How to Fix Java.Lang.OutOfMemoryError: Unable to Create New Native Thread
- Unable to Create New Native Thread Error in Java
-
How to Resolve the
java.lang.OutOfMemoryError: unable to create new native thread
- Conclusion
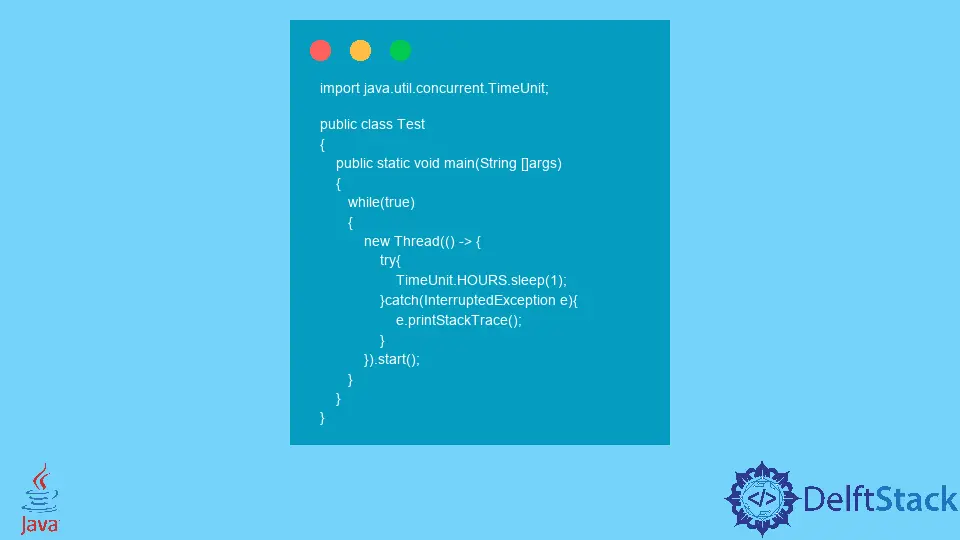
In this article, we will learn about java.lang.OutOfMemoryError: unable to create new native thread
error in Java.
Unable to Create New Native Thread Error in Java
Most real-world applications built on Java are multi-threaded in nature, with many components that perform different tasks. These tasks are executed on different threads for better throughput purposes. But the maximum number of threads the Java application can create depends on the Operating System (OS) we are using.
So, the unable to create new native thread
error is thrown by Java Virtual Machine (JVM) whenever the operating system cannot create new kernel threads, also known as system or OS threads.
The following events happen at the backend, which leads up to this error:
- The program/application inside the JVM requests the operating system for a new thread.
- A request is sent to the OS to create a new kernel thread by the JVM native code.
- The operating system tries to create a new thread that involves the memory allocation step.
- The operating system cannot allocate the memory because either the virtual memory is depleted by the OS or the memory address space is exhausted by the requesting Java application.
- The JVM throws the
unable to create new native thread
error.
Let’s see an example to understand it better.
import java.util.concurrent.TimeUnit;
public class Test {
public static void main(String[] args) {
while (true) {
new Thread(() -> {
try {
TimeUnit.HOURS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
}
}
}
In the above code, we are continuously creating threads using an infinite loop and making them wait, but since we are continuously creating while holding the threads for an hour, we will soon reach the maximum number of threads allowed by the operating system.
How to Resolve the java.lang.OutOfMemoryError: unable to create new native thread
Now, how to resolve this error, or do we have any workaround here?
One solution is to change the settings at the OS level to increase the number of threads allowed, but this solution is not feasible for two reasons.
- First, it’s not easy to reconfigure things at the OS level, and it is not recommended also.
- Second, most times, it has nothing to do with the OS thread limit; the error occurs because we have some programming error; for instance, in the program written above, we are continuously creating using an infinite loop which is not an ideal way.
Another solution or more of a workaround is to use the ExecutorService
framework to limit the number of threads in a thread pool that can be handled at a given time.
Example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
import java.util.stream.IntStream;
public class Test {
public static void main(String[] args) {
ExecutorService es = Executors.newFixedThreadPool(10);
Runnable tasks = () -> {
try {
TimeUnit.HOURS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
};
IntStream.rangeClosed(1, 15).forEach(x -> es.submit(tasks));
}
}
In the code, we have created a fixed-sized thread pool that can have a maximum of 10 threads and then we created a Runnable
task that makes the threads wait for an hour.
Using IntStream
, we submitted 15 tasks to the thread pool, so 10 will be submitted while the remaining 5 will be in the ExecutorService
queue.
Conclusion
In this article, we learned that the unable to create new native thread
error in Java occurs when the operating system cannot create new kernel threads. We also looked at a solution or workaround using the ExecutorService
framework.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack