How to Fix Java.Lang.StringIndexOutOfBoundsException: String Index Out of Range
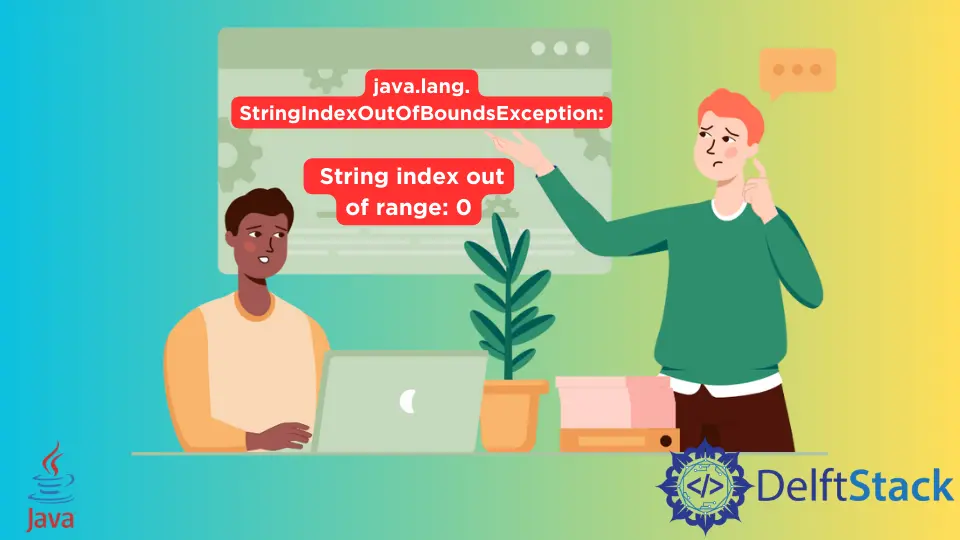
This tutorial demonstrates the Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 0
error in Java.
Fix the java.lang.StringIndexOutOfBoundsException: String index out of range
Error
The StringIndexOutofBoundsException
is an unchecked exception that occurs when accessing the character of a string for which the index is either negative or more than the String length. This exception is also thrown when we use the charAt()
method, which is trying to access a character that is not in the String.
Because StringIndexOutofBoundsException
is an unchecked exception, it doesn’t need to add to throws of a method or constructor; we can handle it using the try-catch
block. Here are the specific scenarios where this exception can occur:
- When using
String.charAt(int index)
, which will return a character at a given String index. If the index is negative or greater than the String’s length, it will throw theStringIndexOutofBoundsException.
- When using
String.valueOf(char[] data, int offset, int count)
which returns a string with given arguments. It will throw theStringIndexOutofBoundsException
when any index is negative oroffset + count
is greater than the size of the array. - When using
CharSequence.subSequence(int beginIndex, int endIndex)
which returns a character sequence. It will throw theStringIndexOutofBoundsException
whenbeginIndex
is greater thanendIndex,
orendIndex
is greater than the length of the String. - When using
String.substring(int beginIndex)
, which returns a substring from the given String. It will throw theStringIndexOutofBoundsException
whenbeginIndex
is negative or greater than the length of the String. - When using
String.substring(int beginIndex, int endIndex)
, which returns a sub-string between a specific range. It will throw theStringIndexOutofBoundsException
when any index is negative, orendIndex
is greater than the length of String ofbeginIndex
is greater thanendIndex
.
Let’s try an example for StringIndexOutofBoundsException
. See example:
package delftstack;
public class Example {
public static void main(String[] arg) {
String DemoStr = "delftstack";
System.out.println(DemoStr.charAt(11));
}
}
The code above will throw StringIndexOutofBoundsException
because there is no character at index 11. See output:
Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 11
at java.base/java.lang.StringLatin1.charAt(StringLatin1.java:48)
at java.base/java.lang.String.charAt(String.java:1512)
at delftstack.Example.main(Example.java:7)
The StringIndexOutOfBoundsException
can be handled using try-catch
blocks. See solution:
package delftstack;
public class Example {
public static void main(String[] arg) {
String DemoStr = "delftstack";
try {
System.out.println(DemoStr.charAt(11));
} catch (StringIndexOutOfBoundsException e) {
System.out.println("Please insert a index under String length: " + DemoStr.length());
}
}
}
Now the output for the above code is:
Please insert a index under String length: 10
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack