How to Fix JavaFx Exception in Application Start Method
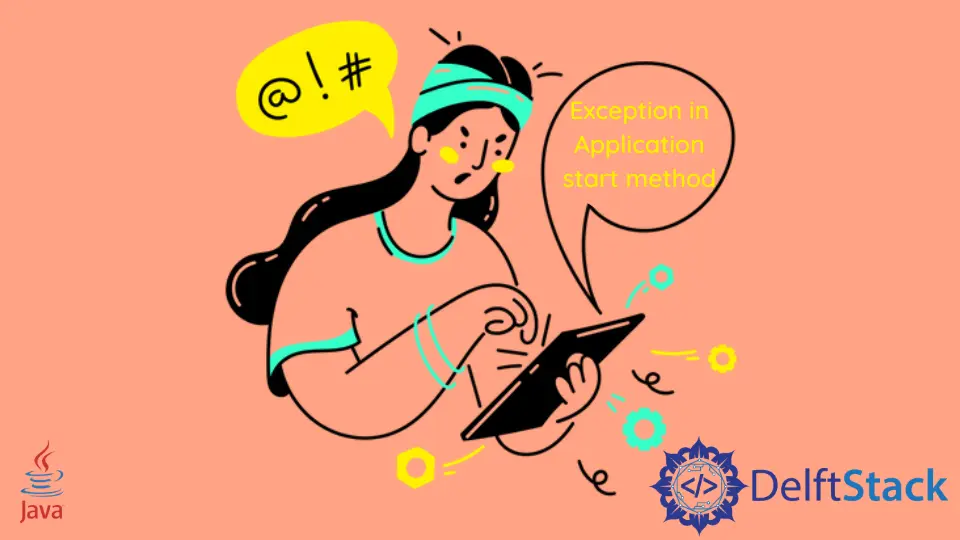
JavaFX is a highly enriched library whose code gets written in native Java code. The library gets used to making Rich Internet Applications, often known as RIA.
The library is a set of interfaces and classes that are easily understandable and are a friendly alternative to Java Virtual Machine or JVM. The code written using the library can run across multiple platforms without fail like desktops, mobiles, televisions, etc.
Long back, the graphical User Interface gets built using Swing templates, but after the advent of JavaFX, one can easily rely on the language to work over the same. The applications built using JavaFx have a penetration rate of 76 percent.
The Exception in Application start method
is the runtime error that occurs when the application is running and when compilation gets done. The state occurs when the application is inefficient in loading runtime variables or files. It can throw NullPointerException, FileNotFound type of exceptions when not handled properly.
Additionally, plugins like SonarLint, programming mistake detector(PMD), find bugs can help identify the runtime issues beforehand without actual program runs.
Below is an example to show the Exception in Application start method
error in JavaFx.
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class ApplicationStart extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage stage) throws Exception {
Parent parent = FXMLLoader.load(getClass().getResource("AnyXML.fxml"));
Scene scene = new Scene(parent);
stage.setScene(scene);
stage.setTitle("First Swing Sample");
stage.show();
}
}
The above source code that seems to be in JavaFx has a main
method in the ApplicationStart
class. The given class extends an abstract Application
class and is specifically available in JavaFX Library.
It has a default theme called Caspein
that gets launched once you start the application. The launch
is a static method present in the Application
class and gets called from the main
function. It takes variable arguments or varargs
as its parameters. It throws IllegalStateException
if the launch method gets called more than once.
The Application
class has one abstract method whose implementation must be present in ApplicationStart class. The override
annotation shows that the code below the annotation belongs to the parent Application
class. The implementation of the method that gets proceeded by the annotation override is present below the annotation.
The start
method is the main entry for the JavaFX applications, as main
is the entry location for the Java applications. The main
method gets first called when the Application
or main thread gets initialized.
The function takes Stage
as the parameter. The Stages denotes the primary step or view and gets loaded when the application launches in the applet viewer. It also throws Exception that gets defined along with the method.
The first statement inside the method is to load the XML file. The FXMLLoader
class loads an object hierarchy from the XML object model. It gets used to bring the object hierarchy from an FXML document into a Parent
instance. It takes the parameter as the URL to the location where the XML document hierarchy is present.
The resultant gets stored in a Parent
class instance that holds the subtypes in the graph format. The Scene
class present in the JavaFX library is the container unit that stores all the data in a graph view. The background of the scene gets filled by the specified property. The instance of Stage class gets created and can get used with other properties.
Below mentioned are the properties used to display the scene over the browser.
- The
setScene
method makes its use to specify the scene and gets used along with the stage instance variable. - The
setTitle
function gets used to set scene title present over the browser. Theshow
function gets used to populate the scene over the stage.
Below is the output for the above code block.
Exception in Application start method java.lang.reflect.InvocationTargetException at sun.reflect
.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl
.invoke(NativeMethodAccessorImpl.java : 62) at sun.reflect.DelegatingMethodAccessorImpl
.invoke(DelegatingMethodAccessorImpl.java : 43) at java.lang.reflect.Method
.invoke(Method.java : 498) at com.sun.javafx.application.LauncherImpl
.launchApplicationWithArgs(LauncherImpl.java : 389) at com.sun.javafx.application.LauncherImpl
.launchApplication(LauncherImpl.java : 328) at sun.reflect.NativeMethodAccessorImpl
.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl
.invoke(NativeMethodAccessorImpl.java : 62) at sun.reflect.DelegatingMethodAccessorImpl
.invoke(DelegatingMethodAccessorImpl.java : 43) at java.lang.reflect.Method
.invoke(Method.java : 498) at sun.launcher.LauncherHelper$FXHelper
.main(LauncherHelper.java : 873) Caused by
: java.lang.RuntimeException
: Exception in Application start method at com.sun.javafx.application.LauncherImpl
.launchApplication1(LauncherImpl.java : 917) at com.sun.javafx.application.LauncherImpl
.lambda$launchApplication$1(
LauncherImpl.java : 182) at java.lang.Thread.run(Thread.java : 748) Caused by
: java.lang.NullPointerException
: Location is required.at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java : 3207) at javafx.fxml
.FXMLLoader.loadImpl(FXMLLoader.java : 3175) at javafx.fxml.FXMLLoader
.loadImpl(
FXMLLoader.java : 3148) at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java : 3124) at javafx
.fxml.FXMLLoader.loadImpl(FXMLLoader.java : 3104) at javafx.fxml.FXMLLoader
.load(FXMLLoader.java : 3097) at ApplicationStart.start(ApplicationStart.java : 15) at com.sun
.javafx.application.LauncherImpl.lambda$launchApplication1$8(LauncherImpl.java : 863) at com.sun
.javafx.application.PlatformImpl.lambda$runAndWait$7(PlatformImpl.java : 326) at com.sun.javafx
.application.PlatformImpl.lambda$null$5(PlatformImpl.java : 295) at java.security
.AccessController.doPrivileged(Native Method) at com.sun.javafx.application.PlatformImpl
.lambda$runLater$6(PlatformImpl.java : 294) at com.sun.glass.ui.InvokeLaterDispatcher$Future
.run(InvokeLaterDispatcher.java : 95) at com.sun.glass.ui.win.WinApplication
._runLoop(Native Method) at com.sun.glass.ui.win.WinApplication.lambda$null$3(
WinApplication.java : 177)... 1 more Exception running application ApplicationStart
In the output shown above, the issue gets raised at the parameter position that uses the FXMLLoader
class to load the XML object, but it returns a null value. In simple words, the getResource()
method does not locate the path provided in the function parameter.
Hence, the null value populates NullPointerException, which is a type of runtime exception. And are handled by giving an absolute path where the file can get located. The stack trace often shows the line number where the issue starts populating. The target must be correct when get given in the load parameter.
Hence the given solution to the problem is below.
- Give the absolute path to the location where the file gets present.
- Add a SonarLint plugin to the Integrated Development Environment that helps in evaluating or handling the exceptions at the write time.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack