Identifier Expected Error in Java
-
Understanding
<identifier> expected
Error in Java - Example 1: When Parameters in a Method Are Missing Data Type or Name
- Example 2: When the Expression Statements Are Misplaced
- Example 3: When Declaration Statements Are Misplaced
- Conclusion
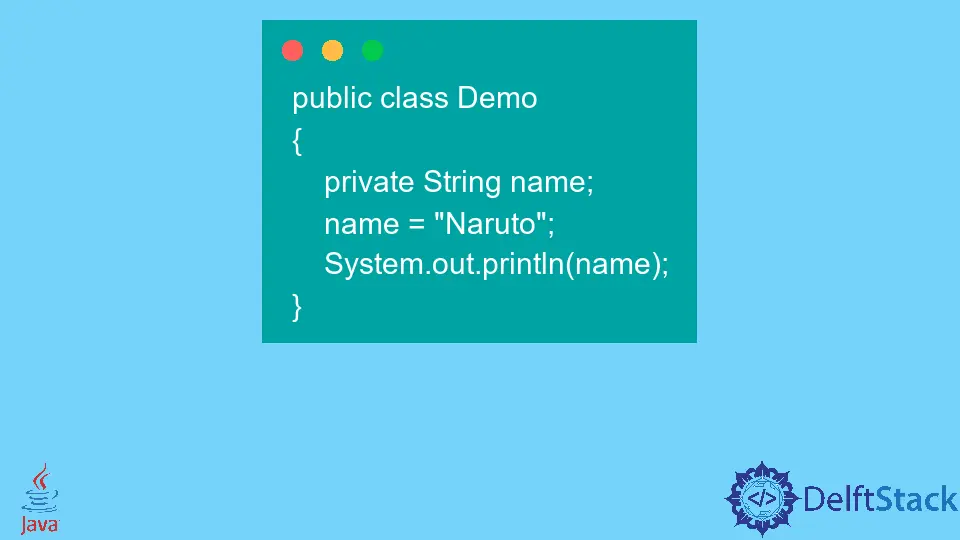
In this article, we will learn about Java’s <identifier> expected
error.
Understanding <identifier> expected
Error in Java
The <identifier> expected
is the most common Java compile-time error faced by novice programmers. This error occurs when a method parameter does not have a data type or name declared or when an expression statement is written outside a method, a constructor, or an initialization block.
In Java, an identifier is a sequence of one or more characters where the 1st character must be a letter or $
or _
, and the subsequent characters can be letters, digits, $
or _
.
These identifiers are used to name a variable, a method, a class, a package, an interface, etc.
When the Java code is compiled initial phase involves lexical analysis of the program. Here the program is categorized into tokens, and all the tokens, including identifiers, are verified against a set of grammar rules.
And whenever as per grammar rules, an identifier is expected, but it’s not present, and something else is found in its place compiler raises the <identifier> expected
error. Angle brackets here signify a reference to the token objects.
Let’s look at some examples to understand it better now.
Example 1: When Parameters in a Method Are Missing Data Type or Name
Parameters inside a method must have data followed by the variable name, which is an identifier; missing either of those will inevitably raise the <identifier> expected
error.
-
Data of the parameter is missing
public class Demo { public static int square(x) { return x * x; } public static void main(String[] args) { System.out.println(square(10)); } }
Output:
square.java:9: error: <identifier> expected public static int square(x) { ^ 1 error
If we observe the error above, we see that variable
x
is missing the data type. If we give the data type for it, it will work properly.public class Demo { public static int square(int x) { return x * x; } public static void main(String[] args) { System.out.println(square(10)); } }
Output:
100
-
The parameter’s data type is the identifier; the variable name is missing here.
public class Demo { public static int square(int) { return x * x; } public static void main(String[] args) { System.out.println(square(10)); } }
Output:
square.java:9: error: <identifier> expected public static int square(int) { ^ 1 error
If we observe the error above, we can clearly see that we specified the data type but didn’t attach any variable name.
public class Demo { public static int square(int x) { return x * x; } public static void main(String[] args) { System.out.println(square(10)); } }
Output:
100
Example 2: When the Expression Statements Are Misplaced
The compiler raises an error when an expression statement is written outside a method, a constructor or an initialization block <identifier> expected
.
Example code:
public class Demo {
private String name;
name = "Naruto";
System.out.println(name);
}
Output:
Demo.java:9: error: <identifier> expected
name = "Naruto";
^
Demo.java:10: error: <identifier> expected
System.out.println(name);
^
Demo.java:10: error: <identifier> expected
System.out.println(name);
^
3 errors
If we observe the error above, the error occurs because the statements are not enclosed within a function or a constructor. Let’s write these statements inside a method named print
.
public class Demo {
private String name;
public void print() {
name = "Naruto";
System.out.println(name);
}
public static void main(String[] args) {
Demo obj = new Demo();
obj.print();
}
}
Output:
Naruto
Example 3: When Declaration Statements Are Misplaced
This is rare but not unheard of when working with try-with-resources
statements. All these statements require that any closable resource be declared immediately after the try
keyword.
If the resource variable is declared outside the try-with-resources
statement, then the <identifier> expected
error might be raised by the compiler (as compiler to compiler, this may vary).
Example code: Here, the closable resource is BufferedReader
.
import java.io.*;
public class Demo {
public static void main(String[] args) {
StringBuilder obj = new StringBuilder();
BufferedReader br = null;
try (br = new BufferedReader(new InputStreamReader(System.in))) {
String lines = "";
while (!(lines = br.readLine()).isBlank()) {
obj.append(lines);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Demo.java:13: error: <identifier> expected
try (br = new BufferedReader(new InputStreamReader(System.in))) {
^
1 error
The error will be resolved if we declare the BufferedReader obj
inside the try
block.
import java.io.*;
public class Demo {
public static void main(String[] args) {
StringBuilder obj = new StringBuilder();
try (BufferedReader br = new BufferedReader(new InputStreamReader(System.in))) {
String lines = "";
while (!(lines = br.readLine()).isBlank()) {
obj.append(lines);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Conclusion
In this article, we learned about why the <identifier> expected
error had been raised by the compiler and how it’s the most common compile-time error faced by newbies. We also looked at different examples analyzing the cases when this error was raised.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack