How to Fix java.net.ConnectException Error in Java
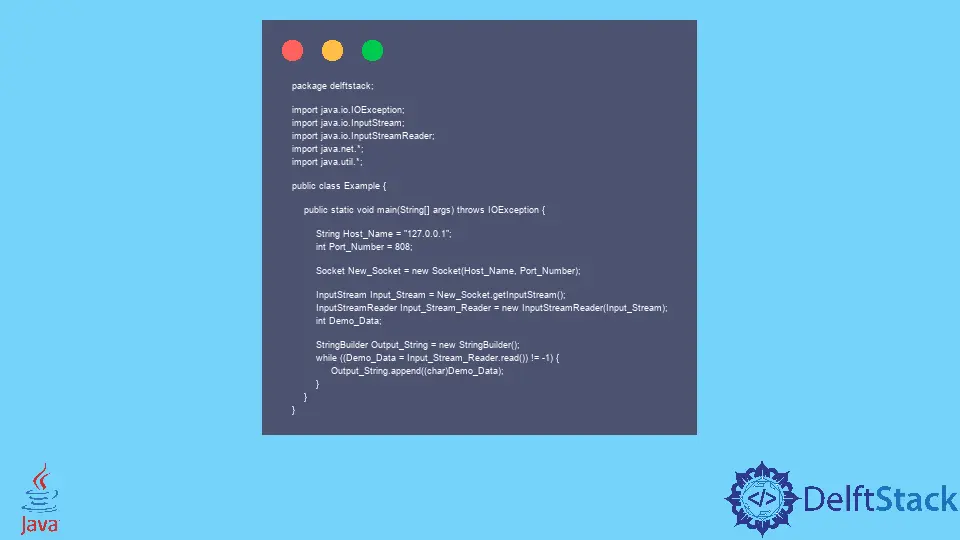
This tutorial demonstrates the java.net.ConnectException
error in Java.
the java.net.ConnectException
Error in Java
The java.net.ConnectException
is the most common exception while working with networking. It mostly occurs when making a TCP connection between the client, application, and server.
It is a checked application that can be handled in the code using the try-catch blocks. This exception is a sub-class of the IO exception.
Here is the hierarchy of this exception:
java.lang.Object
java.lang.Throwable
java.lang.Exception
java.io.IOException
java.net.SocketException
java.net.ConnectException
Here are the possible reasons for this exception:
- When any client and server are not in the same network, both should be in the network like internet or LAN; otherwise, it will throw the
ConnectException
. - When the server is not running. Usually, the ports like 3306 (MySQL), 8080 (for Tomcat), 27017 (MongoDB), and 3000 or 4200 (for React/Angular) are completely down or occupied by some other entity, and it will throw the
ConnectException
. - When the security is disallowing communication, for example, in the case of a Firewall. In this case, it will throw the
ConnectException
. - When the settings are overridden, the server may be running but listing on ports, it will throw the
ConnectException
. - When the connection string is not correct, for example,
Connection DemoConnection = DriverManager.getConnection("jdbc:mysql://localhost/:3306<databasename>?" + "user=<username>&password=<password>");
. It will throw theConnectException
. - When the wrong port number is given by mistake, it will throw the
ConnectException
.
Let’s try an example for ConnectException
:
package delftstack;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.*;
import java.util.*;
public class Example {
public static void main(String[] args) throws IOException {
String Host_Name = "127.0.0.1";
int Port_Number = 808;
Socket New_Socket = new Socket(Host_Name, Port_Number);
InputStream Input_Stream = New_Socket.getInputStream();
InputStreamReader Input_Stream_Reader = new InputStreamReader(Input_Stream);
int Demo_Data;
StringBuilder Output_String = new StringBuilder();
while ((Demo_Data = Input_Stream_Reader.read()) != -1) {
Output_String.append((char) Demo_Data);
}
}
}
The code above will throw the ConnectException
because the port and IP are invalid. See output:
Exception in thread "main" java.net.ConnectException: Connection refused: connect
at java.base/sun.nio.ch.Net.connect0(Native Method)
at java.base/sun.nio.ch.Net.connect(Net.java:579)
at java.base/sun.nio.ch.Net.connect(Net.java:568)
at java.base/sun.nio.ch.NioSocketImpl.connect(NioSocketImpl.java:588)
at java.base/java.net.SocksSocketImpl.connect(SocksSocketImpl.java:327)
at java.base/java.net.Socket.connect(Socket.java:633)
at java.base/java.net.Socket.connect(Socket.java:583)
at java.base/java.net.Socket.<init>(Socket.java:507)
at java.base/java.net.Socket.<init>(Socket.java:287)
at delftstack.Example.main(Example.java:16)
Now, we can handle the exception by using the try-catch
blocks. As it is a checked exception, it is necessary to add throws
to the method.
Let’s see the solution:
package delftstack;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.*;
import java.util.*;
public class Example {
public static void main(String[] args) throws IOException {
String Host_Name = "127.0.0.1";
int Port_Number = 808;
try (Socket New_Socket = new Socket(Host_Name, Port_Number)) {
InputStream Input_Stream = New_Socket.getInputStream();
InputStreamReader Input_Stream_Reader = new InputStreamReader(Input_Stream);
int Demo_Data;
StringBuilder Output_String = new StringBuilder();
while ((Demo_Data = Input_Stream_Reader.read()) != -1) {
Output_String.append((char) Demo_Data);
}
}
catch (ConnectException ex) {
System.out.println(
"The Connect Exception occured becuase the given hostname and port are invalid : "
+ ex.getMessage());
}
}
}
Now the exception is handled, so it will not crash the code. See output:
The Connect Exception occured becuase the given hostname and port are invalid : Connection refused: connect
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack