How to Create Global Variable in Java
-
Create Global Variable Using the
static
Keyword in Java -
Create Global Variables by Using
interfaces
in Java -
Create Global Variable Using
static
andfinal
Keyword in Java
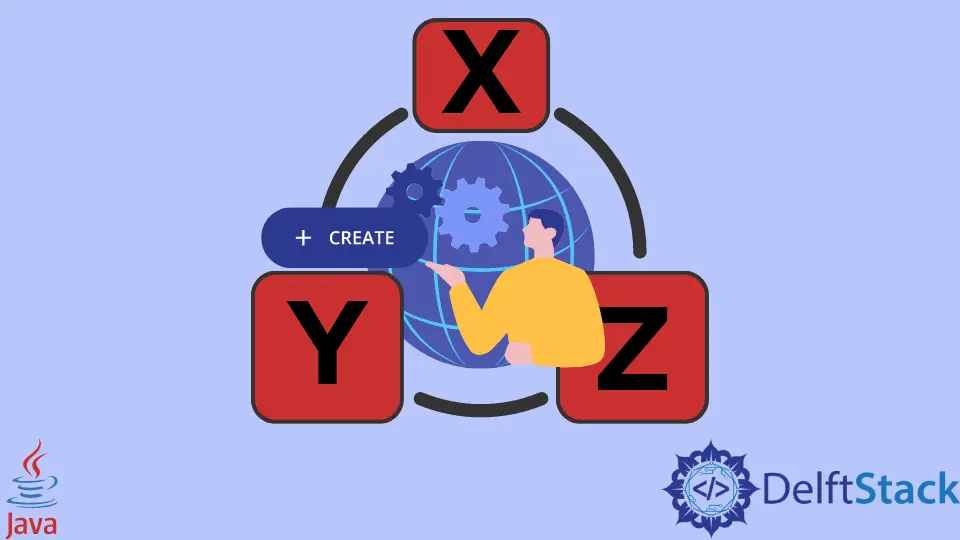
This tutorial introduces how to create a global variable in Java.
There is no concept of a global variable in Java. We cannot create global variables as we do in other programming languages such as C or C++. However, we can achieve this by using some existing concepts such as static and final static variables in class or the use of an interface where we can declare a variable as constant and use it as a global variable.
We use a static variable to create a global variable because the static variable is used to share common properties between objects and does not belong to any specific object. All the static variables belong to class only. Let’s see some examples.
Create Global Variable Using the static
Keyword in Java
This is the simplest way to create a global variable in Java. We make a class Employee
containing two static variables, id
and name
, and we call them inside the other class SimpleTesting
. Static variables can be accessed by using the class name. We don’t need to create an object to call static variables. See the example below.
class Employee {
static int id;
static String name;
}
public class SimpleTesting {
public static void main(String[] args) {
Employee.id = 125;
Employee.name = "Rohan";
int empId = Employee.id;
String name = Employee.name;
System.out.println("Id: " + empId);
System.out.println("Name: " + name);
}
}
Output:
Value in double: 12.9
Value in int: 12
Create Global Variables by Using interfaces
in Java
This is another option that can be used to create a global variable in Java. Here, we create an interface, GlobalVals
containing two variables, id
and name
. All the variables of an interface are public static and final by default so that they can be used as global variables.
Variables of an interface neither require object nor interface name to call. We need to implement the interface in a class and call the variable as a local variable. See the example below.
interface GlobalVals {
int id = 1212;
String name = "Samre";
}
public class SimpleTesting implements GlobalVals {
public static void main(String[] args) {
int empId = id;
String empName = name;
System.out.println("Id: " + empId);
System.out.println("Name: " + empName);
}
}
Output:
Id: 1212
Name: Samre
Create Global Variable Using static
and final
Keyword in Java
The concept of static and final variables is used to create a constant in Java. We can use this to create a global variable too. We create a class, GlobalVals
that contains two static final variables, and in the SimpleTesting
class, we call them by simple class name. See the example below.
class GlobalVals {
static final int ID = 1212;
static final String NAME = "Samre";
}
public class SimpleTesting {
public static void main(String[] args) {
int empId = GlobalVals.ID;
String empName = GlobalVals.NAME;
System.out.println("Id: " + empId);
System.out.println("Name: " + empName);
}
}
Output:
Id: 1212
Name: Samre
Related Article - Java Variable
- Java Synchronised Variable
- Difference Between Static and Final Variables in Java
- How to Set JAVA_HOME Variable in Java
- How to Create Counters in Java
- How to Increase an Array Size in Java
- How to Access a Variable From Another Class in Java