How to Fix Java.IO.NotSerializableException in Java
-
Reproduce the
java.io.NotSerializableException
in Java -
Causes of the
java.io.NotSerializableException
in Java -
Fix the
java.io.NotSerializableException
in Java
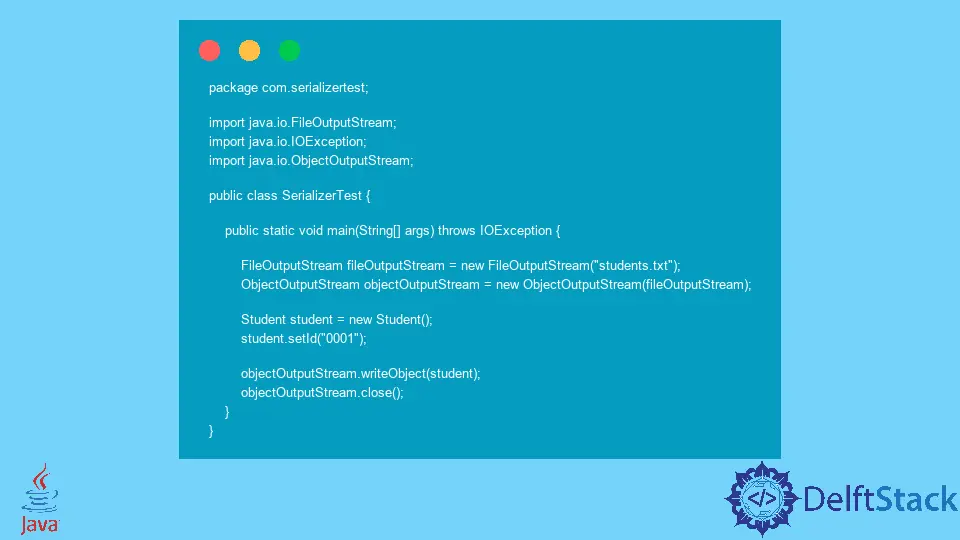
Today, we will reproduce the java.io.NotSerializableException
while writing a program in Java. We will also understand what this error means, leading to its causes and solutions.
Reproduce the java.io.NotSerializableException
in Java
Example Code (Student.java
file):
package com.serializertest;
class Student {
private String studentId;
public String getId() {
return studentId;
}
public void setId(String id) {
this.studentId = id;
}
}
The Student
class is a helper class with a member variable named studentId
. It also has member methods named getId()
and setId()
to get and set the id
of a student.
Example Code (SerializerTest.java
file):
package com.serializertest;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
public class SerializerTest {
public static void main(String[] args) throws IOException {
FileOutputStream fileOutputStream = new FileOutputStream("students.txt");
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
Student student = new Student();
student.setId("0001");
objectOutputStream.writeObject(student);
objectOutputStream.close();
}
}
The SerializerTest
is our main class where we have the main()
driver method. Inside the main()
, we create an instance of the FileOutputStream
class to create a file.
Similarly, we create another instance of the ObjectOutputStream
class.
Then, we create an object of the Student
class and call its setId()
method by passing a string argument. Next, we use the object of the ObjectOutputStream
class to write an object to the stream.
For that, we are using the writeObject()
method.
After that, we close the stream using the close()
method and run the program, but it gives us the following exception
in the program’s output console.
Exception in thread "main" java.io.NotSerializableException: com.serializertest.Student
Why are we facing this? Let’s understand the error to find out its causes below.
Causes of the java.io.NotSerializableException
in Java
It is essential to understand NotSerializableException
and Serialization
to find the causes.
Serialization
is a mechanism in Java programming that we use to write an object’s state into the byte stream. In contrast, the reverse operation of it is called Deserialization
.
The Serialization
and Deserialization
are platform-independent. It means both processes can be performed on different platforms.
We use Serialization
in Remote Method Invocation (RMI
) and Enterprise Java Beans (EJB
). It is also used in Java Persistence API (JPA
), Hibernate, etc.
The NotSerializableException
is an exception that extends the ObjectStreamException
class, defined as a superclass of all other exceptions specific to the Object Stream classes.
Additionally, the ObjectStreamException
extends the IOException
, which further signals that an I/O exception has been generated.
Now, we know the Serialization
and NotSerializableException
in Java. This discussion takes us to discover the reasons for NotSerializableException
in Java.
- The
NotSerializableException
occurs when an instance of a class must implement aSerializable
interface. - We also get this exception either by an instance of a class or the serialization runtime. Remember, the argument of
NotSerializableException
is a name of a class. - As per the documentation, the complete object graph requires to be serializable. The
NotSerializableException
also occurs if at least one of the fields does not implement theSerializable
interface while attempting to serialize an instance of a class.
Fix the java.io.NotSerializableException
in Java
We can fix the java.io.NotSerializableException
in Java using the following solutions.
-
Find the class that
throws
this exception and make itSerializable
by implementing theSerializable
interface. Remember, this solution might not be a good choice if a class that throws theNotSerializableException
belongs to the third-party library. -
We can declare the objects as
transient
if the class refers to the non-serializable objects where these objects must not be serializable. The question is, why declare it astransient
?It is because the fields of the class declared as
transient
would be ignored by serializable runtime, and we will not get any exceptions. -
If we need its data and its third party, we can consider other means of serialization. For instance, XML, JSON, etc., where we can get the third-party objects serialized without changing their definitions.
In our case, implementing the Serializable
fixed the java.io.NotSerializableException
. See the following example code.
Example Code (Students.java
file):
package com.serializertest;
import java.io.Serializable;
class Student implements Serializable {
private String studentId;
public String getId() {
return studentId;
}
public void setId(String id) {
this.studentId = id;
}
}
Example Code (SerializerTest.java
file):
package com.serializertest;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
public class SerializerTest {
public static void main(String[] args) throws IOException {
FileOutputStream fileOutputStream = new FileOutputStream("students.txt");
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
Student student = new Student();
student.setId("0001");
objectOutputStream.writeObject(student);
objectOutputStream.close();
}
}
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack