How to Fix Java StackOverflowError
-
the
java.lang.StackOverflowError
in Java -
Causes of
java.lang.StackOverflowError
-
Solution of
java.lang.StackOverflowError
in Java
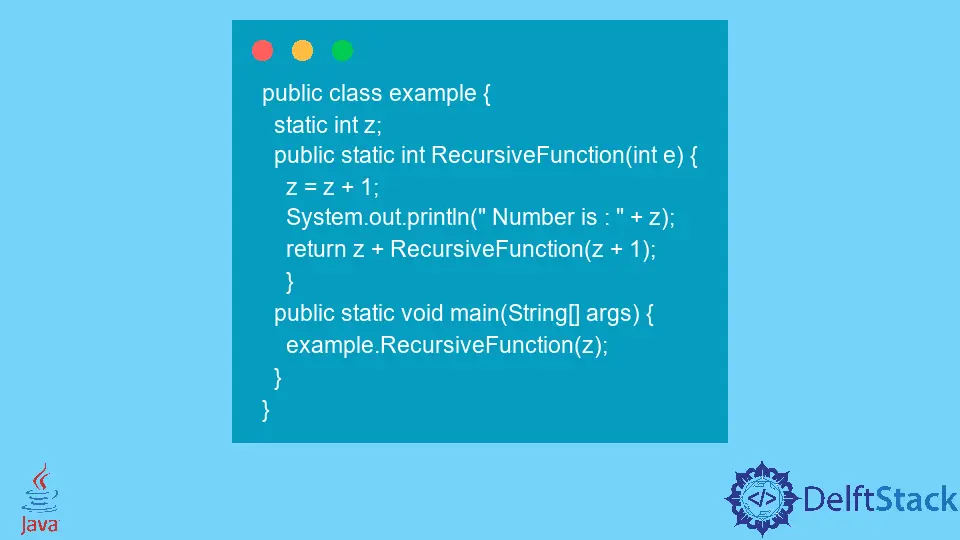
This article tackles the possible causes of the java.lang.StackOverflowError
error in the main()
method of a Java program.
the java.lang.StackOverflowError
in Java
The java.lang.StackOverflowError
is thrown in Java to signal that the application’s stack has been depleted due to particularly deep recursion. The java.lang.StackOverflowError
class is an extension of the VirtualMachineError
class, which signals that the JVM
is either malfunctioning or has exhausted all of its resources and cannot function.
Causes of java.lang.StackOverflowError
Let’s look at an example to comprehend java.lang.StackOverflowError
and the reasons why it occurs.
We will first include a main method in the example class. After that, we’ll build a function named RecursiveFunction()
, which will be called recursively within the main method.
In the RecursiveFunction()
function, we will display the numbers with an increment of 1
added after each call.
Complete Source Code:
public class example {
static int z;
public static int RecursiveFunction(int e) {
z = z + 1;
System.out.println(" Number is : " + z);
return z + RecursiveFunction(z + 1);
}
public static void main(String[] args) {
example.RecursiveFunction(z);
}
}
You will get similar results, such as the one shown below.
Output:
Exception in thread “main” java.lang.StackOverflowError
at java.io.PrintStream.write(Unknown Source)
at java.io.PrintStream.print(Unknown Source)
at java.io.PrintStream.println(Unknown Source)
at StackOverflowErrorClass.RecursiveFunction(StackOverflowErrorClass.java:7)
at StackOverflowErrorClass.RecursiveFunction(StackOverflowErrorClass.java:8)
at StackOverflowErrorClass.RecursiveFunction(StackOverflowErrorClass.java:8)
.
.
.
Let’s talk about the issues in the example program that causes the java.lang.StackOverflowError
.
- We discussed an example where the stack of the
thread
is where the methods, primitivedatatypes
,variables
,object references
, andreturn
values are stored while thethread
is performing them. All of them are using memory. - A
java.lang.StackOverflowError
will be triggered if athread's
stack size increases to the point where it exceeds the maximum amount of memory it has been allotted. Main()
method callsRecursiveFunction()
function in this program. TheRecursiveFunction()
method repeatedly invokes itself and will be called infinitely due to this implementation.
When this happens, there will be an unlimited number of calls to the RecursiveFunction()
method. Thread's
stack size limit would be surpassed after a few hundred or thousand iterations.
The code we discussed prints the numbers but does not have a condition that causes it to end. This is the cause of the java.lang.StackOverflowError
, which indicates that the stack’s size has been totally used up and cannot be expanded further.
Solution of java.lang.StackOverflowError
in Java
The following are possible solutions to the java.lang.StackOverflowError
exception.
Find Recursive Lines
We must properly analyze the thread
stack and look for a pattern of lines that keeps occurring. These lines point to the code being called upon recursively.
After we have found these lines, we need to do a comprehensive analysis of the code to determine whether the recursion never comes to an end.
Increase Thread Size
We have the choice to increase the size of the Thread
stack to make room for a more significant number of commands. Altering the parameters of the compiler allows for an increase in the maximum size of the Thread
stack.
Termination Condition
To prevent making the same calls repeatedly, you need to make an effort to implement a correct terminating condition or any other condition for the recursive calls to assure that it terminates.
Let’s add a termination condition so that we may fix the problem that occurred in the following example code. We’ll use the if
statement to check whenever z
reaches 19
; it will terminate the function.
if (z == 19)
return z;
Complete Source Code:
public class example {
static int z;
public static int RecursiveFunction(int e) {
z = z + 1;
System.out.println(" Number is : " + z);
if (z == 19)
return z;
return z + RecursiveFunction(z + 1);
}
public static void main(String[] args) {
example.RecursiveFunction(z);
}
}
Output:
Number is : 1
Number is : 2
Number is : 3
Number is : 4
Number is : 5
Number is : 6
Number is : 7
Number is : 8
Number is : 9
Number is : 10
Number is : 11
Number is : 12
Number is : 13
Number is : 14
Number is : 15
Number is : 16
Number is : 17
Number is : 18
Number is : 19
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack