How to Create Table in Java
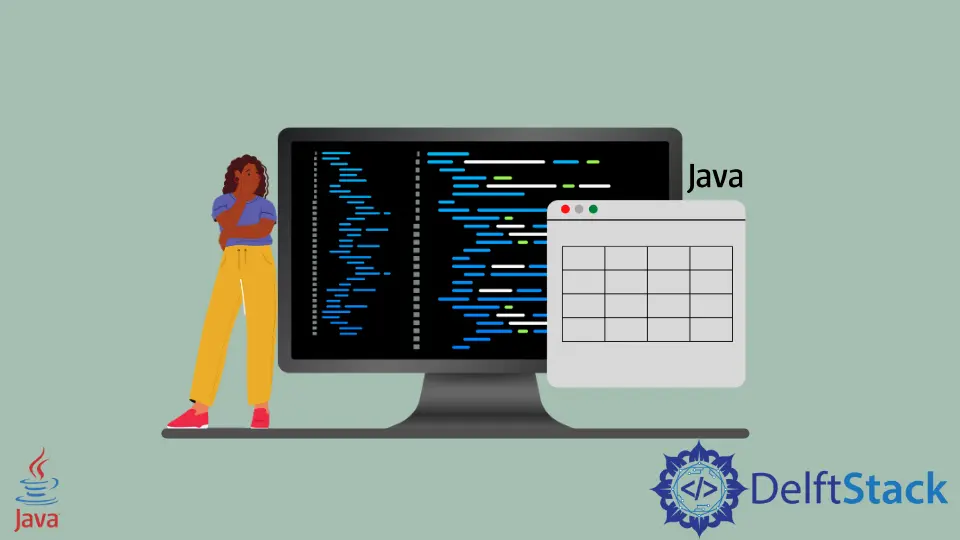
In this article, we will look at tables in Java.
We will talk about two ways to use tables in the following sections. A basic table has rows and columns to show data in a readable structure.
Use JTable
to Create Table in Java
In this example, we use the JTable
component of the GUI library Swing
in Java. We create a JFrame
object to show the table in the window; then, we create a two-dimensional array tableData
containing the raw data.
We create an array with the field names to show the column fields. Now we create an object of JTable
and pass the tableData
and tableColumn
as arguments in the constructor.
We set the size of the JTable
using the setBounds()
method. To make the table scrollable when the table data grows more than the size, we use JScrollPane
, which shows scroll bars when the components go beyond the view.
At last, we add the JScrollPane
object to the JFrame
and set the size and visibility of the frame.
import javax.swing.*;
public class JavaExample {
public static void main(String[] args) {
JFrame jFrame = new JFrame();
String[][] tableData = {{"01", "Adam", "1986"}, {"02", "John", "1990"}, {"03", "Sam", "1989"},
{"04", "Derek", "1991"}, {"05", "Ben", "1981"}};
String[] tableColumn = {"ID", "FIRST NAME", "BIRTH YEAR"};
JTable jTable = new JTable(tableData, tableColumn);
jTable.setBounds(30, 40, 230, 280);
JScrollPane jScrollPane = new JScrollPane(jTable);
jFrame.add(jScrollPane);
jFrame.setSize(350, 300);
jFrame.setVisible(true);
}
}
Output:
Use Guava Library to Create Table in Java
We can also use the Table
interface of the com.google.common.collect
package, a part of the Guava library. In the program, we create an instance of the HashBasedTable
class that implements the Table
interface by calling the create()
and returns an object of the Table
type.
The Table
has three type parameters: first is the type of keys of the row, second is the type of column keys, and the last parameter is the type of values mapped to the keys. The keys of rows and columns are associated with a single value.
We call the put()
method using the Table
object. In the put()
function, we pass three arguments: the key for the row, the column, and the last are for the value to be mapped.
We can perform several operations using the Table
interface and its classes. The row()
and column()
are two methods to fetch the values and the keys corresponding to the row or column key.
When we call table.row()
and pass the row key, we get a map in return. We use this getRows
map to call the entrySet()
that returns a Set
of the elements.
Now we use the Set
to get the stringEntry
of Map.Entry
that gives a map entry. We fetch the key and the value using the method getKey
and getValue
.
We follow the same steps to get the row key and the value using the column()
method. Methods like rowMap()
and columnMap()
return the whole table data.
The rowKeySet()
returns all the row keys in the table.
import com.google.common.collect.HashBasedTable;
import com.google.common.collect.Table;
import java.util.Map;
public class JavaExample {
public static void main(String[] args) {
Table<String, String, String> table = HashBasedTable.create();
table.put("Adam", "1990", "101");
table.put("John", "1994", "102");
table.put("Jane", "1991", "103");
table.put("John", "1995", "104");
table.put("Adam", "1996", "105");
table.put("Sam", "1991", "106");
table.put("Watson", "1994", "107");
table.put("Kelly", "1994", "108");
table.put("Martha", "1995", "109");
table.put("Billy", "1994", "107");
Map<String, String> getRows = table.row("Adam");
System.out.println("Row Results: ");
for (Map.Entry<String, String> stringEntry : getRows.entrySet()) {
System.out.println(
"Birth Year: " + stringEntry.getKey() + " | ID: " + stringEntry.getValue());
}
System.out.println();
Map<String, String> getCols = table.column("1994");
System.out.println("Column Results: ");
for (Map.Entry<String, String> stringEntry : getCols.entrySet()) {
System.out.println(
"First Name: " + stringEntry.getKey() + " | ID: " + stringEntry.getValue());
}
System.out.println();
System.out.println("Row Map Data Of Table: " + table.rowMap());
System.out.println();
System.out.println("All The Keys: " + table.rowKeySet());
}
}
Output:
Row Results:
Birth Year: 1990 | ID: 101
Birth Year: 1996 | ID: 105
Column Results:
First Name: Billy | ID: 107
First Name: John | ID: 102
First Name: Watson | ID: 107
First Name: Kelly | ID: 108
Row Map Data Of Table: {Adam={1990=101, 1996=105}, Billy={1994=107}, John={1994=102, 1995=104}, Watson={1994=107}, Jane={1991=103}, Sam={1991=106}, Martha={1995=109}, Kelly={1994=108}}
All The Keys: [Adam, Billy, John, Watson, Jane, Sam, Martha, Kelly]
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn