How to Fix Unsupported Major Minor Version Error in Java
-
the
Unsupported major.minor version
Error in Java -
the
Unsupported major.minor version
Error in Eclipse Project
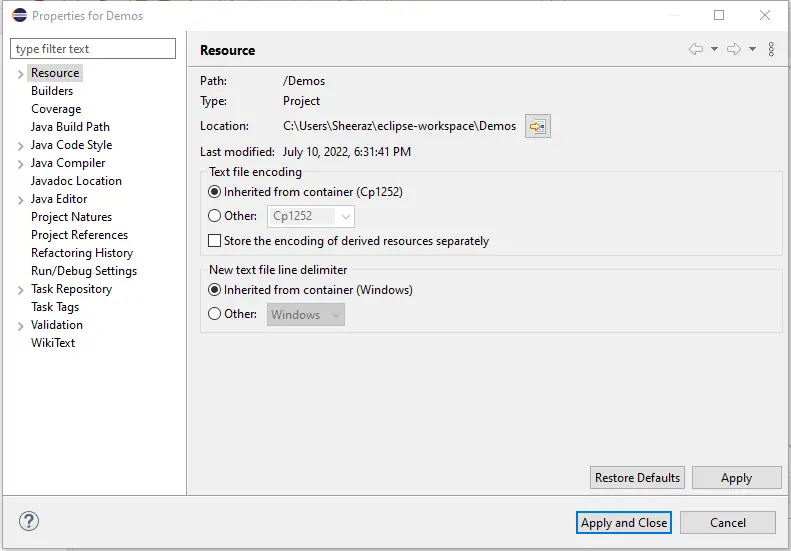
The Unsupported major.minor version
error or Java.lang.UnsupportedClassVersionError
occurs because of lower JDK during runtime and higher JDK during compile.
This tutorial demonstrates how to solve the Unsupported major.minor version
in Java.
the Unsupported major.minor version
Error in Java
The JDK should be the same during compile and runtime, or at least the compile-time JDK should not be higher than the runtime JDK; otherwise, it will throw Java.lang.UnsupportedClassVersionError
or Unsupported major.minor version
error.
Let’s try an example that will throw this error because we use Java 1.8 to compile it and 1.7 to execute it. See example:
public class Unsupported_Version_Error {
public static void main(String args[]) {
System.out.println("Hello this is Delftstack.com");
}
}
The code above will throw the unsupported version exception, as shown below.
Exception in thread "main" java.lang.UnsupportedClassVersionError: Unsupported_Version_Error : Unsupported major.minor version 52.0
at java.lang.ClassLoader.defineClass1(Native Method)
at java.lang.ClassLoader.defineClass(ClassLoader.java:800)
at java.security.SecureClassLoader.defineClass(SecureClassLoader.java:142)
at java.net.URLClassLoader.defineClass(URLClassLoader.java:449)
at java.net.URLClassLoader.access$100(URLClassLoader.java:71)
at java.net.URLClassLoader$1.run(URLClassLoader.java:361)
at java.net.URLClassLoader$1.run(URLClassLoader.java:355)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(URLClassLoader.java:354)
at java.lang.ClassLoader.loadClass(ClassLoader.java:425)
at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:308)
at java.lang.ClassLoader.loadClass(ClassLoader.java:358)
at sun.launcher.LauncherHelper.checkAndLoadMain(LauncherHelper.java:482)
The output shows the error because the code is compiled at Java 1.8 and executed at Java 1.7. because Major version 52
denotes the Java SE 8, the error Unsupported major.minor version 52.0
will be thrown.
The solution is to build the application with the same versions at compile and runtime, or at least the compile-time version is lower than the runtime version. The list of the versions of JRE compatible with the class is below.
Java SE 17 = 61,
Java SE 16 = 60,
Java SE 15 = 59,
Java SE 14 = 58,
Java SE 13 = 57,
Java SE 12 = 56,
Java SE 11 = 55,
Java SE 10 = 54,
Java SE 9 = 53,
Java SE 8 = 52,
Java SE 7 = 51,
Java SE 6.0 = 50,
Java SE 5.0 = 49,
JDK 1.4 = 48,
JDK 1.3 = 47,
JDK 1.2 = 46,
JDK 1.1 = 45
Follow the tips below to solve the Unsupported major.minor version
error.
-
We can upgrade the JRE version on our production environment to the latest version. Or at least similar to the build environment.
-
If upgrading JRE is not possible, we can downgrade the JDK on the build environment to match the version on the production environment or make it lower from the production environment.
-
One simple solution is to use the Java cross-compilation. If the production Environment JDK is lower than the build Environment, we can generate a class file with a lower version using cross-compilation.
The following command will be used to create a class file for the code above.
javac -target 1.7 Unsupported_Version_Error.java
The above command will generate the class file Unsupported_Version_Error.java
, which will be compatible with the 1.7 version of the JDK so that we can run it on the build environment.
the Unsupported major.minor version
Error in Eclipse Project
If you are using an IDE like Eclipse, then we need to change the current project’s Java version in the IDE. Follow the steps below to change the Java version in Eclipse, which will solve the Unsupported major.minor version
error.
-
Right-click on the project name in Eclipse and go to
Properties
. -
Go to
Java Build Path
thenLibraries
. -
Remove the previous
JRE System Library
. Select theJRE System Library
and clickRemove
. -
The next step is to add the
JRE System Library
. Click onAdd Library
. -
Select
JRE System Library
and clickNext
. -
Select the
Alternate JRE
option and select the latest JRE. ClickFinish
.
Following this process, our production environment JRE will be similar to or higher than the build environment and the Unsupported major.minor version
error will be solved.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack