How to Fix Java NoClassDefFoundError Error
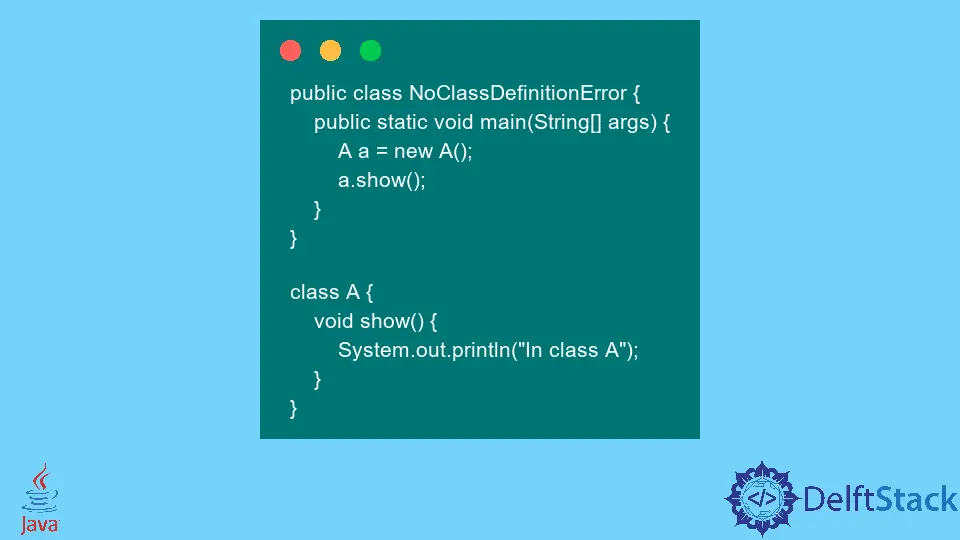
In the Java language, errors are anomalies that mainly occur due to a lack of resources. Additionally, errors cannot be caught at the compile time and occur at run time.
The errors are also called unchecked exceptions. One cannot check if any error can occur or not even the compilation gets done successfully.
The variety of errors that are present under Error
class are AssertionError
, NoClassDefFoundError
, OutOfMemoryError
, etc.
The NoClassDefFoundError
is a sub-type of the Error
class that says or denotes the Java Runtime Environment or (JRE) cannot load the class definition, which was present at the time of compilation but is not available at runtime. The Error
class resides inside the javas.lang
package.
The NoClassDefFoundError
class resides under LinkageError
. The Error
is a type of linkage issue that comes into action or encounters when we do not use proper IDE (Integrated Development Environment) like IntelliJ IDEA, Eclipse, etc., and use the console to run the program.
The IDE’s work in a way that compiles the classes that are dependent on the main class beforehand. But in a console or terminal, it is the responsibility of the user to compile all the dependent classes and then run the main class, thereby reducing the risk of the linkage type error.
Let’s understand the error using a Java code block.
public class NoClassDefinitionError {
public static void main(String[] args) {
A a = new A();
a.show();
}
}
class A {
void show() {
System.out.println("In class A");
}
}