How to Fix java.net.SocketException: Software Caused Connection Abort: Recv Failed in Java
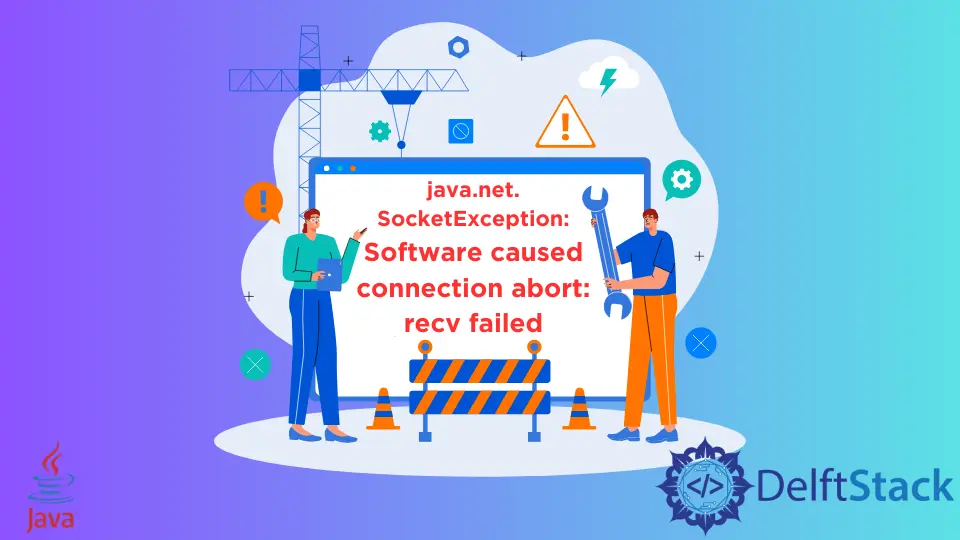
This tutorial demonstrates how to solve the following error in Java:
java.net.SocketException: Software caused connection abort: recv failed
Solve the java.net.SocketException: Software Caused Connection Abort: Recv Failed
in Java
The SocketException
is a sub-class of IOException
, which occurs when we are trying to access a socket. The Software Caused Connection Abort: Recv Failed
appears when a network error such as a timeout or the server cannot authenticate the TLS client.
Most of the time, this error occurs when using platforms like Tomcat. This error occurs when the TCP connection is reset while data is still in the buffer, which stops the connection abruptly.
This error is similar to the Connection Reset by Peers
exception. Here is an example that can throw the java.net.SocketException: Software caused connection abort: recv failed
exceptions.
BufferedReader Buffered_Reader;
String URI = "https://www.delftstack.com/";
try {
URL DEMOURL = new URL(URI);
Buffered_Reader = new BufferedReader(new InputStreamReader(DEMOURL.openStream()));
} catch (MalformedURLException e) {
throw new IOException("Enter the correct URL: " + e);
}
String DemoBuffer;
StringBuilder Result_StringBuilder = new StringBuilder();
while (null != (DemoBuffer = Buffered_Reader.readLine())) {
Result_StringBuilder.append(DemoBuffer);
}
Buffered_Reader.close();
The code above is trying to access the HTTP client, but the connection is closed abruptly. The error will occur at the line:
Buffered_Reader = new BufferedReader(new InputStreamReader(DEMOURL.openStream()));
This is because DEMOURL.openStream()
will not work properly for an HTTP client. Using Apache Commons HttpClient
will solve this issue.
The HttpClient
will re-open the connection and retry the request. Using the HttpClient
library will give us a better understanding of the error messages. The HttpClient
has connection pooling, retry mechanism, keep-alive, and many other features.
Here is a sample used for this scenario:
String URI = "https://www.delftstack.com/";
HttpClient Demo_Http_Client =
HttpClients.custom()
.setConnectionTimeToLive(10, TimeUnit.SECONDS)
.setMaxConnTotal(200)
.setMaxConnPerRoute(200)
.setDefaultRequestConfig(
RequestConfig.custom().setSocketTimeout(15000).setConnectTimeout(2500).build())
.setRetryHandler(new DefaultHttpRequestRetryHandler(3, true))
.build();
// the httpClient can be re-used as it is thread-safe and pooled.
HttpGet Http_Get_request = new HttpGet(URI);
HttpResponse Http_Response = Demo_Http_Client.execute(Http_Get_request);
Buffered_Reader = new BufferedReader(new InputStreamReader(Http_Response.getEntity().getContent()));
// handle the response.
The code above will handle the client-server operation properly, and if any network error occurs, the HttpClient
will retry the process.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack