How to Solve the Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
-
the
Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
Error -
Solve the
Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
Error
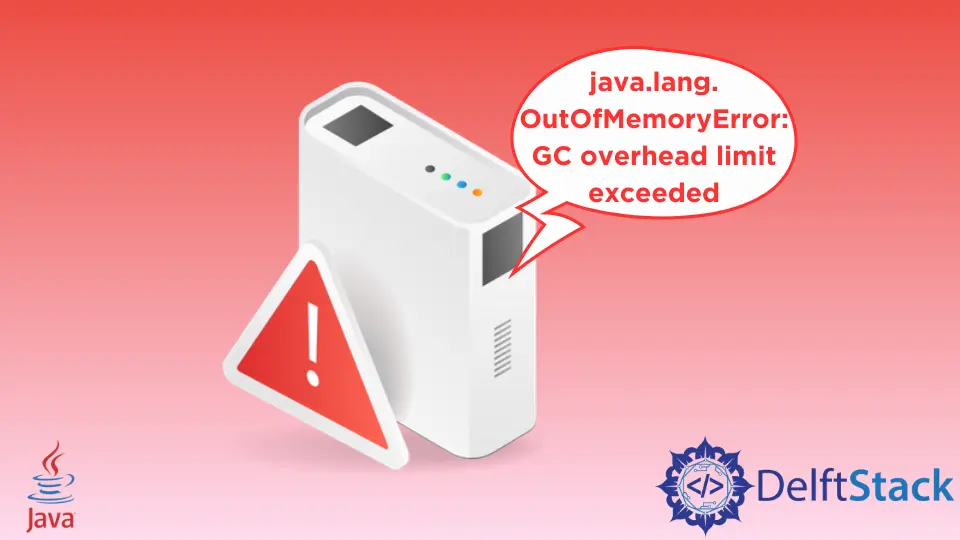
This tutorial demonstrates the Java.lang.outofmemoryerror: GC overhead limit exceeded
error in Java.
the Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
Error
Unlike other programming languages, Java runtime uses a garbage collector GC process for Garbage collection. In Java, the garbage collector will clear the memory for them whenever a particular part of memory is not used.
The error Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
occurs when our code or application is exhausted all the memory, and GC cannot clean the memory anymore.
The error Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
signals that the JVM has taken too long to perform the garbage collection. JVM will throw this error when it takes 98% of the time to do the garbage collection, and only the other 2% of the heap can recover.
Here is an example that throws the same error:
package delftstack;
import java.util.*;
public class Example {
public static void main(String args[]) throws Exception {
Map DemoMap = System.getProperties();
Random DemoRandom = new Random();
while (true) {
DemoMap.put(DemoRandom.nextInt(), "value");
}
}
}
The code above will continuously put the random value in the map until garbage collection reaches 98%, and it will throw the Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
. The heap memory is used in the maps.
See output:
Exception: java.lang.OutOfMemoryError thrown from the UncaughtExceptionHandler in thread "main"
Even if we run this code using a parallel garbage collector, it will still throw the same error. See the example through CMD:
java -Xmx100m -XX:+UseParallelGC Example.java
It will still throw the same error. See output:
C:\Users\Sheeraz>java -Xmx100m -XX:+UseParallelGC Example1.java
Note: Example1.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
Exception in thread "main" java.lang.OutOfMemoryError: GC overhead limit exceeded
at java.base/java.util.concurrent.ConcurrentHashMap.putVal(ConcurrentHashMap.java:1047)
at java.base/java.util.concurrent.ConcurrentHashMap.put(ConcurrentHashMap.java:1006)
at java.base/java.util.Properties.put(Properties.java:1301)
at delftstack.Example.main(Example1.java:9)
Solve the Java.Lang.OutOfMemoryError: GC Overhead Limit Exceeded
Error
The solution to this error is to prevent memory leaks. Make sure the points below are followed so you can prevent memory leaks:
- Identify the places where memory allocation for the heap is done.
- It is the best practice to avoid creating a large amount of weakly referenced or temporary objects because mostly, they are the reason for memory leakage.
- Identify the object which occupies a large space on the heap.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack