How to Fix the Java.Lang.ClassNotFoundException: Org.Springframework.Web.Context.ContextLoaderListener Error in Java
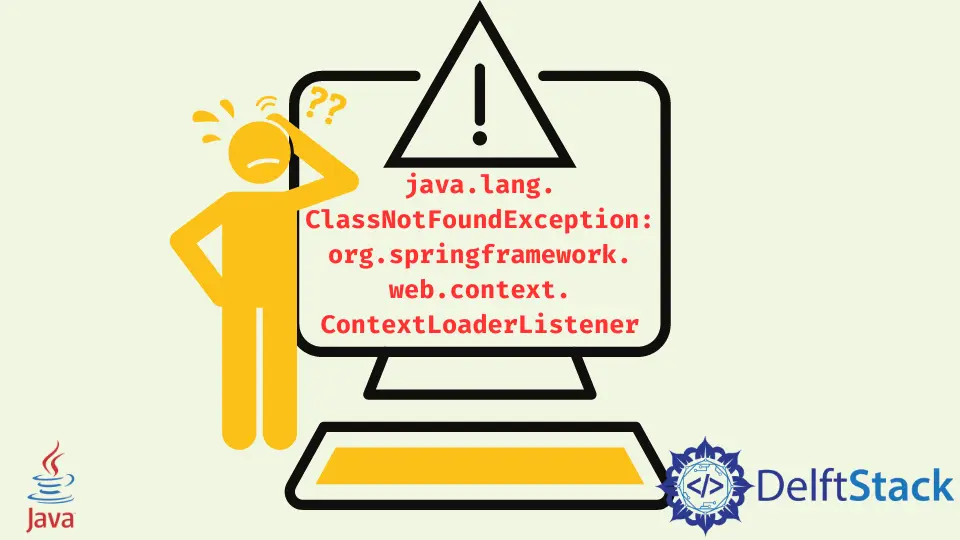
Today, we will learn about the org.springframework.web.context.ContextLoaderListener
error in Java. As the name suggests, it occurs at runtime.
We will also identify the reason for this error, leading to various possible solutions.
Prerequisites
For this tutorial, we are using the following tools and technologies.
- Apache Tomcat 9.0
- Spring MVC Framework 3.1
- Java 8
- Apache NetBeans 14 (you may use NetBeans or Eclipse)
Error Demonstration, Reasons and Solutions
Example Code (our project has the following web.xml
file):
<?xml version="1.0" encoding="ISO-8859-1" ?>
<web-app xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"
version="2.4">
<display-name>Spring MVC Application</display-name>
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/dispatcher-servlet.xml</param-value>
</context-param>
<listener>
<listener-class>
org.springframework.web.context.ContextLoaderListener
</listener-class>
</listener>
</web-app>
Example Code (our project has the following dependencies in the pom.xml
):
<dependency>
<groupId>jstl</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>3.0-alpha-1</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
The project fails during startup and results in the following error.
java.lang.ClassNotFoundException: org.springframework.web.context.ContextLoaderListener
To figure out the problem which results in this error, let’s understand what the ContextLoaderListener
is and why we are using it.
The ContextLoaderListener
is an important component of the Spring
MVC framework. Probably, it is the most vital after DispatcherServlet
itself.
It belongs to org.springframework.web.context
package. We use it in Spring
web applications to create a root
context.
Also, it is accountable for loading beans shared by many DispatcherServlet
. Generally, we use two application contexts, DispatcherServlet
and ContextLoaderListener
, while developing a Spring
MVC-based web app.
Here, the DispatcherServlet
is used to load web component-specific beans, for instance, controllers, view, handler mappings, etc., while the ContextLoaderListener
is used to load data-tier and middle-tier beans that shape the Spring
application’s back-end.
Remember, the ContextLoaderListener
is just like the other Servlet
listener, which must be declared in the deployment descriptor (also known as web.xml
) to listen to the events. Implementing ServletContextListener
only listens for shutting down and starting up the server and also creates/destroys the Spring
-managed beans.
The worth noting point is that we configure the ContextLoaderListener
in a file named web.xml
while creating a Spring
MVC app. If you are using Spring 3.1
and Spring 3.0
, then we can configure it without using deployment descriptor but only Java configurations.
At this point, we know the use of ContextLoaderListener
, and we registered in the web.xml
as required. Now, why are we getting the java.lang.ClassNotFoundException: org.springframework.web.context.ContextLoaderListener
error?
Reason for Getting ContextLoaderListener
Error
We get this error because we use the Spring
MVC framework to develop our Java web app and configure the ContextLoaderListener
as a listener in our deployment description (web.xml
file), but a jar
that contains this class
is not listed in the CLASSPATH
of the web application.
So, how to resolve this? See the following section.
Possible Solutions to Fix ContextLoaderListener
Error
There are number of ways that we can use to fix java.lang.ClassNotFoundException: org.springframework.web.context.ContextLoaderListener
error, you can use according to your situation and project requirements.
-
The
web.xml
is an important file while working using theSpring
MVC framework because it is responsible for loading ourSpring
configuration files, for instance,dispatcher-servlet.xml
andapplication-context.xml
. Make sure that you have registered theContextLoadListener
in it as follows:<context-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/dispatcher-servlet.xml</param-value> </context-param> <listener> <listener-class> org.springframework.web.context.ContextLoaderListener </listener-class> </listener>
-
If the project uses
Spring
framework version 3.0, we need to addspring-web.jar
into theCLASSPATH
. By adding into theCLASSPATH
, we mean to put that inside theWEB-INF/lib
folder. -
If the project is using
Spring
framework version 2.0 or less than that, then we need to put thespring.jar
file into theWEB-INF/lib
folder, which means we are adding this file into our app’sCLASSPATH
. -
If we have already added the
spring-web.jar
orspring.jar
file based on whatSpring
version we are using.Then, the error most probably occurs due to configuring
CLASSPATH
incorrectly. Recheck that and make it correct. -
If we are working with Maven, we must add the following Maven dependency in our
pom.xml
. This was the reason in our case.
```xml
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>3.1.0.RELEASE</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
```
We add this dependency because it serves with core `HTTP` integration to integrate with other `HTTP` technologies and web frameworks. Some of us might have `spring-webmvc`, which is also fine.
The `spring-webmvc` is the implementation of `Spring` MVC; it depends on the `spring-web`. So, including `spring-webmvc` will transitively include `spring-web`; we do not need to add that explicitly.
We can add the `spring-webmvc` as follows:
```xml
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>3.1.0.RELEASE</version>
<type>jar</type>
<scope>compile</scope>
</dependency>
```
-
If you are Eclipse friendly and getting this error while using Eclipse and Tomcat. Then, you must go through some simple steps to ensure that the
CLASSPATH
contains Maven dependencies.Further, they are visible to the web application class loader of Tomcat. You only need to do the following steps.
- Select the project. Right-click on it and choose
Properties
. - Select
Deployment Assembly
from the left side of the window. You can also come here by selecting the project, right-click on it and selectBuild Path
>Configure Build Path
. - Then select
Deployment Assembly
. You can use any option to get here. - Click on the
Add
button. It is available on the window’s right side. - Once you hit the
Add
button, you will see a new window. ChooseJava Build Path Entries
from there and clickNext
. - Select the
Maven Dependencies
from theJava Build Path Entries
menu, then clickFinish
.
- Select the project. Right-click on it and choose
We will see Maven dependencies added to the web deployment assembly definition once all steps are successfully done.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack