How to Fix Java Error: The Constructor Is Undefined
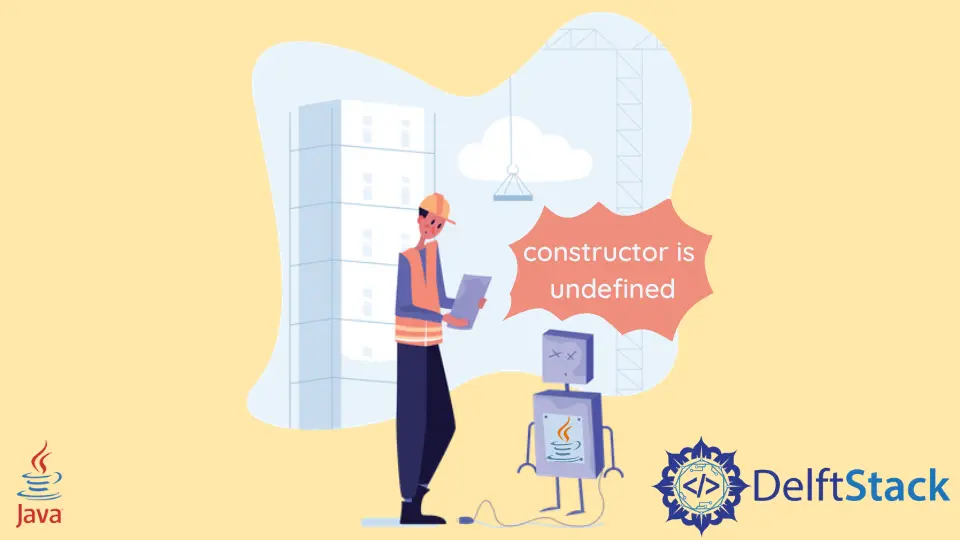
This guide will look into Java’s error, constructor not defined
.
the constructor is undefined
Error in Java
The constructor is undefined
is a very common error. This error occurs when we have not defined a constructor, as in the title.
It is very easy to come by this error as users may get confused between a default constructor and a parameterized constructor. The most common issue is that users define a constructor with parameters, but during the call, they don’t mention the parameters; Hence, this error occurs.
Another reason might be the way the constructor is defined. Constructors are to be defined without any return type, so if a return type is mentioned, it might give the same error.
Code Example:
public class Shapes {
double Shapes;
double Width;
double Height;
public void Shapes(double Name) // constructor defined
{
Name = Shape;
}
public void setHeight(double HeightOfShape) {
Height = HeightOfShape;
}
public void setWidth(double WidthOfShape) {
Width = WidthOfShape;
}
}
public class TestClass {
public static void main(String[] args) {
Shapes Shape1 = new Shapes(); // Error will occor here.
Shape1.setHeight(5);
Shape1.setWidth(3);
}
}
Output:
TestClass.java:24: error: constructor Shapes in class Shapes cannot be applied to given types;
Shapes Shape1 = new Shapes(); //Error will occor here.
^
required: double
found: no arguments
reason: actual and formal argument lists differ in length
This error constructor Shapes in class Shapes cannot be applied to given types;
is the same as the constructor is undefined
. A simple shapes function is defined here as a parameterized constructor, but the function won’t be called with parameters during the call.
The second reason is that a constructor does not accept a return type, So even mentioning a void
is against the rule.
Fix the constructor is undefined
Error in Java
The solution is as mentioned above while removing a return type, void
. By mentioning the parameters in the calling function, we can successfully remove the error, constructor is undefined
.
The code snippet below demonstrates how to fix the error stated in the previous code section. By removing these errors, we can run the program as we wish.
Code Example:
class Shapes {
double shape;
double Width;
double Height;
public Shapes(double Name) // removing return type
{
Name = shape;
}
public void setHeight(double HeightOfShape) {
Height = HeightOfShape;
}
public void setWidth(double WidthOfShape) {
Width = WidthOfShape;
}
}
public class TestClass {
public static void main(String[] args) {
Shapes Shape1 = new Shapes(3); // adding proper parameters
Shape1.setHeight(5);
Shape1.setWidth(3);
}
}
There won’t be any errors left, and the code will be successfully executed as desired. Note that the simplest of mistakes can cause these types of unnecessary errors.
Always keep in mind to properly revise the code and learn about the syntaxes of Java so that these types of errors won’t occur in the future.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack