How to Fix the Unexpected Type Error in Java
- Understanding the Unexpected Type Error
- Common Causes of Unexpected Type Errors
- How to Fix Unexpected Type Errors
- Conclusion
- FAQ
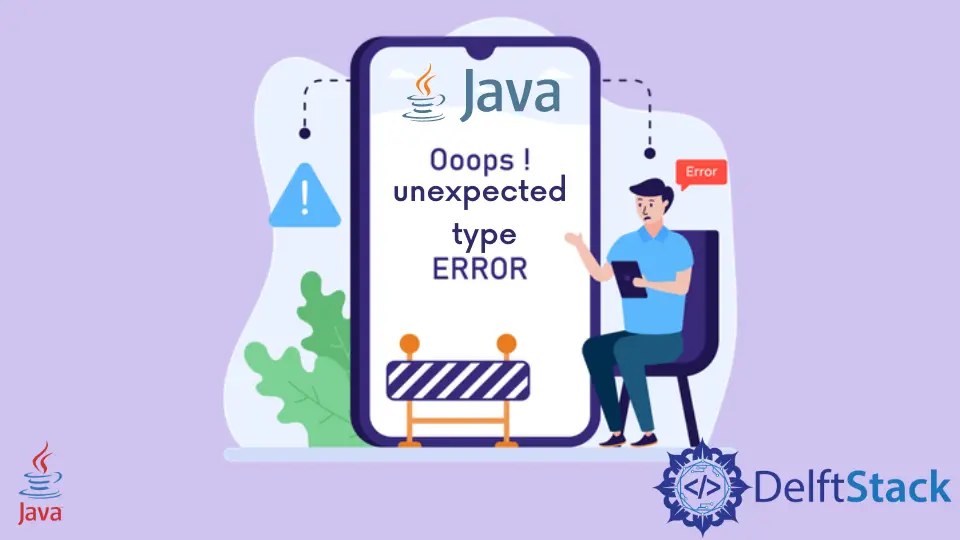
Java is a powerful programming language, but developers often encounter various errors that can be frustrating. One common issue is the unexpected type error, which typically arises during compilation when the Java compiler encounters a type mismatch. This error can disrupt your workflow and lead to confusion, especially if you’re new to Java.
In this tutorial, we will explore the unexpected type error in Java, its causes, and how to fix it effectively. Whether you’re a beginner or an experienced developer, understanding this error will enhance your coding skills and improve your overall programming experience.
Understanding the Unexpected Type Error
The unexpected type error in Java usually occurs when there is a mismatch between the expected data type and the actual data type being used in your code. This can happen in various scenarios, such as when passing parameters to methods, using generics, or working with collections. For instance, if you expect an integer but provide a string, you’ll run into this error.
To effectively address this issue, it is important to first identify the line of code causing the error. The Java compiler typically provides a message indicating where the type mismatch has occurred. Once you pinpoint the problem, you can take appropriate action to resolve it.
Common Causes of Unexpected Type Errors
-
Mismatched Data Types: This is the most straightforward cause. If a method expects a certain type and you provide a different one, the compiler will throw an unexpected type error.
-
Generics Misuse: When using collections or generics, if the type parameters do not match, you will encounter this error. For example, using a List
where a List is expected. -
Incorrect Casting: When you attempt to cast an object to an incompatible type, it can lead to unexpected type errors. Always ensure that the object you are casting is of the correct type.
-
Method Overloading: If method signatures are overloaded incorrectly, the compiler may not be able to determine which method to call based on the provided arguments, leading to type mismatches.
How to Fix Unexpected Type Errors
Method 1: Check Data Types
One of the simplest ways to fix an unexpected type error is to double-check the data types you are using. Ensure that the variable types match what is expected in your methods or operations. Here’s a simple example:
public class TypeErrorFix {
public static void main(String[] args) {
int number = 5;
String text = "Number: " + number;
System.out.println(text);
}
}
In this example, we correctly concatenate an integer with a string. If you were to mistakenly try to concatenate a string with a non-integer type, you would encounter an unexpected type error.
Output:
Number: 5
By ensuring that the data types match what is expected, you can avoid unexpected type errors. Always validate your variables before performing operations or passing them to methods.
Method 2: Use Generics Correctly
When working with collections, it is crucial to use generics appropriately. This helps prevent type mismatches. Here’s an example of how to correctly use generics:
import java.util.ArrayList;
import java.util.List;
public class GenericExample {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
for (Integer number : numbers) {
System.out.println(number);
}
}
}
In this code, we create a list of integers and add integer values to it. By specifying the generic type <Integer>
, we ensure that only integers can be added, preventing unexpected type errors.
Output:
10
20
Using generics correctly not only helps in avoiding type errors but also makes your code more readable and maintainable. Always specify the type when creating collections.
Method 3: Proper Casting
If you need to cast objects, make sure you are casting them to the correct type. Incorrect casting can lead to unexpected type errors. Here’s how to do it correctly:
class Animal {}
class Dog extends Animal {}
public class CastingExample {
public static void main(String[] args) {
Animal myAnimal = new Dog();
Dog myDog = (Dog) myAnimal;
System.out.println("Dog casted successfully.");
}
}
In this example, we create an Animal
reference that points to a Dog
object. We then cast it back to a Dog
type. If we tried to cast it to an incompatible type, such as Cat
, we would face an unexpected type error.
Output:
Dog casted successfully.
Proper casting is essential in Java, especially when dealing with inheritance. Always ensure that the object you are casting is of the correct type to avoid errors.
Method 4: Method Overloading
When overloading methods, ensure that the method signatures are unique enough for the compiler to distinguish between them. This can help prevent unexpected type errors. Here’s an example:
public class OverloadingExample {
public void display(int number) {
System.out.println("Integer: " + number);
}
public void display(String text) {
System.out.println("String: " + text);
}
public static void main(String[] args) {
OverloadingExample obj = new OverloadingExample();
obj.display(10);
obj.display("Hello");
}
}
In this code, we have two overloaded methods named display
. One accepts an integer, and the other accepts a string. This clear distinction prevents type errors when calling the methods.
Output:
Integer: 10
String: Hello
Method overloading is a powerful feature in Java. By ensuring that your method signatures are distinct, you can avoid confusion and unexpected type errors.
Conclusion
The unexpected type error in Java can be a stumbling block for many developers, but understanding its causes and solutions can make a world of difference. By checking data types, using generics correctly, ensuring proper casting, and carefully managing method overloading, you can effectively mitigate these errors. Remember, coding is a learning process, and each error presents an opportunity for growth. So, the next time you encounter an unexpected type error, you’ll be equipped with the knowledge to tackle it head-on.
FAQ
-
What is an unexpected type error in Java?
An unexpected type error occurs when there is a mismatch between the expected and actual data types in your code. -
How can I avoid unexpected type errors in Java?
You can avoid these errors by checking data types, using generics correctly, ensuring proper casting, and managing method overloading. -
What should I do if I encounter an unexpected type error?
Identify the line of code causing the error, check the data types, and make sure they match the expected types. -
Can unexpected type errors occur with collections?
Yes, using generics incorrectly with collections can lead to unexpected type errors. -
Is there a way to debug unexpected type errors?
Yes, you can use debugging tools in your IDE to step through your code and identify where the type mismatch occurs.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack