How to Solve the Java.Sql.SQLException: No Suitable Driver Error in Java
-
Cause of the
java.sql.SQLException: No suitable driver
Error in Java -
Register the JDBC Driver to Solve the
java.sql.SQLException: No suitable driver
Error in Java -
Add the JDBC Driver to ClassPath to Solve the
java.sql.SQLException: No suitable driver
Error in Java
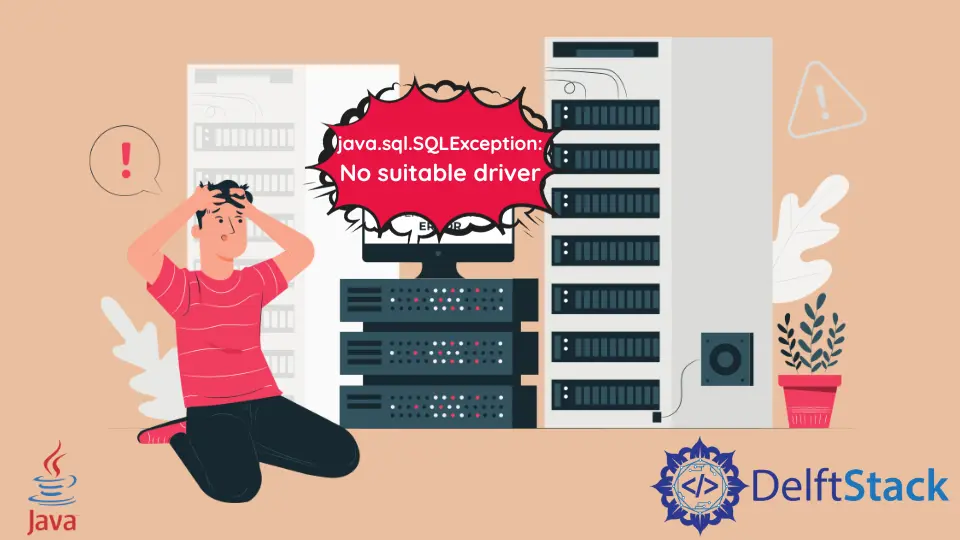
This tutorial demonstrates the java.sql.SQLException: No suitable driver
error in Java.
Cause of the java.sql.SQLException: No suitable driver
Error in Java
The error java.sql.SQLException: No suitable driver
occurs when we are trying to connect to MySQL or any other database and trying to listen to a port. The no suitable driver corresponds to java.sql.SQLException: No suitable driver found for jdbc:mysql://localhost:3306/test
in the console.
The reasons for this error are:
- When there is no JDBC driver registered for the database and port before invoking the
DriverManager.getConnection()
. - When the MySQL JDBC driver is not added to the classpath.
The solutions for the java.sql.SQLException: No suitable driver
errors are given in the following sections.
Register the JDBC Driver to Solve the java.sql.SQLException: No suitable driver
Error in Java
If your JDBC driver is not registered, then the JDBC URL is not accepted by any of the loaded drivers using the method acceptsURL
. To solve this problem, mention the MySQL JDBC driver, as shown below:
MySQL JDBC URL:
jdbc:mysql://localhost:3306/test?useSSL=false
Prototype of acceptsURL
:
boolean acceptsURL(String url) throws SQLException
The complete syntax for database connection will be:
DataBase_Connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/Your_DatabaseName?useSSL=false", "Your_UserName", "Your_Password");
Here is a simple example of how you can register a JDBC driver and make a successful database connection:
package delftstack;
import java.sql.Connection;
import java.sql.DriverManager;
public class Example {
public static void main(String[] args) {
Connection Database_Connection = null;
try {
Class.forName("com.mysql.jdbc.Driver");
Database_Connection = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/test?useSSL=false", "admin", "123456");
System.out.println("Database is successfully connected.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
The Class.forName("com.mysql.jdbc.Driver");
will force the driver to register itself. After that, the database will be connected with the correct URL.
See output:
Database is successfully connected.
Add the JDBC Driver to ClassPath to Solve the java.sql.SQLException: No suitable driver
Error in Java
To add the JDBC driver to the classpath, we need to download the MySQL Connector Jar, which also includes the JDBC driver. Follow the steps below to add the JDBC driver to the classpath of IDE:
-
Download the
MySQL Connector
jar from here. -
Extract the downloaded file.
-
Go to the
Properties
of your Java project in your IDE. In our case, the IDE is Eclipse. -
Go to Java Build Path and click Add External Jars.
-
Select the
MySQL Connector
jar and click Open. -
Once the jar is added, click Apply and Close.
The above steps will add the JDBC driver to your classpath; now, the error java.sql.SQLException: No suitable driver
will be solved.
Usually, any one of these solutions will fix the exception java.sql.SQLException: No suitable driver
. Because either it is a syntax problem or a problem with the classpath.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack