How to Fix the Java Error: Generic Array Creation
-
Understanding the
generic array creation
Error in Java -
Possible Causes for the
generic array creation
Error in Java -
Possible Solutions to Eradicate the
generic array creation
Error in Java -
Solution 1: Use the
Object
Array -
Solution 2: Use the
reflect
Feature -
Solution 3: Use an
ArrayList
orList
-
Solution 4: Use
Varargs
and@SafeVarargs
(Java 7 and Later) - Conclusion
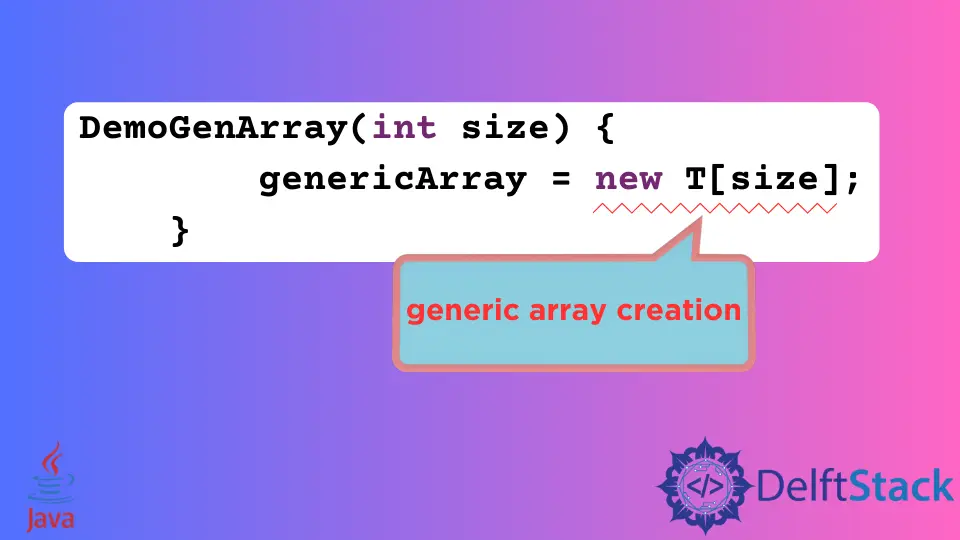
Java, as a statically typed language, often enforces strict type-checking to enhance code reliability and safety. One common issue encountered by developers is the "generic array creation"
error, which arises when attempting to create an array of a generic type directly.
This tutorial illustrates the generic array creation
error via code sample and highlights why we have this error while creating a generic array. This discussion will lead to the solution where we will learn how to create a generic array using the Object
array and reflect
feature.
We will explore various methods to address this error, providing detailed explanations and examples for each approach.
Understanding the generic array creation
Error in Java
The generic array creation error occurs because Java’s generics are implemented using type erasure, meaning that generic type information is not available at runtime. Consequently, creating an array of a generic type is problematic as the compiler cannot ensure type safety.
Below is a code demonstrating the Java error "generic array creation"
(DemoGenArray.java
Class):
import java.util.Arrays;
public class DemoGenArray<T> {
private T[] genericArray;
DemoGenArray(int size) {
genericArray = new T[size]; //<----This Line Has Generic Array Creation Error
}
public T get(int index) {
return (T) genericArray[index];
}
public void set(int index, T item) {
genericArray[index] = item;
}
@Override
public String toString() {
return Arrays.toString(genericArray);
}
public static void main(String[] args) {}
}
In this class, we try to create and initialize a generic array with the specified array size
. Further, we add elements to the array and return the items individually from the given index
. The toString()
returns the whole array at once.
The issue is that we have a generic array creation
error at the line we are pointing out in the constructor. Let’s explore the possible causes for this error, which will help us to move towards its solution.
Possible Causes for the generic array creation
Error in Java
We are trying to create a generic array in our code, which is impossible in Java. It is because Java consciously decides to explicitly stop these syntaxes from working.
Remember, arrays are covariant (we can assign a sub-type to its super-type reference) in Java, while generics are not.
private T[] genericArray;
genericArray = new T[size];
The above two lines of code are the same as given below. You can use any one of them.
private T[] genericArray = new T[size];
It happens due to the type-erasure
that Java implements. It is a process carried out by the Java compiler.
It removes parameterized types in generics and maps them to raw types in the byte code.
Remember, the byte code doesn’t have any details on generics. Using the type T
will be fine at compile-time but has no meaning at runtime.
In other ways, we can circumvent this restriction that are given below with code examples. Let’s learn each of them below.
Possible Solutions to Eradicate the generic array creation
Error in Java
As we already know, the compiler does not have any information regarding parameterized types at runtime. So, whenever we are required to use generics, it is good to prefer and use the list component of the Java Collections
framework instead of using arrays.
However, we can still create array-like generic structures, but this solution depends on whether it is checked
or unchecked
. We use the Object
array if it is unchecked
.
In the case of checked
, we can use Java’s reflect
feature. Let’s see how each of them works.
Solution 1: Use the Object
Array
One workaround is to use arrays of the raw type Object
and then cast elements as needed. While this approach sacrifices some type safety, it provides a practical solution to the generic array creation
error.
Example Code (DemoGenArray.java
class):
import java.util.Arrays;
public class DemoGenArray<T> {
private Object[] genericArray;
DemoGenArray(int size) {
genericArray = new Object[size];
}
public T get(int index) {
return (T) genericArray[index];
}
public void set(int index, T item) {
genericArray[index] = item;
}
@Override
public String toString() {
return Arrays.toString(genericArray);
}
}
Here, we use the Object
array to simulate the generic array because the DemoGenArray
class is unchecked
(weak typing). We can use this approach if we know that no type checking would be performed on objects passed as the arguments.
Further, we use generic get()
and set()
methods to return value and set value, respectively. The get()
method uses an explicit cast to T
where T
acts like a placeholder for the generics and represents any value/element.
This works fine if we use set()
and get()
methods and do not let the user directly access the Object
array.
Coming to the GenArray
class, which contains the main()
method. Here, we create the instance of the DemoGenArray
class.
We pass the required type while instantiating the DemoGenArray
class and populating them. After that, we use the toString()
method to write the contents of all instances.
Example Code (GenArray.java
class):
public class GenArray {
public static void main(String[] args) {
DemoGenArray<String> strArray = new DemoGenArray(3);
strArray.set(0, "one");
strArray.set(1, "two");
strArray.set(2, "three");
DemoGenArray<Integer> intArray = new DemoGenArray(3);
intArray.set(0, 10);
intArray.set(1, 20);
intArray.set(2, 30);
DemoGenArray<Double> doubleArray = new DemoGenArray(3);
doubleArray.set(0, 15.0);
doubleArray.set(1, 110.0);
doubleArray.set(2, 10.0);
System.out.println("Integer Array: " + intArray);
System.out.println("String Array: " + strArray);
System.out.println("Double Array: " + doubleArray);
}
}
Output:
Integer Array: [10, 20, 30]
String Array: [one, two, three]
Double Array: [15.0, 110.0, 10.0]
Solution 2: Use the reflect
Feature
Reflection provides a way to create arrays of generic types by capturing the component type at runtime. While this method involves more complexity, it offers a workaround for the generic array creation
error.
Example Code (DemoGenArray.java
class):
import java.lang.reflect.Array;
import java.util.Arrays;
public class DemoGenArray<T> {
private T[] genericArray;
DemoGenArray(Class<T> classType, int size) {
genericArray = (T[]) Array.newInstance(classType, size);
}
public T get(int index) {
return (T) genericArray[index];
}
public void set(int index, T item) {
genericArray[index] = item;
}
@Override
public String toString() {
return Arrays.toString(genericArray);
}
}
Here, we are using the reflection class to create the generic array whose type will only be known at runtime. This solution is similar to Solution 1 except for two differences in the DemoGenArray
class.
First, we have a constructor in the DemoGenArray
class that accepts two arguments: the type and size of the array. Second, we initialize the genericArray
using the Array.newInstance()
method.
The Array.newInstance()
method creates a new array using the given dimension (size of the array) and component type.
Example Code (GenArray.java
class):
public class GenArray {
public static void main(String[] args) {
DemoGenArray<String> strArray = new DemoGenArray(String.class, 3);
strArray.set(0, "one");
strArray.set(1, "two");
strArray.set(2, "three");
DemoGenArray<Integer> intArray = new DemoGenArray(Integer.class, 3);
intArray.set(0, 10);
intArray.set(1, 20);
intArray.set(2, 30);
DemoGenArray<Double> doubleArray = new DemoGenArray(Double.class, 3);
doubleArray.set(0, 15.0);
doubleArray.set(1, 110.0);
doubleArray.set(2, 10.0);
System.out.println("Integer Array: " + intArray);
System.out.println("String Array: " + strArray);
System.out.println("Double Array: " + doubleArray);
}
}
Output:
Integer Array: [10, 20, 30]
String Array: [one, two, three]
Double Array: [15.0, 110.0, 10.0]
Solution 3: Use an ArrayList
or List
An effective workaround to the generic array creation
error is to use ArrayList
or List
from the Java Collections Framework. These dynamic data structures allow the storage of elements without encountering the limitations associated with generic arrays.
Instead of creating an array of a generic type directly, utilize a parameterized collection such as ArrayList
or List
. This allows for dynamic resizing and avoids the generic array creation
error.
Example 1: Utilizing ArrayList
for Strings
Consider the following example where we use an ArrayList
to store strings dynamically:
import java.util.ArrayList;
import java.util.List;
public class GenericArrayExample1 {
public static void main(String[] args) {
// Using ArrayList to store strings
List<String> stringList = new ArrayList<>();
// Adding elements dynamically
stringList.add("Item 1");
stringList.add("Item 2");
// Converting ArrayList to an array
// No generic array creation error
String[] stringArray = stringList.toArray(new String[0]);
}
}
In this example, we employ an ArrayList
to store strings, dynamically adding elements and later converting them to an array using the toArray
method. This approach is flexible and aligns with Java’s Collections framework.
Example 2: Using List
for Generic Elements
Here, we demonstrate the use of a generic List
to handle a collection of various types:
import java.util.ArrayList;
import java.util.List;
public class GenericArrayExample2 {
public static void main(String[] args) {
// Using List to store generic elements
List<Object> genericList = new ArrayList<>();
// Adding elements dynamically
genericList.add("Item 1");
genericList.add(42);
genericList.add(3.14);
genericList.add(true);
// Converting List to an array
Object[] genericArray = genericList.toArray();
}
}
In this case, we use a generic List
to store elements of different types, showcasing the flexibility and versatility of this approach.
Solution 4: Use Varargs
and @SafeVarargs
(Java 7 and Later)
Varargs
, short for variable-length argument lists, is a feature introduced in Java 5 that allows methods to accept a variable number of arguments of the same type. This flexible syntax can be leveraged to overcome the generic array creation
error.
Java 7 introduced the @SafeVarargs
annotation, allowing safe use of varargs
with generics. While not a direct solution, it provides a way to suppress unchecked warnings.
Example 1: Basic Varargs
Usage
Consider the following example where a generic method uses varargs
to create an array.
public class VarargsExample1 {
public static void main(String[] args) {
// No generic array creation error with varargs
String[] stringArray = createArray("Item 1", "Item 2", "Item 3");
}
// Generic method using varargs
private static <T> T[] createArray(T... elements) {
return elements;
}
}
In this example, the createArray
method utilizes varargs
to accept an arbitrary number of elements and returns an array of the inferred type.
the Role of @SafeVarargs
While varargs
offer a flexible solution, they come with a warning about potential unchecked operations. To address this concern, Java 7 introduced the @SafeVarargs
annotation.
This annotation assures the compiler that the varargs
method does not perform any unsafe operations.
Example 2: Using @SafeVarargs
In the following example, the createSafeArray
method is annotated with @SafeVarargs
to suppress unchecked warnings.
public class VarargsExample2 {
public static void main(String[] args) {
// No unchecked warnings with @SafeVarargs
String[] stringArray = createSafeArray("Item 1", "Item 2", "Item 3");
}
// Generic method with @SafeVarargs
@SafeVarargs
private static <T> T[] createSafeArray(T... elements) {
return elements;
}
}
The @SafeVarargs
annotation signals to the compiler that the method is safe to use with variable arguments, addressing the unchecked warning and reducing the chances of runtime issues.
Conclusion
This comprehensive article addresses the "generic array creation"
error in Java, a common challenge when attempting to create arrays of generic types directly. It starts by illustrating the error through a code sample, explaining the reasons behind its occurrence, and examining the impact of type erasure.
The article explores various solutions, providing in-depth explanations and examples for each approach:
- Using
Object
Arrays and Reflection:- Demonstrates how to create arrays of raw type
Object
and use explicit casting, sacrificing some type safety. - Introduces reflection as a more complex but powerful method, capturing the component type at runtime to create arrays of generic types.
- Demonstrates how to create arrays of raw type
- Using
ArrayList
orList
:- Recommends employing dynamic data structures from the Java Collections framework as an effective workaround.
- Shows examples of using
ArrayList
to store elements dynamically, avoiding the limitations associated with generic arrays.
- Using
Varargs
and@SafeVarargs
(Java 7 and later):- Explores the use of variable-length argument lists (
Varargs
) to create arrays without the generic array creation error. - Introduces the
@SafeVarargs
annotation to suppress unchecked warnings, enhancing safety and code readability.
- Explores the use of variable-length argument lists (
The article concludes by offering developers a versatile toolbox of techniques, allowing them to choose the most suitable solution based on their specific requirements and design principles. Whether opting for simplicity, sophistication, flexibility, or modern elegance, this article equips developers with the knowledge to navigate and mitigate the challenges posed by the generic array creation error in Java.
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack