How to Fix the Java.Text.ParseException: Unparseable Date Error in Java
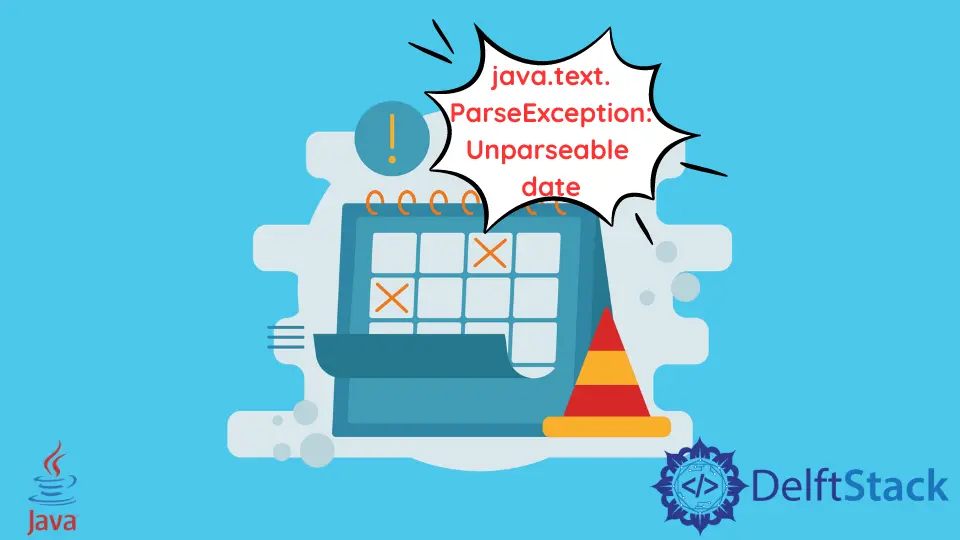
This tutorial demonstrates the java.text.ParseException: Unparseable date
error in Java.
Fix the java.text.ParseException: Unparseable date
Error in Java
The error java.text.ParseException: Unparseable date
usually occurs while using the SimpleDateFormat
class in Java. This class is used to format the date in Java.
Most of the time, the error java.text.ParseException: Unparseable date
occurs when we try to convert the string date into another desired date format. There are primarily three problems with the date string which cause this error:
When Using the Wrong Date Format
The date format is very important while parsing the date to the desired format. The date is case-sensitive in Java, so all the elements should be correct in the case.
The table below shows the correct syntax for each element of date:
Name | Syntax |
---|---|
Day |
dd |
Month |
MM |
Year |
yyyy |
Hour |
HH |
Minute |
mm |
Second |
ss |
According to the above table, the correct format of date in Java will be:
SimpleDateFormat DemoDateFormat = new SimpleDateFormat("dd-MM-YYYY HH:mm:ss");
When Using the Wrong Localization
Localization is another important factor while writing the date in Java. If we put the date in an English locale and try to parse it on another system that is not English, it will throw the java.text.ParseException: Unparseable date
error.
We need to specify the locale in the date format so there is no problem parsing the data on other systems. Because if we don’t specify, the JVM will use the default locale.
Here is the example for the locale syntax:
SimpleDateFormat DemoDateFormat = new SimpleDateFormat("dd-MM-YYYY HH:mm:ss", Locale.English);
When Using the Wrong Timezone
Using the correct timezone is also important while writing the date format in Java. The SimpleDateFormat
class is programmed to use the default timezone, the JVM’s current timezone, if not specified in the format.
The JVM timezone can change based on the user’s location, so if we don’t set the timezone in this case, there will be an error like wrong format or unexpected error.
Here is the syntax to set timezone in Java:
DemoDateFormat.setTimeZone(TimeZone.getTimeZone("UTC"));
Let’s try an example that throws the java.text.ParseException: Unparseable date
error in Java:
package delftstack;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Example {
public static void main(String[] arg) throws ParseException {
String DemoDate = "Tue Aug 16 12:30:10 IST 2022";
SimpleDateFormat Simple_Date_Format = new SimpleDateFormat("MMM d, yyyy HH:mm:ss");
Date Current_Date;
Current_Date = Simple_Date_Format.parse(DemoDate);
System.out.println(Current_Date);
}
}
The date pattern in the code above doesn’t correspond to the input string, that is why it is throwing the following error:
Exception in thread "main" java.text.ParseException: Unparseable date: "Tue Aug 16 12:30:10 IST 2022"
at java.base/java.text.DateFormat.parse(DateFormat.java:399)
at delftstack.Example.main(Example.java:13)
The solution with the correct format of date according to the given string is:
package delftstack;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
public class Example {
public static void main(String[] arg) throws ParseException {
String DemoDate = "Tue Aug 16 12:30:10 IST 2022";
SimpleDateFormat Simple_Date_Format =
new SimpleDateFormat("EE MMM dd HH:mm:ss z yyyy", Locale.ENGLISH);
Date Current_Date;
Current_Date = Simple_Date_Format.parse(DemoDate);
System.out.println(Current_Date);
}
}
The output for the code is:
Tue Aug 16 15:30:10 PKT 2022
If the given output doesn’t look good, we can add another SimpleDateFormat
and format the Current_Date
according to our choice. See example:
package delftstack;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
public class Example {
public static void main(String[] arg) throws ParseException {
String DemoDate = "Tue Aug 16 12:30:10 IST 2022";
SimpleDateFormat Simple_Date_Format =
new SimpleDateFormat("EE MMM dd HH:mm:ss z yyyy", Locale.ENGLISH);
Date Current_Date;
Current_Date = Simple_Date_Format.parse(DemoDate);
SimpleDateFormat Print_Date = new SimpleDateFormat("EE MMM d, yyyy HH:mm:ss");
System.out.println(Print_Date.format(Current_Date));
}
}
The code above prints the date in a more easy-to-read format. See output:
Tue Aug 16, 2022 15:30:10
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack