The findFirst() Stream Method in Java
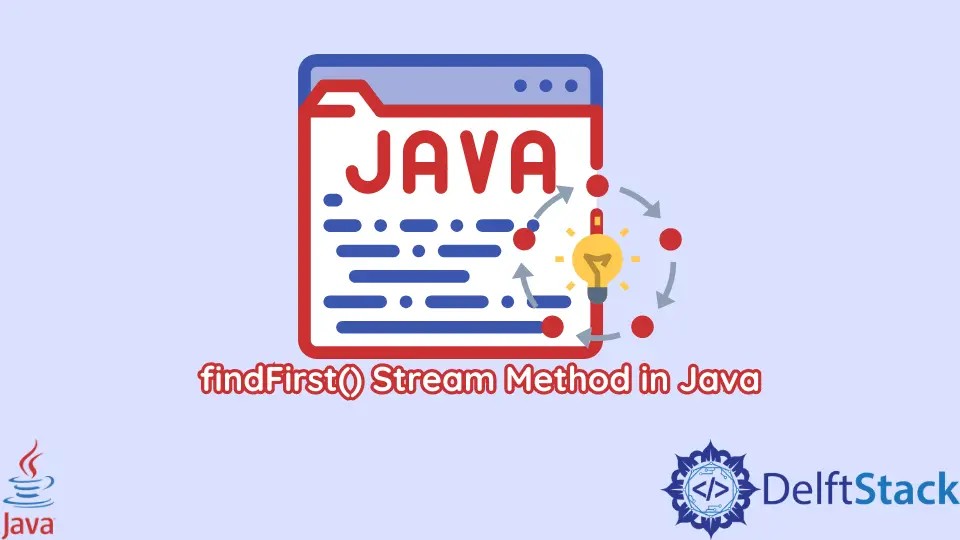
The java.util.stream
API was introduced in Java 8; it is used to process a collection of objects. Different sources such as arrays or collections can create a stream.
Here, we will look closely at findFirst()
and findAny()
Stream methods and when to use them.
findFirst()
Stream Method in Java 8
Stream does not alter the original data; it evaluates the elements of the stream using the pipeline methods.
The Stream API method findFirst()
is a terminal operation; it terminates and returns a result. We use the findfFirst()
method when we need the first element from the sequence.
This method returns an Optional<T>
, which describes the first element of the stream. Here T
is the type which is String
.
Optional
is a container object; it might or might not hold a non-null value. If the stream is empty, the Optional
returned will be empty.
We create a List of fruits
and numbers
that hold String
and Integer
type data, respectively, using the findFirst()
method in a different context. We call the .stream()
on the list that returns a stream of list elements.
We need the first element from that list, so we call the findFirst()
method on it. It returns an Optional
, which we store in first
.
We check if the Optional
instance contains a value or not using the isPresent()
method that returns a boolean
. If it returns true, we have a value, and we get it using first.get()
and print it out. If the Optional
instance is empty, we print no value.
The second statement numbers.stream().filter((x)->x>5).findFirst()
gives a second Optional
instance firstN
. We first apply the filter()
method to return a filtered stream based on a given predicate.
This method returns a new stream of elements with a value greater than 5
. From that stream, we need to get the first element. In our case, the first element with a value greater than 5
in the stream of numbers
is only 8
.
import java.util.List;
import java.util.Optional;
public class StreamTest {
public static void main(String[] args) {
List<String> fruits = List.of("Apple", "Grapes", "Orange", "Kiwi");
List<Integer> numbers = List.of(4, 5, 3, 8);
Optional<String> first = fruits.stream().findFirst();
if (first.isPresent()) {
String string = first.get();
System.out.println("First String is : " + string);
} else {
System.out.println("No value");
}
Optional<Integer> firstN = numbers.stream().filter((x) -> x > 5).findFirst();
if (firstN.isPresent()) {
System.out.println("First Number is : " + firstN.get());
} else {
System.out.println("No value");
}
}
}
Output:
First String is : Apple
First Number is : 8
findAny()
Stream Method in Java 8
The findAny()
method returns an Optional
instance describing any element of the stream. We have a list of Integer
type elements in the program below.
We create a stream from the list and filter that stream to return numbers that have a value less than 5
. Then we call findAny()
to get any number from that filtered stream.
In most cases, it will return the first element. If the Optional
instance firstN
is not empty, we print the instance value we get using the get()
method.
import java.util.List;
import java.util.Optional;
public class StreamTest {
public static void main(String[] args) {
List<Integer> numbers = List.of(4, 5, 2, 8);
Optional<Integer> firstN = numbers.stream().filter((x) -> x < 5).findAny();
if (firstN.isPresent()) {
System.out.println("Any First Number less then 5 is : " + firstN.get());
} else {
System.out.println("No value");
}
}
}
Output:
Any First Number less then 5 is : 4
Note that in the case of both sequential and parallel streams, the behavior of the methods findAny()
and findFirst()
won’t’ change. The behavior of findAny()
in the case of non-parallel streams is not guaranteed.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn