How to Resolve Missing Method Body or Declare Abstract in Java
- Understanding the Error
- Scenario 1: Missing Method Body in a Concrete Class
- Scenario 2: Declaring Abstract Methods in Abstract Classes
- Scenario 3: Interfaces and Method Implementation
- Conclusion
- FAQ
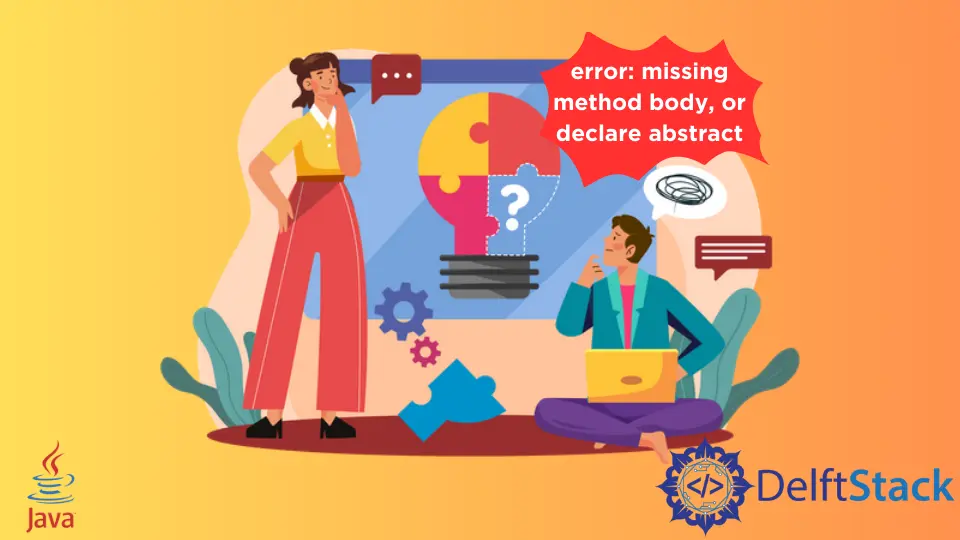
When programming in Java, developers often encounter various errors that can halt their progress. One such common error is the “missing method body or declare abstract” message. This error typically arises when the Java compiler expects a method to have an implementation but finds none. It can be frustrating, especially for beginners, but understanding the reasons behind it can help you troubleshoot effectively.
In this tutorial, we will explore the causes of this error and provide practical solutions to resolve it. Whether you are new to Java or an experienced developer, this guide aims to enhance your understanding and improve your coding skills.
Understanding the Error
The “missing method body or declare abstract” error occurs when a method is declared but lacks an implementation. This often happens in two scenarios: when a method is declared in an interface or an abstract class without being implemented in a concrete class. The Java compiler requires that all methods in a concrete class must have a body unless the class itself is declared abstract.
Let’s delve deeper into the situations that lead to this error and how to fix them.
Scenario 1: Missing Method Body in a Concrete Class
In this scenario, you may have declared a method in a concrete class without providing its implementation. Here’s how you can resolve this issue.
class MyClass {
void myMethod(); // Missing method body
}
To fix this, you need to provide a proper implementation for the method:
class MyClass {
void myMethod() {
System.out.println("Hello, World!");
}
}
Output:
Hello, World!
In this corrected example, the myMethod
now has a body that prints a message to the console. By providing the implementation, the error is resolved, and the Java compiler can successfully compile the class. Remember, every non-abstract class must implement all its methods. If you do not intend to provide an implementation, consider declaring the class as abstract.
Scenario 2: Declaring Abstract Methods in Abstract Classes
If you are working with abstract classes, you can declare methods without providing a body. However, the class itself must be declared as abstract. Here’s an example that illustrates this scenario.
abstract class MyAbstractClass {
abstract void myAbstractMethod(); // Abstract method
}
Now, if you create a subclass of this abstract class, you must implement the abstract method:
class MyConcreteClass extends MyAbstractClass {
void myAbstractMethod() {
System.out.println("Abstract method implemented.");
}
}
Output:
Abstract method implemented.
In this case, MyAbstractClass
defines an abstract method, which is valid. The subclass MyConcreteClass
provides the implementation for that method. If you forget to implement the abstract method in the subclass, you will encounter the same missing method body error. Always ensure that any subclass of an abstract class implements all abstract methods.
Scenario 3: Interfaces and Method Implementation
In Java, interfaces can also declare methods without bodies. If a class implements an interface, it must provide implementations for all the interface’s methods.
Here’s how you might run into trouble:
interface MyInterface {
void myInterfaceMethod(); // Method without a body
}
class MyClass implements MyInterface {
// Missing implementation for myInterfaceMethod
}
To resolve this, you need to implement the method in your class:
class MyClass implements MyInterface {
public void myInterfaceMethod() {
System.out.println("Interface method implemented.");
}
}
Output:
Interface method implemented.
By implementing myInterfaceMethod
, the error is resolved. This is a crucial aspect of working with interfaces in Java. Remember, if you implement an interface, you must provide implementations for all its methods, or else your class will also need to be declared abstract.
Conclusion
Understanding the “missing method body or declare abstract” error in Java is essential for any developer. By recognizing the scenarios where this error occurs, you can effectively troubleshoot and resolve it. Whether you are dealing with concrete classes, abstract classes, or interfaces, ensuring that all declared methods are properly implemented or declared abstract is key to writing functional Java code. With these insights, you can avoid common pitfalls and enhance your coding proficiency.
FAQ
-
what causes the missing method body error in Java?
The error occurs when a method is declared without an implementation in a concrete class or when an abstract method is not implemented in a subclass. -
how can I fix the missing method body error?
Provide an implementation for the method in the class where it is declared, or declare the class as abstract if it contains abstract methods. -
what is the difference between an abstract class and an interface in Java?
An abstract class can have both abstract and concrete methods, while an interface can only declare methods without implementations. -
can I declare a method without a body in a concrete class?
No, all methods in a concrete class must have implementations. If you want to declare a method without a body, the class must be declared abstract. -
what happens if I forget to implement an abstract method in a subclass?
If you forget to implement an abstract method in a subclass, you will encounter a compilation error, and the subclass must also be declared abstract.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack