How to Fix Missing Return Statement Error Type in Java
-
Why Does the
missing return statement
Error Occur in Java -
Fix
Missing Return Statement
Error Type in Java
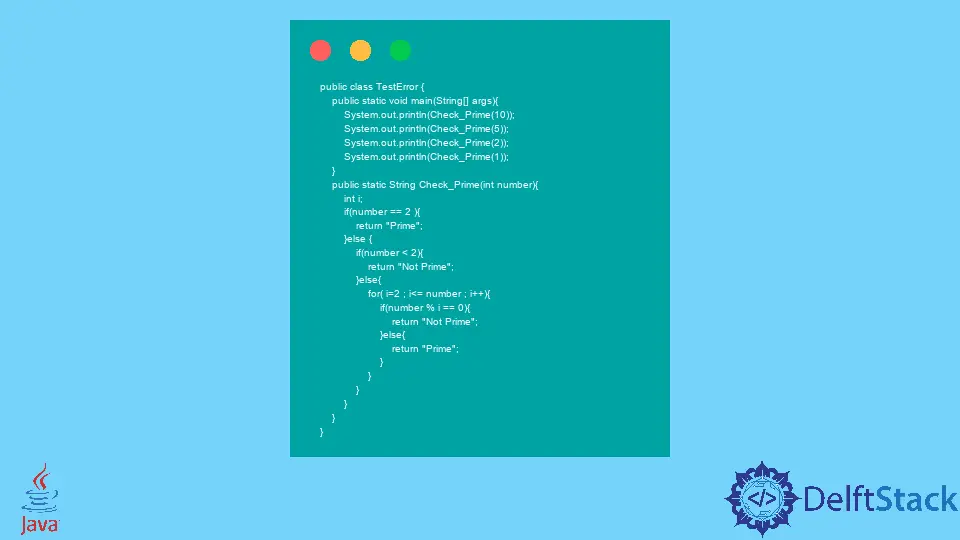
The Java compiler might report a missing return statement
error when using the if
, for
, or while
loops in a method.
This article explains why this happens and how to deal with it.
Why Does the missing return statement
Error Occur in Java
One of the most common Java errors is a missing return statement
.
It is an error that occurs during the compilation process. As the statement implies, the issue is usually caused by a missing return statement in the program.
Let’s take a program for reference that can produce this error.
Here is a method that returns a String
type variable after determining whether or not a number passed as an argument is prime. This method utilizes the if
, else
, and for
loops.
When we look at this program carefully, we can see the first pair of the if-else
loop, each returning a String
. In the else
loop, we run a for
loop that in turn returns a String
using the if
and else
.
It looks alright at first glance. But after careful examination, we can find that the else
statement doesn’t return anything in case the for
loop fails.
In other words, the compiler doesn’t know that the for
loop inside the else
loop will return a String
. It creates ambiguity.
Even if the for
loop runs and returns a type, the compiler has to assume that there’s a possibility of it not returning anything. So to clear this confusion for the compiler, the else
method should return a String
type.
Java documentation suggests that if we declare a method with a return type, there needs to be a return
statement at the end of the method. Otherwise, the compiler will show a missing return statement
error.
This error is thrown when we mistakenly omit the return statement of the method, as it does not have a return type or has not been declared using the void
type.
public class TestError {
public static void main(String[] args) {
System.out.println(Check_Prime(10));
System.out.println(Check_Prime(5));
System.out.println(Check_Prime(2));
System.out.println(Check_Prime(1));
}
public static String Check_Prime(int number) {
int i;
if (number == 2) {
return "Prime";
} else {
if (number < 2) {
return "Not Prime";
} else {
for (i = 2; i <= number; i++) {
if (number % i == 0) {
return "Not Prime";
} else {
return "Prime";
}
}
}
}
}
}
Fix Missing Return Statement
Error Type in Java
public class TestError {
public static void main(String[] args) {
System.out.println(Check_Prime(10));
System.out.println(Check_Prime(5));
System.out.println(Check_Prime(2));
System.out.println(Check_Prime(1));
}
public static String Check_Prime(int number) {
int i;
int k = number / 2;
if (number == 2) {
return "Prime";
} else {
if (number < 2) {
return "Not Prime";
} else {
for (i = 2; i <= k; i++) {
if (number % i == 0) {
return "Not Prime";
} else {
return "Prime";
}
}
return "";
}
}
}
}
Output:
Not Prime
Prime
Prime
Not Prime
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack