can lead to compilation failures.
Understanding and resolving this error is crucial for maintaining clean and functional Java code. In this section, we will delve into the common causes of the cannot find symbol
error and provide practical solutions through a comprehensive example.
import java.util.Date; // Add the import statement for Date
public class SymbolErrorExample {
public static void main(String[] args) {
// Scenario 1: Typo or Misspelling
String message = "Hello, Java!";
System.out.println(message); // Corrected variable name
// Scenario 2: Incorrect Scope
int number = generateNumber();
System.out.println(number);
// Scenario 3: Incorrect Import Statement
Date currentDate = new Date();
System.out.println(currentDate.toString());
}
// Scenario 2: Incorrect Scope - Method definition
private static int generateNumber() {
return 42;
}
}
Let’s dissect the code step by step, addressing each scenario and showcasing the solutions.
Scenario 1: Typo or Misspelling
In the first scenario, we encounter a common culprit: a typo in the variable name. The variable massage
is corrected to message
. Such typographical errors are a frequent cause of the cannot find symbol
error.
Scenario 2: Incorrect Scope
Moving on to the second scenario, we explore incorrect scope issues. The generateNumber
method is called within the main
method, demonstrating proper scoping.
The generateNumber
method, though defined below main
, is accessible due to its global scope within the class.
Scenario 3: Incorrect Import Statement
The third scenario deals with incorrect import statements. Here, we correctly import the Date
class from the java.util
package. Failing to import required classes results in the cannot find symbol
error.
By addressing these scenarios, we ensure that our Java code is free from the clutches of the cannot find symbol
error. Now, let’s run the code and witness the seamless execution.
Output:
Hello, Java!
42
Fri Jan 12 13:41:49 SGT 2024
This output reaffirms the effectiveness of the corrections, showcasing a successful execution devoid of any compilation errors. Understanding and rectifying common causes of the cannot find symbol
error is a pivotal skill in Java development, leading to robust and error-free code.
Best Practices to Avoid Cannot Find Symbol
Errors
Descriptive and Consistent Naming
Using meaningful and consistent names for variables, methods, and classes enhances code readability and reduces the likelihood of typographical errors.
Effective Scoping
Carefully defining the scope of variables and methods ensures proper accessibility, preventing issues related to symbol visibility.
Thoughtful Package Organization
Organizing classes into logical packages with clear structures minimizes the chances of incorrect import statements and facilitates better code maintenance.
Regular Compilation and Testing
Frequent compilation and testing of code help catch symbol-related errors early in the development process, allowing for swift resolution.
Mindful Import Statements
Being vigilant with import statements ensures that necessary classes are available for use, reducing the risk of cannot find symbol
errors.
Strategic Class and Interface Implementation
When implementing interfaces or extending classes, ensure that all required methods are correctly overridden, preventing symbol-related issues during compilation.
Methodical Build Tool Configuration
In multi-module projects, configuring build tools like Maven or Gradle with attention to dependencies and the compilation order is crucial for a smooth development workflow.
Utilizing Integrated Development Environments (IDEs)
Leveraging IDE features for code completion, error highlighting, and quick fixes can aid in identifying and resolving symbol-related issues efficiently.
Continuous Learning and Code Review
Staying informed about Java best practices, learning from experiences, and conducting regular code reviews contribute to a proactive approach in preventing cannot find symbol
errors.
Conclusion
Mastering the nuances of javac cannot find symbol
is pivotal for Java developers. By addressing common causes, implementing effective solutions, and adopting best practices, one can navigate through this compilation challenge.
Thoughtful naming, scoping, and import statement usage, coupled with strategic class implementation and continuous learning, form a robust defense against such errors. Embracing these practices ensures cleaner, error-free code and a more seamless Java development experience.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Javac
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack