How to Fix java.lang.reflect.InvocationTargetException Error in Java
-
the
java.lang.reflect.InvocationTargetException
Error in Java -
Causes of the
java.lang.reflect.InvocationTargetException
Error -
Fix the
java.lang.reflect.InvocationTargetException
Error in Java - Conclusion
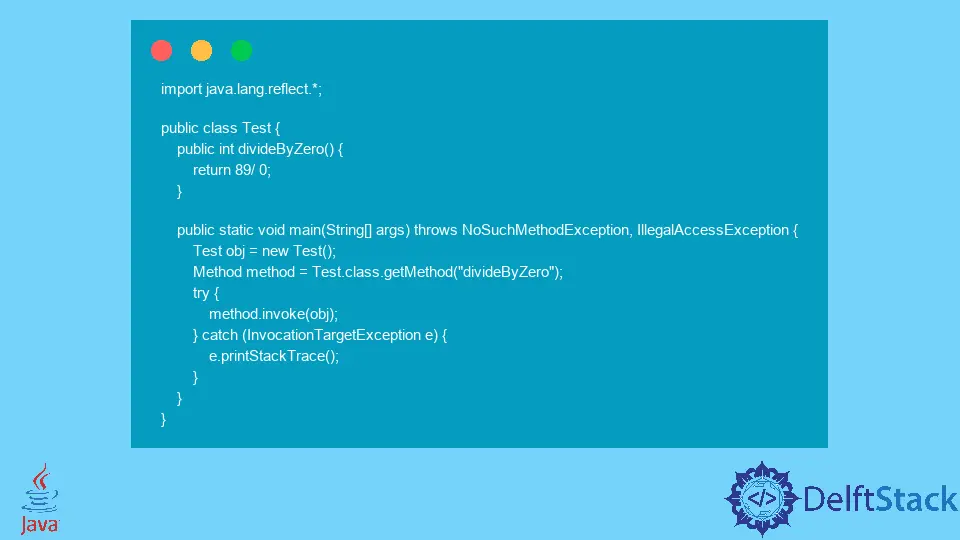
In this article, we will learn about java.lang.reflect.InvocationTargetException
in Java.
the java.lang.reflect.InvocationTargetException
Error in Java
The java.lang.reflect.InvocationTargetException
is a very common exception whenever a developer is working with Java Reflection
API. A checked
exception holds an exception thrown by the invoked method or a constructor.
As of release JDK 1.4,
this exception has been retrofitted to conform to the general-purpose exception chaining mechanism. In short whenever a developer tries to invoke a class using the Method.invoke()
we get InvocationTargetException
and it is wrapped around by java.lang.reflect.InvocationTargetException
.
Causes of the java.lang.reflect.InvocationTargetException
Error
The InvocationTargetException
mainly occurs when a developer is working with the reflection layer and trying to invoke a constructor or a method that throws an underlying exception itself. So the Java reflection API
wraps the exception thrown by the method with the InvocationTargetException
.
Let’s have a code example to understand it better.
Example code:
import java.lang.reflect.*;
public class Test {
public int divideByZero() {
return 89 / 0;
}
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException {
Test obj = new Test();
Method method = Test.class.getMethod("divideByZero");
try {
method.invoke(obj);
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
}
Output:
java.lang.reflect.InvocationTargetException
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:77)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:568)
at Test.main(Test.java:13)
Caused by: java.lang.ArithmeticException: / by zero
at Test.divideByZero(Test.java:6)
... 5 more
Fix the java.lang.reflect.InvocationTargetException
Error in Java
Based on the above, we understood that the underlying exception is the reason for the java.lang.reflect.InvocationTargetException
error. We can get more information about the underlying exception using the getCause ()
method of the Throwable
class.
Hence, solving the InvocationTargetException
involves finding and resolving the underlying exception.
Example code:
import java.lang.reflect.*;
public class Test {
public int divideByZero() {
return 89 / 0;
}
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException {
Test obj = new Test();
Method method = Test.class.getMethod("divideByZero");
try {
method.invoke(obj);
} catch (InvocationTargetException e) {
System.out.println(e.getCause());
}
}
}
Output:
java.lang.ArithmeticException: / by zero
In the above output, the actual underlying exception is ArithmeticException
, occurring because we are dividing by zero.
Once we fix the underlying exception, the InvocationTargetException
is also resolved. The following is the full working code with no exceptions; We just removed the divide by zero part.
Complete Source Code:
import java.lang.reflect.*;
public class Test {
public int divideByZero() {
return 89 / 9;
}
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException {
Test obj = new Test();
Method method = Test.class.getMethod("divideByZero");
try {
method.invoke(obj);
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
}
Conclusion
In this article, we learned how underlying exceptions are wrapped when we are working reflection layer in Java. We understood how to get the underlying exception when working with java.lang.reflect.InvocationTargetException
and how to resolve it.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack