How to Fix ExceptionInInitializer Error in Java
-
Brief Introduction to
ExceptionInInitializerError
in Java -
Handle the
ExceptionInInitializerError
in Java - Conclusion
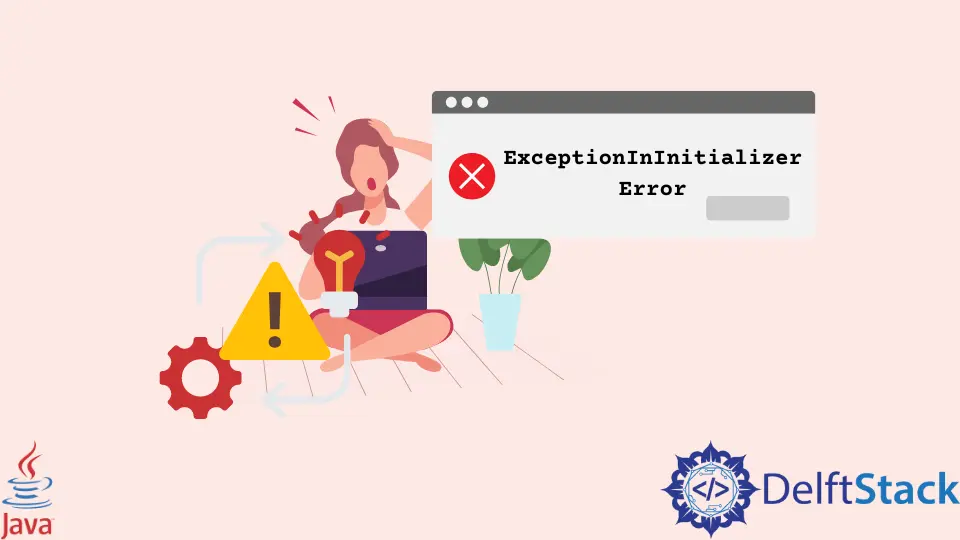
In this article, we will learn about the ExceptionInInitializerError
in Java.
Brief Introduction to ExceptionInInitializerError
in Java
ExceptionInInitializerError
is an unchecked exception in Java, and it’s the child of the Error
class. It falls in the category of Runtime Exceptions.
In Java, whenever the JVM (Java Virtual Machine) fails to evaluate a static initializer block or instantiate or assign a value to a static variable, an exception ExceptionInInitializerError
occurs. This indicates that something has gone wrong in the static initializer.
Whenever this exception occurs inside the static initializer, Java maintains the reference to the actual exception as the root cause by wrapping the exception inside the object of the ExceptionInInitializerError
class.
Examples of ExceptionInInitializerError
in Java
Based on the above discussion, ExceptionInInitializerError
occurs in major cases. Let’s see some examples to understand it better.
Example 1: Scenario where we are assigning values to the static variable.
public class Test {
static int x = 100 / 0;
public static void main(String[] args) {
System.out.println("Value of x is " + x);
}
}
Output:
Exception in thread "main" java.lang.ExceptionInInitializerError
Caused by: java.lang.ArithmeticException: / by zero
at Test.<clinit>(Test.java:4)
In the above code, we assigned a 100/0
value to a static variable x
, which gives an undefined arithmetic behavior, so an exception occurs while assigning values to the static variable, which finally gives us the ExceptionInInitializerError
.
We can also observe in the output that the actual exception ArithmeticException
is wrapped inside an instance of the ExceptionInInitializerError
class.
Example 2: Scenario where inside the static blocks, null values are assigned.
public class Test {
static {
String str = null;
System.out.println(str.length());
}
public static void main(String[] args) {}
}
Output:
Exception in thread "main" java.lang.ExceptionInInitializerError
Caused by: java.lang.NullPointerException: Cannot invoke "String.length()" because "str" is null
at Test.<clinit>(Test.java:7)
In the above code, we have created a static block inside which we have a string str
with the null
value. So, when we try to get its length using the length()
method, we get NullPointerException
as we print the length of a string with null
as its value.
But, as this exception occurs inside a static block, it will be wrapped inside the ExceptionInInitializerError
class, and we get ExceptionInInitializerError
in the output.
Handle the ExceptionInInitializerError
in Java
ExceptionInInitializerError
in Java can be avoided by ensuring the following points:
- Make sure that initializing static variables in a program doesn’t throw any Runtime Exception.
- Make sure that the static initializer blocks in a program don’t throw any Runtime Exception.
Conclusion
In this article, we learned about ExceptionInInitializerError
in Java, indicating that some exceptions occurred while initializing a static variable or evaluating a static block. This error works as a runtime wrapper for the underlying exception and stops the JVM until the programmer resolves the underlying exception.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack