How to Fix Android Java.Lang.IllegalStateException: Could Not Execute Method of the Activity
-
Reproduce the
java.lang.IllegalStateException: Could not execute method of the activity
Error -
Cause of the
java.lang.IllegalStateException: Could not execute method of the activity
Error -
Fix the
java.lang.IllegalStateException: Could not execute method of the activity
Error
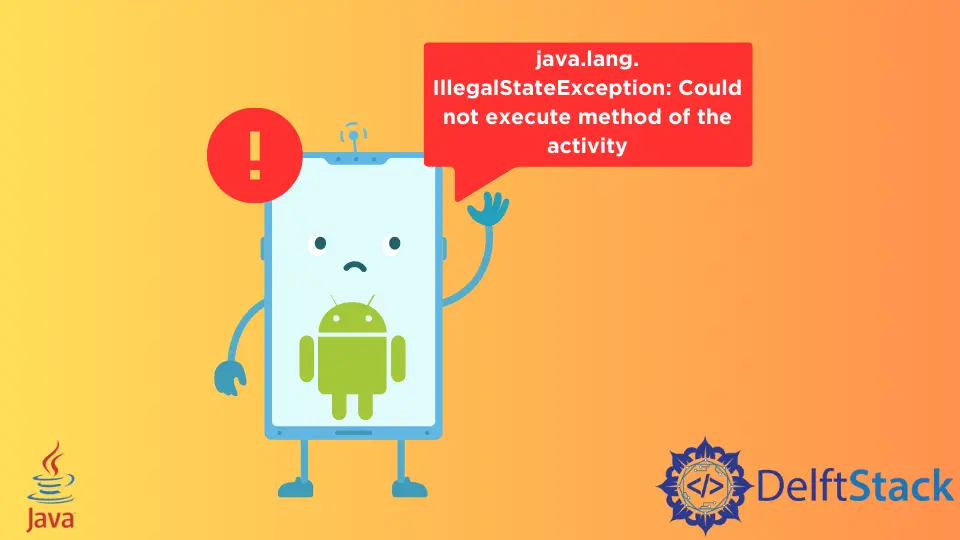
This tutorial takes us through the demonstration of the java.lang.IllegalStateException: Could not execute method of the activity
error while making an android application in Java. This also discusses the reason behind this error and provides the solution to resolve it.
Reproduce the java.lang.IllegalStateException: Could not execute method of the activity
Error
Example Code:
package com.example.npe;
import android.app.Activity;
import android.os.Bundle;
import android.text.Editable;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.*;
public class MainActivity extends Activity implements OnClickListener {
EditText ipAddressField;
TextView scanOut;
TextView portResults;
EditText startPort;
EditText stopPort;
protected void onCreate(Bundle instanceState) {
super.onCreate(instanceState);
setContentView(R.layout.activity_main);
ipAddressField = (EditText) findViewById(R.id.ip_address_field);
ipAddressField.getText();
scanOut = (TextView) findViewById(R.id.scan_out);
Button scanButton = (Button) findViewById(R.id.scanButton);
scanButton.setOnClickListener(this);
}
public void onClick(View view) {
Editable host = ipAddressField.getText();
switch (view.getId()) {
case R.id.scanButton:
try {
String pingCommand = "ping -c 5 " + host;
String pingOutput = "";
Runtime runtime = Runtime.getRuntime();
Process process = runtime.exec(pingCommand);
BufferedReader bufferedReader =
new BufferedReader(new InputStreamReader(process.getInputStream()));
String str;
while ((str = bufferedReader.readLine()) != null) {
System.out.println(str);
scanOut.setText(str + "\n"
+ "\n");
pingOutput += str;
scanOut.setText("/n" + pingOutput);
}
bufferedReader.close();
} // try
catch (IOException exception) {
System.out.println(exception);
}
break;
default:
break;
}
}
public void OnClickPort(View view) {
switch (view.getId()) {
case R.id.button1:
int rangeOfStartPort = Integer.parseInt(startPort.getText().toString());
int rangeOfStopPort = Integer.parseInt(stopPort.getText().toString());
for (int j = rangeOfStartPort; j <= rangeOfStopPort; j++) {
try {
Socket serverSocket = new Socket("192.168.0.1", j);
Toast.makeText(getApplicationContext(), "Port that is in use: " + j, Toast.LENGTH_LONG)
.show();
serverSocket.close();
} catch (Exception exception) {
exception.printStackTrace();
}
System.out.println("Port that is not in use: " + j);
}
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater menuInflater = getMenuInflater();
menuInflater.inflate(R.menu.activity_main, menu);
return super.onCreateOptionsMenu(menu);
}
}
We have written this simple code to make the semi-useful application.
We are basically at a minute taking useful bits of the Java code (ping A, Port scan). Then, we add them to our application, but when we run it, it generates the following error at runtime.
Error Description:
FATAL EXCEPTION: main
java.lang.IllegalStateException: Could not execute method of the activity
Why are we having this annoying error? Check the following section to find out the possible reason.
Cause of the java.lang.IllegalStateException: Could not execute method of the activity
Error
This error is caused by NullPointerException
, which generates the IllegalStateException
in an exception chain. So, it is essential to know enough about null
, NullPointerException
, and IllegalStateException
to figure out why this error occurs.
The null
is a very well-known keyword among computer programmers. It is a literal for reference variables or data types, for instance, Arrays
, Enums
, Interfaces
, and Classes
.
The NullPointerException
is a runtime exception that occurs when the application tries to retrieve an object reference which means accessing methods with a null
value. Looking back at our program, we can see that any variable is not initialized with a null
value.
The important point is that the null
value automatically gets stored in a reference variable whenever we declare it but do not initialize it. The reference data types contain a null
value as their default value if they are not initialized during the declaration process.
On the other hand, the IllegalStateException
is a runtime exception that shows that the method has been called or invoked at the illegal or wrong time.
We now know that the error is caused by NullPointerException
, which generates the IllegalStateException
in an exception chain. So, let’s figure out how to fix this.
Fix the java.lang.IllegalStateException: Could not execute method of the activity
Error
At the start of the MainActivity.java
class, we have two variables named startPort
and stopPort
of type EditText
that we have not initialized but declared. It means their default value is null
at the time when we try to run the following lines of code.
Example Code:
int rangeOfStartPort = Integer.parseInt(startPort.getText().toString());
int rangeOfStopPort = Integer.parseInt(stopPort.getText().toString());
The reason for getting the java.lang.IllegalStateException: Could not execute method of the activity
error is that we are trying to access the reference variables of type EditText
, which contains null
. So, we need to initialize startPort
and stopPort
inside the onCreate()
method by using findViewById()
as follows.
Example Code:
startPort = (EditText) findViewById(R.id.write_start_field_here);
stopPort = (EditText) findViewById(R.id.write_stop_field_here);
The findViewById()
method will take the component’s id
value as a parameter and helps to locate a component that is present in the android application. Another solution is to add a try-catch
block as follows.
Example Code:
try {
int rangeOfStartPort = Integer.parseInt(startPort.getText().toString());
int rangeOfStopPort = Integer.parseInt(stopPort.getText().toString());
} catch (Exception ex) {
ex.printStackTrace();
}
Using the try-catch
block, we can catch
the NullPointerException
and run our application without making it crash. Here, we write only that piece of code inside the try
block that may generate an exception.
While the catch
block is responsible for handling that exception that occurs in the try
block and resumes execution from there. Remember, after executing the catch
block, the program does not return to the try
block.
That is why we only write those lines of code in the try
block, which can result in exceptions.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack