Java Paint Component
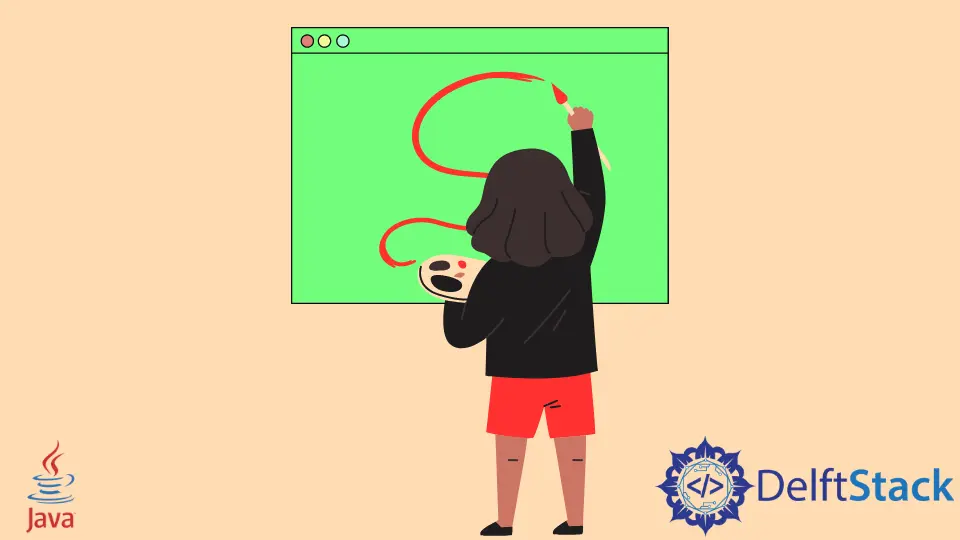
First, we’ll go over how the Java paintComponent()
method works. When does it invoke, and what is the structure of its subsystems and classes?
Finally, we’ll show you how to use this method to draw graphics on the screen. Keep reading to find out more.
the PaintComponent()
Method in Java
Consider a location where you can keep all of your painting and graphics code. Consider that it is locked by default and will only let you in when you request.
What does it indicate? When it’s time to paint, this method is initiated.
Painting, on the other hand, starts higher up the class hierarchy. Analyze its class hierarchy: java.awt.Component
.
The painting subsystem will call this method whenever your component needs to be rendered.
How does the Java paintComponent()
get invoked? The Java AWT supports a callback mechanism.
It is worth noting that the AWT callback mechanism is the same for both heavyweight and lightweight components. These components are used for painting irrespective of how a particular paint request is invoked/triggered.
A program should place the rendering code inside a particular overridden method. And the same toolkit will call this method when it’s time to paint.
java.awt.Component
.Syntax:
import java.awt.*;
import javax.swing.*;
public void paintComponent(Graphics g) {
// your code goes here
}
In addition, javax.swing.JComponent
extends this class by partitioning the paint
method into three distinct methods.
protected void paintComponent(Graphics g)
protected void paintBorder(Graphics g)
protected void paintChildren(Graphics g)
We believe that you have understood the basics of this method until now. Now, let us discuss another method we usually use with the paintComponent()
.
Also, now is not the time to hand it over to the previously discussed system. We can, nevertheless, ask the system to freshen up the display using the repaint()
method.
repaint()
leads to the call to paintComponent()
.Best practice to use Paint
:
- Do not place rendering code anywhere; it could be invoked outside of the scope of the paint callback. Such code may be invoked when it is inappropriate to paint.
- Before the component is visible or has access to a valid Graphics object, it is not recommended that programs directly call
paint()
.
A paint operation is requested asynchronously by AWT. Check out the following code block to have an idea.
Code:
public void repaint() public void repaint(long xyz) public void repaint(int a, int b,
int definewidth,
int defineheight) public void repaint(long xyz, int a, int b, int definewidth, int defineheight)
Here is a complete Java demo example.
Code:
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
// main class
@SuppressWarnings("serial")
class PainComponentDemo extends JPanel {
public PainComponentDemo() {
// setting background
setBackground(Color.CYAN);
}
@Override
// override java paintComponent
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawOval(0, 0, getWidth(), getHeight());
}
// driver function
public static void main(String[] args) {
// extend jfram
JFrame custJF = new JFrame();
custJF.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
custJF.setSize(500, 499);
custJF.add(new PainComponentDemo());
custJF.setVisible(true);
} // driver function ends
} // main class ends
Output:
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn